How to remove an element from an array in Swift
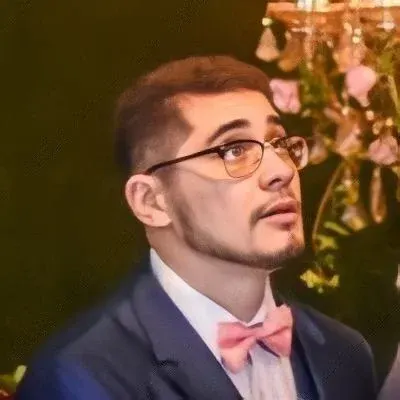
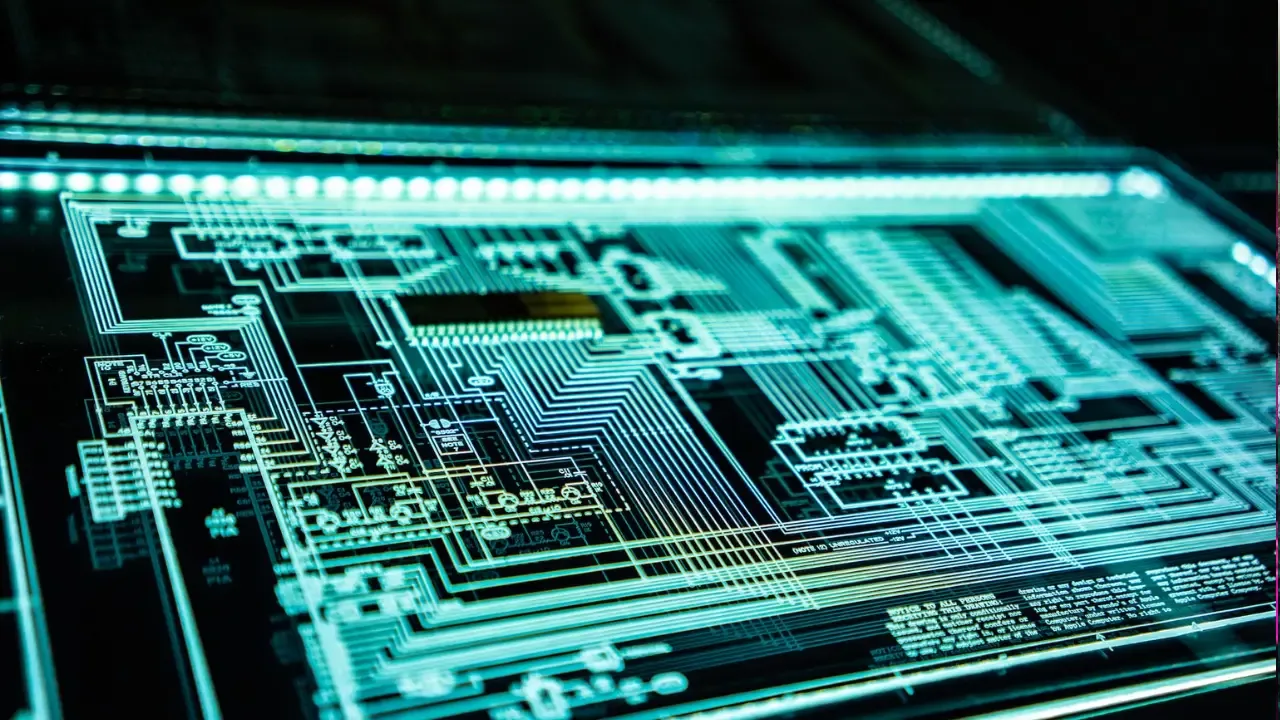
💥 Removing an Element from an Array in Swift
So, you want to kick an element out of your Swift array? No worries, I got your back! 🦾 In this guide, I'll show you how to remove an element from an array in Swift using simple and effective techniques. Let's roll! 🚀
The Scenario 🌄
Imagine you have an array called animals
with the following elements:
let animals = ["cats", "dogs", "chimps", "moose"]
Now, you have your eyes set on removing the element at index 2, which is "chimps"
. How can you do it? Hang tight, and let's dive into the solutions! 💪
Solution 1: Using the remove(at:)
Method 🗑️
Swift has a built-in method called remove(at:)
, which allows us to remove an element at a specific index in an array. Here's how you can use it to remove "chimps"
from your animals
array:
animals.remove(at: 2)
Voila! 🎉 By simply calling remove(at: 2)
, you successfully eliminated "chimps"
from the array. The method automatically adjusts the indices of the remaining elements to keep your array in order.
