How to sort an array of custom objects by property value in Swift
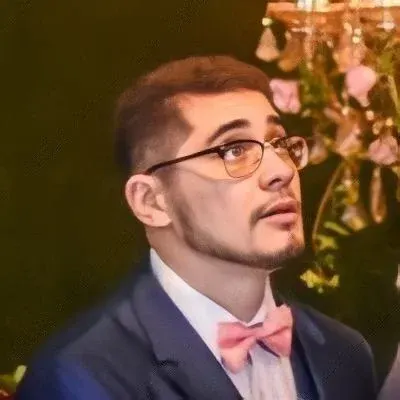

Sorting an Array of Custom Objects by Property Value in Swift
Sorting an array of custom objects by property value can be a tricky task in Swift. However, with the right approach, it can be accomplished efficiently. In this blog post, we will explore common issues faced when sorting an array of custom objects and provide easy solutions in Swift.
The Scenario
Let's consider the scenario where we have a custom class called imageFile
. This class has two properties: fileName
of type String
, and fileID
of type Int
. We have an array named images
, which contains multiple instances of the imageFile
class.
class imageFile {
var fileName = String()
var fileID = Int()
}
var images: [imageFile] = []
var aImage = imageFile()
aImage.fileName = "image1.png"
aImage.fileID = 101
images.append(aImage)
aImage = imageFile()
aImage.fileName = "image2.png"
aImage.fileID = 202
images.append(aImage)
The objective is to sort the images
array based on the fileID
property in either ascending or descending order.
Solution
To sort the images
array by the fileID
property, we can utilize the sort()
or sorted()
method available in Swift.
Sorting in Ascending Order
To sort the array in ascending order, we need to define a closure that compares the fileID
property of two objects and return the result. By using the sort()
method, we can modify the images
array directly.
images.sort { $0.fileID < $1.fileID }
Now, the images
array will be sorted in ascending order based on the fileID
property.
Sorting in Descending Order
To sort the array in descending order, we need to modify the comparison closure by swapping the <
operator with the >
operator.
images.sort { $0.fileID > $1.fileID }
After executing this line, the images
array will be sorted in descending order based on the fileID
property.
Summary
Sorting an array of custom objects by a specific property value is crucial in many Swift projects. By using the sort()
or sorted()
method in Swift and defining a closure based on the desired property, we can easily accomplish this task.
In this blog post, we addressed the common issue of sorting an array of custom objects by property value. We provided easy solutions for sorting the images
array in both ascending and descending order based on the fileID
property.
Try implementing these solutions in your own projects and experience the power of Swift's array sorting capabilities!
We hope you found this blog post helpful. If you have any questions or further insights, please leave a comment below. Happy coding! 😄🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
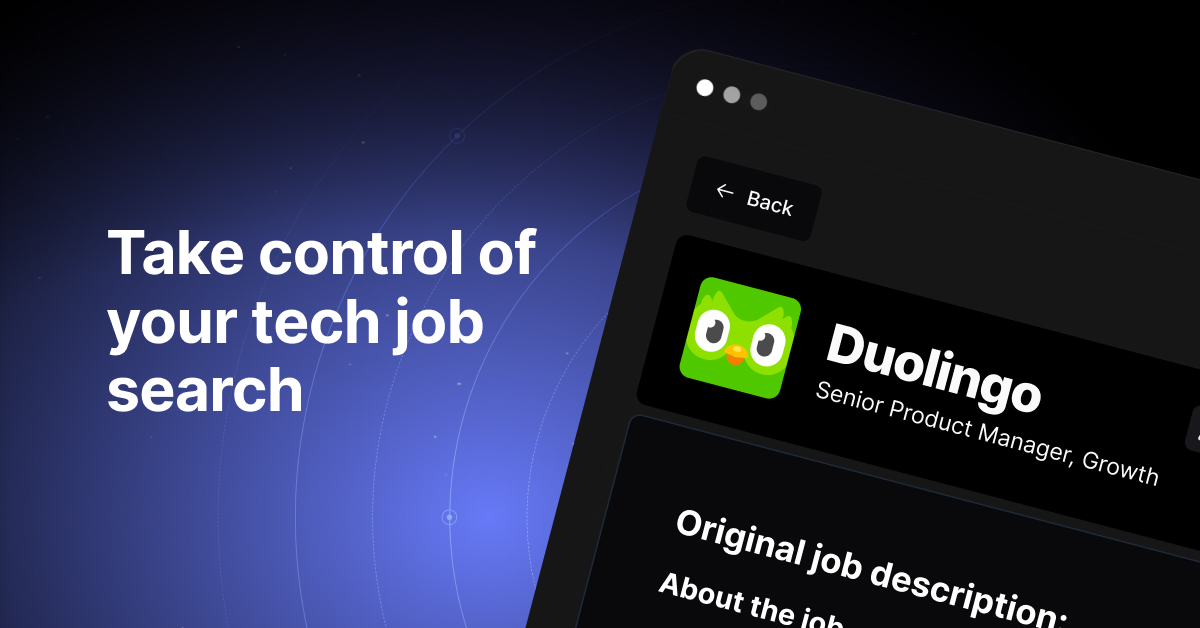