Populating VBA dynamic arrays
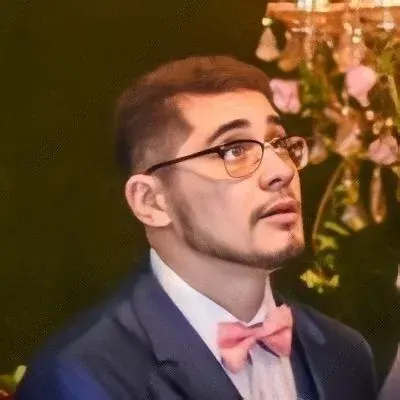
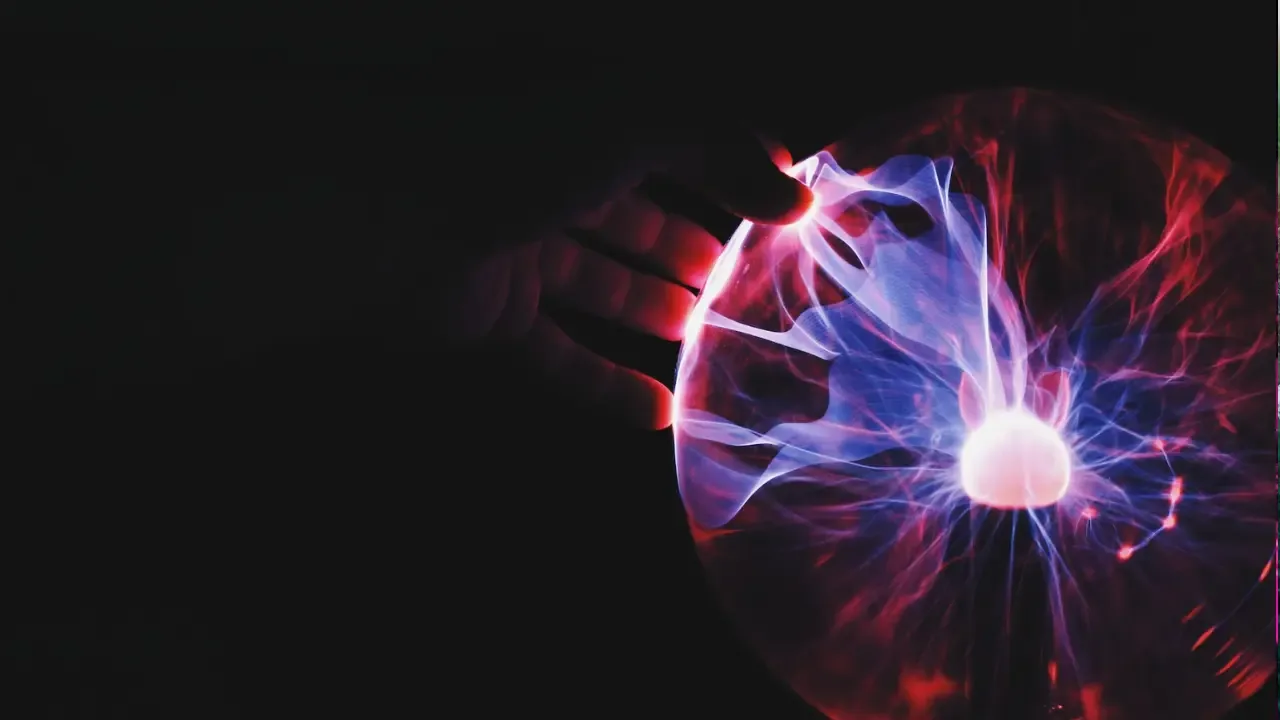
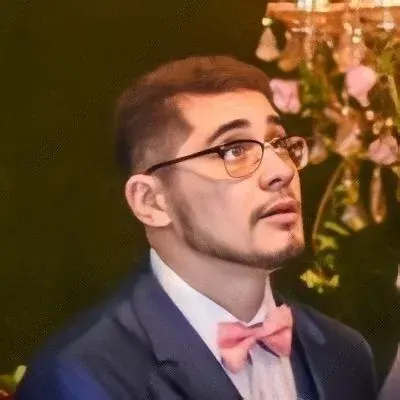
📝 Tech Blog: Populating VBA Dynamic Arrays Made Easy! 😎
Are you experiencing an error 🚫 that says "subscript out of range" when trying to populate a dynamic array in VBA? Fear not! This blog post will guide you through common issues and provide easy solutions to help you populate your VBA dynamic arrays effortlessly. Let's dive in! 💻
Understanding the Problem
In the given code snippet, the error occurs because the dynamic array test()
has not been properly initialized before trying to assign values to its elements. 😕 Unlike JavaScript, VBA requires you to explicitly resize the array before using it.
Solution: Resizing the Dynamic Array
To fix this issue, you need to resize the dynamic array before populating it with values. Let's take a look at the modified code snippet:
Sub test_array()
Dim test() As Integer
Dim i As Integer
ReDim test(0 To 3) ' Resizing the array before populating
For i = 0 To 3
test(i) = 3 + i
Next i
End Sub
By adding the ReDim
statement with the desired range of the array, you create the necessary "spot" for the values you want to store. Now, your dynamic array can be populated without any errors! ✅
Taking it a Step Further: Dynamic Resizing
What if you don't know the exact number of elements you'll be adding to the dynamic array? No worries! VBA allows you to dynamically resize the array based on the number of elements you want to add.
Here's an example that demonstrates dynamic resizing:
Sub test_array_dynamic()
Dim test() As Integer
Dim i As Integer
Dim currentSize As Integer
currentSize = 5 ' Starting with an initial size of 5
ReDim test(0 To currentSize - 1)
For i = 0 To currentSize - 1
test(i) = 3 + i
Next i
' Adding an additional element
currentSize = currentSize + 1
ReDim Preserve test(0 To currentSize - 1)
test(currentSize - 1) = 10
' Displaying the array elements
For i = 0 To currentSize - 1
Debug.Print test(i)
Next i
End Sub
In this example, we start with an initial size of 5 and use the ReDim Preserve
statement to resize the array while preserving the existing elements. By incrementing the currentSize
variable, we can add an additional element dynamically. 🔄
Engage with us!
We hope this blog post helped you resolve the "subscript out of range" error when populating VBA dynamic arrays. If you have any further questions or would like to share your own experiences, feel free to leave a comment below! Let's keep the conversation going! 💬
Remember to share this post with your developer friends who might be struggling with VBA dynamic arrays. Sharing is caring! 🤝
Happy coding! 🚀