How to iterate a loop with index and element in Swift
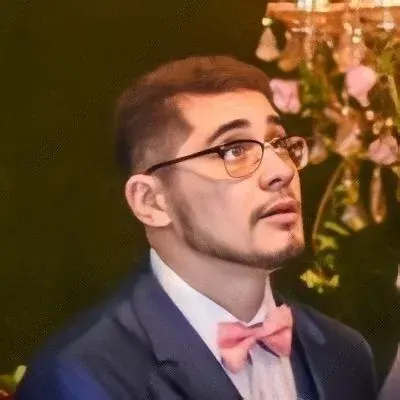
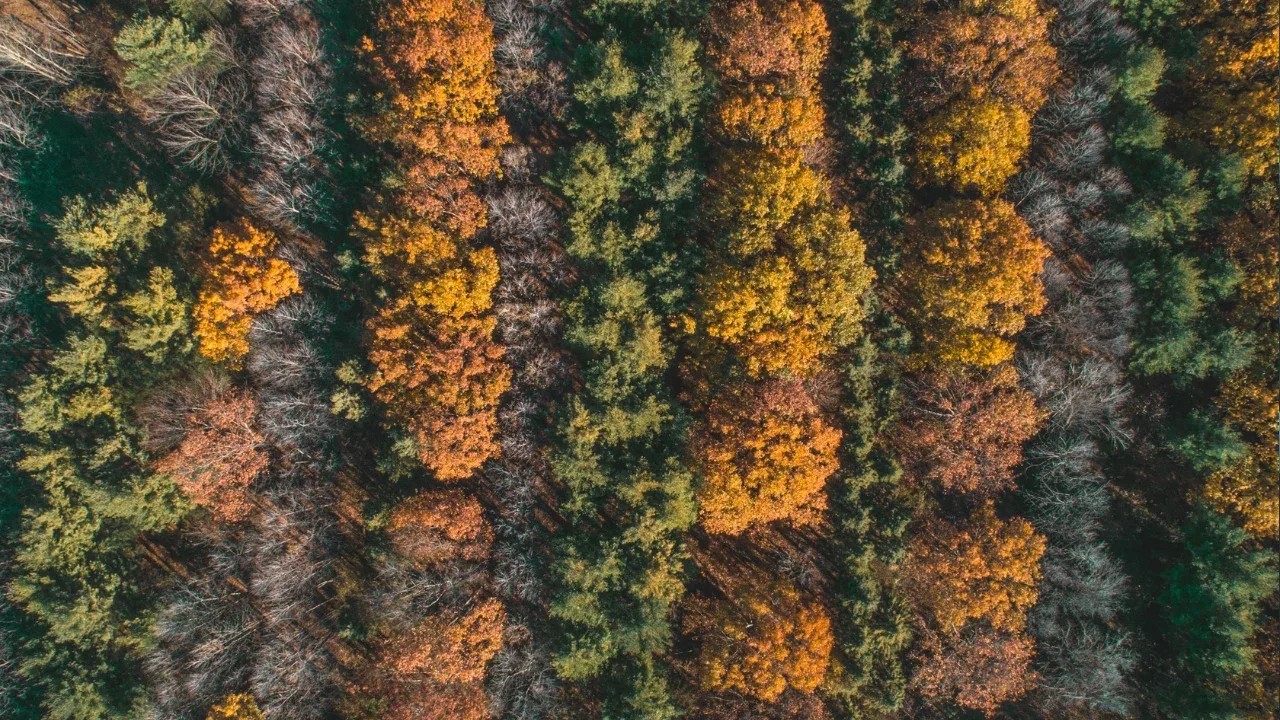
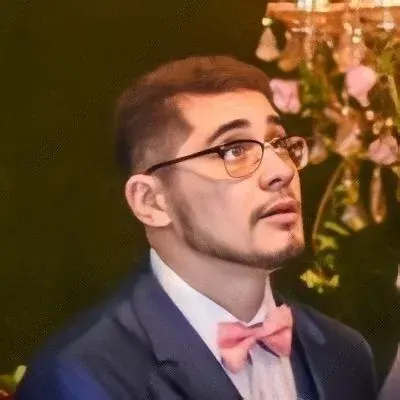
How to Iterate a Loop with Index and Element in Swift ๐๐
Are you a Swift developer looking for a way to iterate over an array and access both the index and the element? ๐คโ
In languages like Python, we have a handy function called enumerate
that allows us to achieve this, but what about Swift? ๐คทโโ๏ธ
Well, fear not! Swift provides us with a simple and elegant solution to tackle this problem. Let's dive in and explore how we can iterate a loop with index and element in Swift! ๐๐
The Problem ๐ง
Imagine you have an array of elements and you want to perform some logic on each element, but you also need to know the index at the same time. Sounds familiar, right? ๐
The Solution ๐ก
Swift gives us a neat solution by leveraging the enumerated()
method. Let's see how it works with an example. ๐งช๐ฉโ๐ป
let list = [10, 20, 30, 40, 50]
for (index, element) in list.enumerated() {
print("Index: \(index), Element: \(element)")
}
Here, we use the enumerated()
method on our array list
. This method returns a sequence of pairs, where the first element is the index and the second element is the original value. By using tuple decomposition, we can assign these values to index
and element
variables within our loop.
The output of the above code snippet would be:
Index: 0, Element: 10
Index: 1, Element: 20
Index: 2, Element: 30
Index: 3, Element: 40
Index: 4, Element: 50
Voila! We can now access both the index and element within our loop, just like the enumerate
function in Python. ๐๐
Common Pitfalls to Avoid ๐ซโ ๏ธ
While iterating a loop with index and element in Swift is straightforward, there are a few common mistakes developers fall into. Let's highlight them to save you some debugging time. โฐ๐
1. Not declaring the index properly
Make sure you define the (index, element)
tuple correctly in your loop declaration. Forgetting to include the parentheses or mixing up the order of the variables can lead to unexpected behavior. ๐โ
2. Modifying the array inside the loop
Avoid modifying the array while iterating over it. This can cause unpredictable results, as the count and order of elements may change during the loop execution. If you need to modify the array, consider making a copy or using a separate array. ๐โ
Take It a Step Further! ๐
Now that you know how to iterate a loop with index and element in Swift, why stop here? ๐ฅ
Think of how this powerful technique can be applied to your own projects. Maybe you need to perform some calculations based on the index, or perhaps you want to access adjacent elements while iterating. The possibilities are endless! ๐
Conclusion ๐
In this guide, we explored how to iterate a loop with index and element in Swift, just like Python's enumerate
function. We learned how to use the enumerated()
method to achieve this and discussed some common pitfalls to avoid.
So go ahead, iterate your Swift arrays with confidence, knowing that you have the power to access both the index and the element! ๐ช๐ป
If you found this guide helpful, make sure to share it with your friends who are also Swift developers. Together, we can make the Swift community even stronger! ๐ค๐
Now it's your turn! Have you encountered any other useful tips while iterating loops in Swift? Share your thoughts and experiences in the comments below. Let's learn from each other! ๐ฌ๐
Happy coding! ๐๐ป