How to find index of list item in Swift?
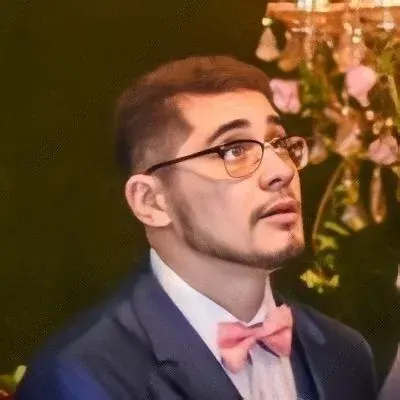
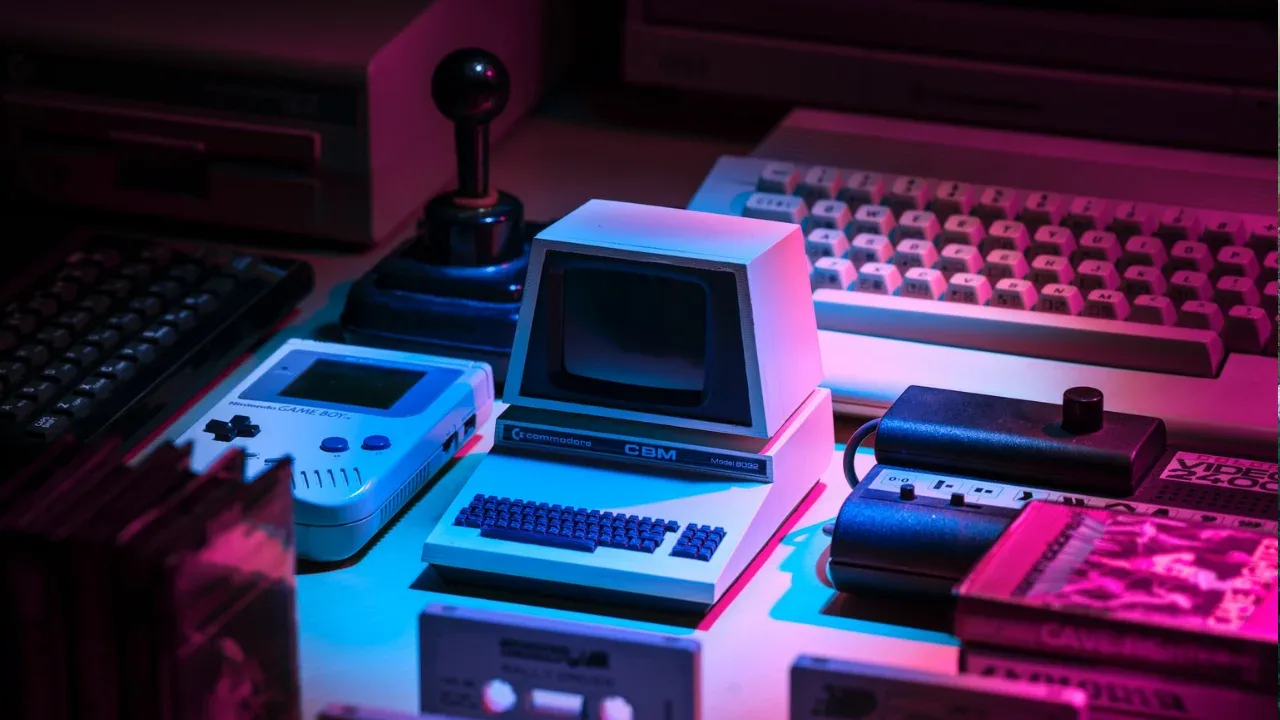
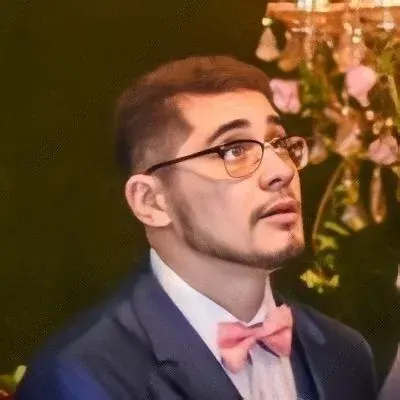
How to Find the Index of a List Item in Swift?
š Looking for an easy way to find the index of an item in a list using Swift? You're in luck! In this blog post, we'll explore how to achieve just that. We'll tackle the common issue of finding the index of a specific item in a list and provide you with a simple solution. Let's dive right in! šŖ
š¤ The Problem:
So, you're trying to find the š index
of an item in a list, but you're not sure how to do it in Swift. Although there is a list.StartIndex
and list.EndIndex
, you are searching for something similar to Python's list.index("text")
.
š§ The Solution:
In Swift, you can use the firstIndex(of:)
method to find the index of an item in a list. This method returns an optional value, which means it will return nil
if the item is not found in the list. Here's how you can use it:
let list = ["apple", "banana", "orange", "kiwi"]
let itemToFind = "banana"
if let index = list.firstIndex(of: itemToFind) {
print("The index of \(itemToFind) is \(index).")
} else {
print("Item not found in the list.")
}
⨠Explanation:
In the code snippet above, we have a list of fruits stored in the list
variable. We want to find the index of the item "banana". By using the firstIndex(of:)
method, we pass in the item we want to find as the argument. If the item is found in the list, the method will return the index of the first occurrence. We use optional binding (if let
) to safely unwrap the optional value and print the index. If the item is not found, the else
block will be executed, notifying us that the item was not found in the list.
š Call-to-Action:
Finding the index of a list item is a common task when working with arrays in Swift. Now that you know how to do it using the firstIndex(of:)
method, give it a try in your own projects! Leave a comment below and let us know if you found this guide helpful. Share this blog post with your fellow Swift developers and help them out too! š
Happy coding! š