How to pass an array to a function in VBA?
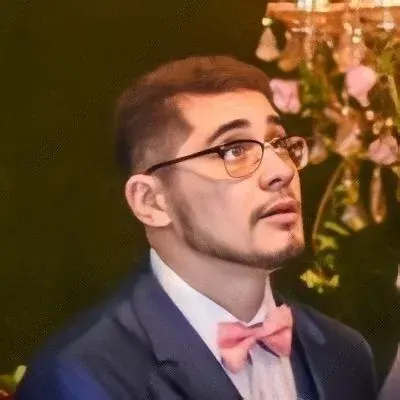
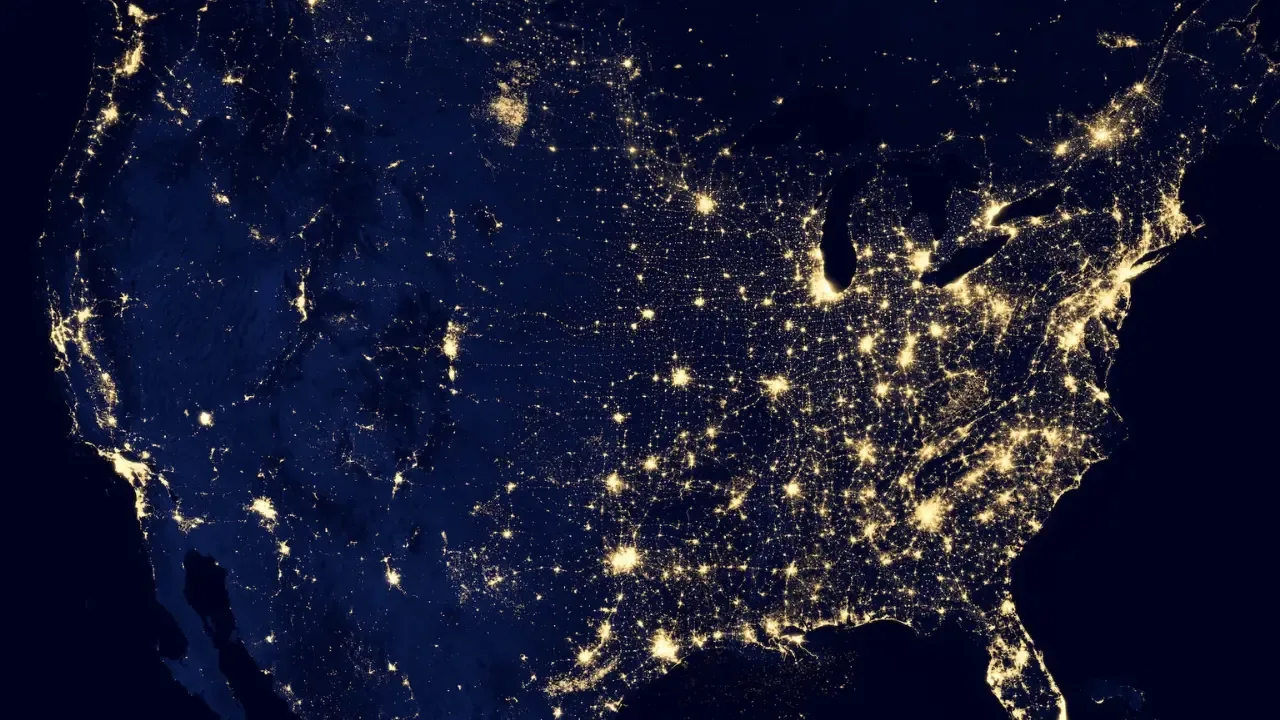
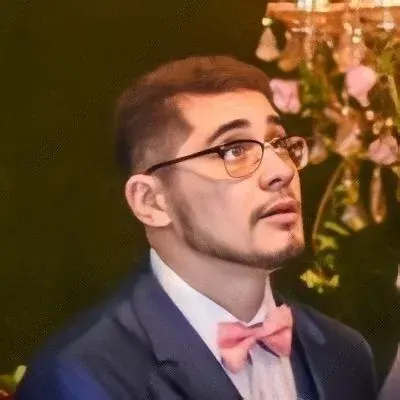
How to Pass an Array to a Function in VBA?
If you're working with VBA and trying to pass an array to a function, you might encounter some challenges. But don't worry, we're here to help! In this guide, we'll walk you through the common issues and provide easy solutions, so you can successfully pass an array to a function in VBA. Let's get started!
Understanding the Problem
In the provided context, the user is attempting to pass an array to a function called processArr
. The code snippet demonstrates the function's implementation, where it receives an array as an argument and concatenates its elements into a single string.
However, when the user tries to call the function with an array as a parameter, they encounter a compile error, "Type mismatch: array or user-defined type expected."
Analyzing the Error
The error message suggests that there is a mismatch between the expected data type and the actual argument passed to the function. In VBA, when you define a function or sub procedure parameter as an array, you should specify that it's a dynamic array using empty parentheses ()
.
Solution
To resolve this issue, let's correct the function declaration and the way we call it.
Function processArr(Arr() As Variant) As String
Dim N As Variant
Dim finalStr As String
For N = LBound(Arr) To UBound(Arr)
finalStr = finalStr & Arr(N)
Next N
processArr = finalStr
End Function
Here's how you should call the processArr
function:
Sub test()
Dim fString As String
fString = processArr(Array("foo", "bar"))
End Sub
Explanation
The corrected code snippet now declares the Arr
parameter as a dynamic array by using empty parentheses ()
. This modification allows the function to accept any number of elements in the array.
Additionally, we have fixed the concatenation logic inside the function by removing the unnecessary &
and using a simpler concatenation operator &
.
When calling the processArr
function in the test
sub procedure, we use the Array
function to create an array with the "foo" and "bar" elements. This corrected code ensures that the function call passes the array correctly, without any type mismatch errors.
Conclusion
Now that you understand how to pass an array to a function in VBA, you can confidently use this knowledge in your own projects. Remember, when working with arrays, it's crucial to declare them correctly and ensure that the function parameters and arguments match.
If you found this guide helpful or have any further questions, feel free to leave a comment below. Happy coding! 💻🚀