Solution 2: Filtering with Closures 🔍
Another approach to removing an element from an array is by filtering it out using a closure. Here's how you can do it:
animals = animals.filter { $0 != "chimps" }
In this solution, we utilize the filter
method along with a closure. The closure condition $0 != "chimps"
checks if each element in the array is not equal to "chimps"
. The filtered result is then assigned back to the animals
array, excluding the element you wanted to remove!
Ready to Rumble? 🥊
There you have it! Two simple solutions to remove an element from an array in Swift. You can use the remove(at:)
method to instantly get rid of an element at a specific index, or employ the power of filtering and closures to exclude unwanted elements.
Now it's time to put your new skills into action! Explore these techniques, experiment with Swift arrays, and have fun coding! 🤓
Did you find this guide helpful? Let me know in the comments below! And hey, share it with your developer friends if they need a hand with removing elements from arrays too! 👬👭👫
Happy coding! 😄✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
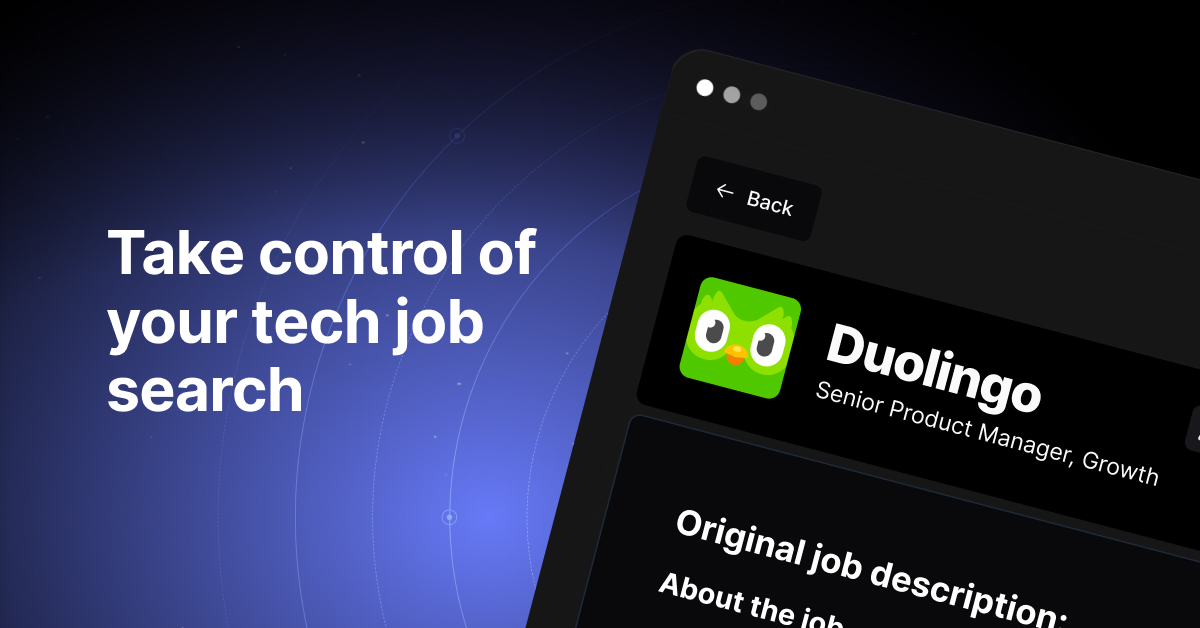