React proptype array with shape
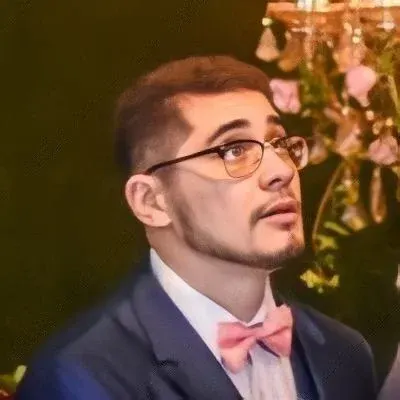
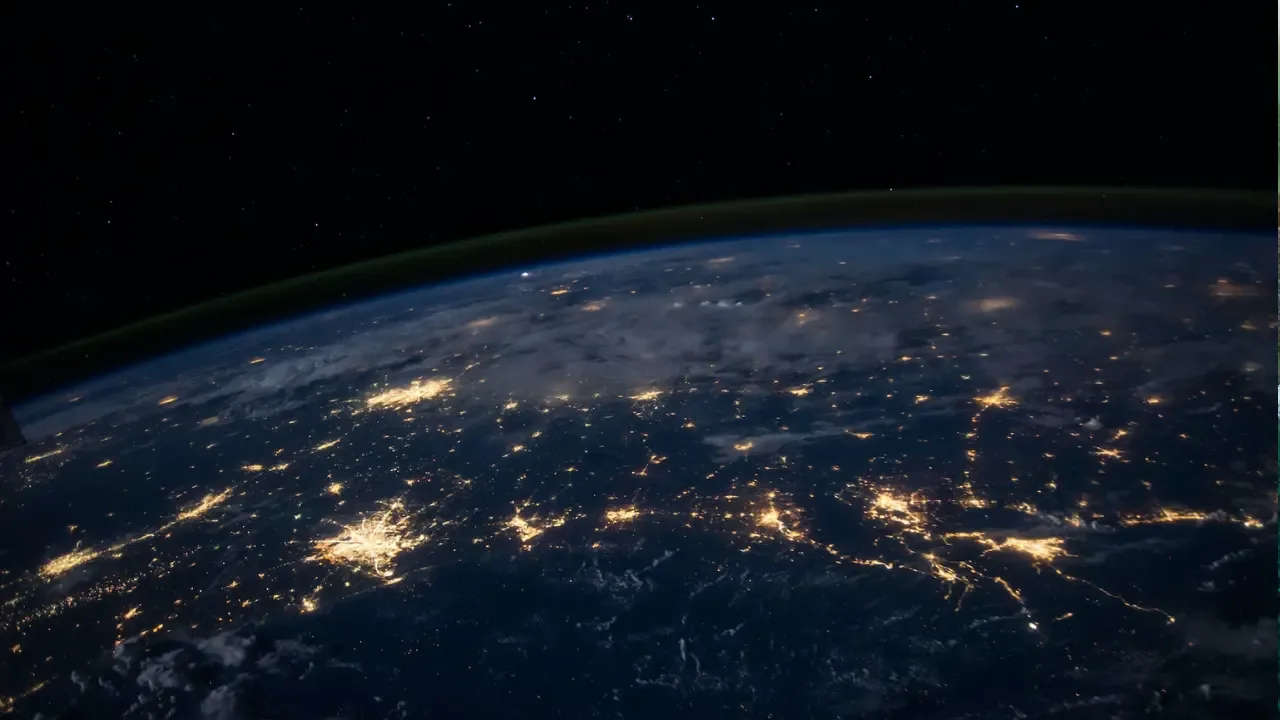
šTitle: Ensuring a Specific Shape for React PropTypes Arrays
š Hey there, tech enthusiasts! š Are you wondering if there's an easy and built-in way to use React PropTypes to ensure that an array of objects passed to a component matches a specific shape? š¤ Well, you've come to the right place! In this blog post, we'll address this common issue, provide you with simple solutions, and get you on your way to writing more robust React components. š
š§ The Problem: Ensuring Shape for an Array of Objects
So, the question at hand: is there a way to ensure that an array of objects being passed to a component is indeed an array of objects that follow a specific shape? š„š Let's take a look at the code example provided as context:
annotationRanges: PropTypes.arrayOf(PropTypes.shape({
start: PropTypes.number.isRequired,
end: PropTypes.number.isRequired,
})),
š¤ The author is asking if there's a way to validate that the annotationRanges
prop is an array of objects, where each object has two properties: start
(a required number) and end
(also a required number). Seems like a reasonable requirement, right? š¤·āāļø
š ļø The Solution: Defining Shape for the PropTypes Array
Surprise! š There's indeed a built-in and super convenient way to handle this kind of situation using React PropTypes. š The code example mentioned in the question is almost spot-on, with just a slight adjustment needed. š
To validate an array of objects with a specific shape using PropTypes, you can make use of PropTypes.arrayOf()
and PropTypes.shape()
like this:
annotationRanges: PropTypes.arrayOf(PropTypes.shape({
start: PropTypes.number.isRequired,
end: PropTypes.number.isRequired,
})).isRequired,
Notice the addition of isRequired
at the end. This addition ensures that the annotationRanges
prop is not only an array of objects with the desired shape but also a required prop. This is especially useful when you want to clearly communicate the expected data structure for your component. š
