How to check if an element is in an array
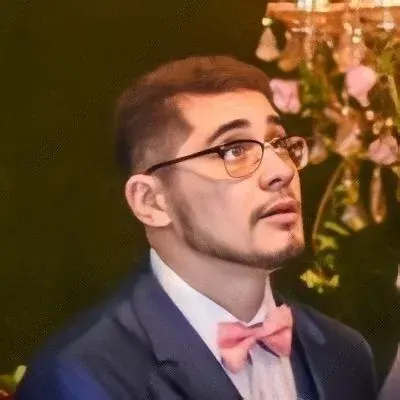
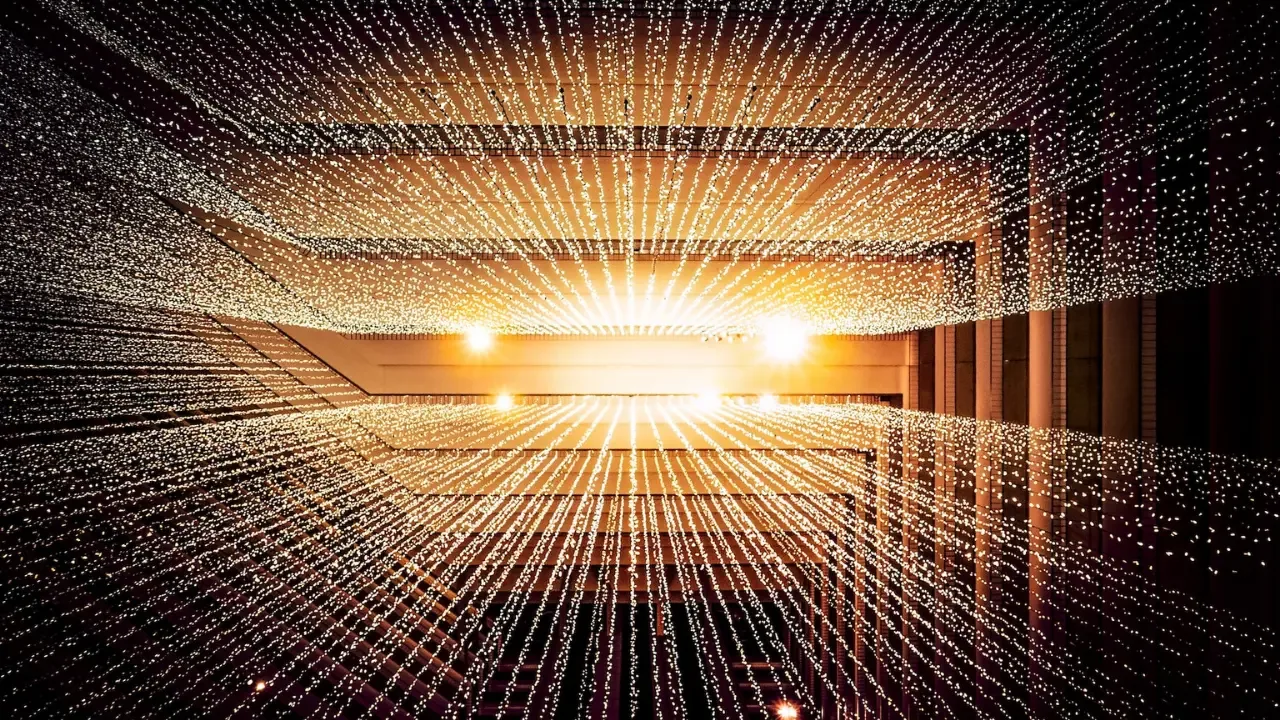
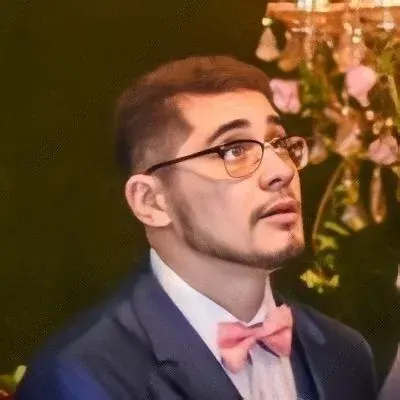
How to Check if an Element is in an Array
So, you're working with Swift and you need to determine whether a specific element exists in an array. Xcode isn't giving you any suggestions for methods like contain
, include
, or has
. Don't worry, we've got you covered! In this blog post, we'll explore the different solutions to this problem and provide you with easy-to-implement code snippets.
The Challenge
Let's assume you have an array called elements
containing some numbers:
var elements = [1, 2, 3, 4, 5]
You want to check if the number 5
exists in this array, and if it does, you want to perform some action.
The Solution
Thankfully, Swift provides a built-in method called contains
that allows you to check if an element is present in an array. Here's how you can use it:
if elements.contains(5) {
// do something
}
In the above example, we're using the contains
method to check if 5
exists in the elements
array. If it does, the code block inside the if
statement will be executed.
A Compelling Call-to-Action
Now that you know how to check if an element is in an array, the possibilities are endless! You can use this knowledge in various scenarios, such as filtering arrays, performing actions on specific elements, and so on.
Try implementing this logic in your own Swift projects and let us know how it goes! Share your experiences in the comments section below and engage with our community of passionate Swift developers.
Remember, understanding the core concepts and built-in methods of a programming language is essential for efficient coding. 🔥
Keep calm and code in Swift! 🚀