And there you have it! Your array of objects will now be validated against the specified shape. šÆ
Now you might be wondering, "Hey, what if I'm dealing with an array that doesn't have a strict length or is empty? Can I still utilize PropTypes to validate it?" š¤·āāļø Absolutely! You can handle those cases as well. Let's explore a few examples below:
An array with an undefined number of objects:
annotationRanges: PropTypes.arrayOf(PropTypes.shape({
start: PropTypes.number.isRequired,
end: PropTypes.number.isRequired,
})), // No isRequired here ā ļø
An empty array:
annotationRanges: PropTypes.arrayOf(PropTypes.shape({
start: PropTypes.number.isRequired,
end: PropTypes.number.isRequired,
})).isRequired, // Now isRequired ā ļø
š„³ Take It to the Next Level: Extra Tips and Tricks
Here are a few additional tips to help you level up your React PropTypes game! š
š Deep Validations: You can nest multiple
PropTypes.shape()
inside one another to define a more complex shape for your objects. Get creative!š¤ Using PropTypes.shape() with Other PropTypes: If you need to validate not just specific properties but their types as well, you can combine
PropTypes.shape()
with other PropTypes, likePropTypes.string
,PropTypes.arrayOf()
, orPropTypes.objectOf()
.
š£ Call-to-Action: Your Turn to Engage!
Hope you found this guide helpful and that it'll save you time and frustration when working with React PropTypes arrays with specific shapes! šŖ
Now it's your turn to share your thoughts! Have you encountered any issues related to validating array shapes with PropTypes? How did you tackle them? Feel free to leave a comment with your experiences or any questions you may have. Let's keep the conversation going and help each other out! š¤
So go ahead, leave a comment below, and share this blog post with your fellow developers who might need a hand with React PropTypes arrays! Together, we can build better React components! š
That's all for now, folks! Keep exploring, keep coding! Happy Reacting! āļøš
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
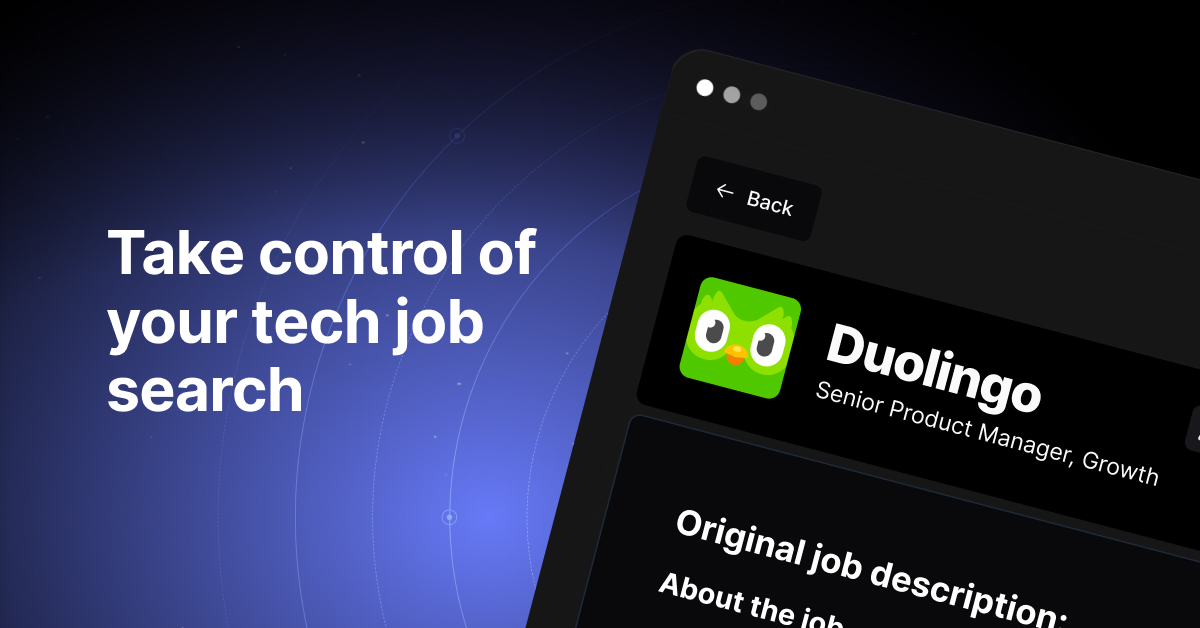