How do I shuffle an array in Swift?
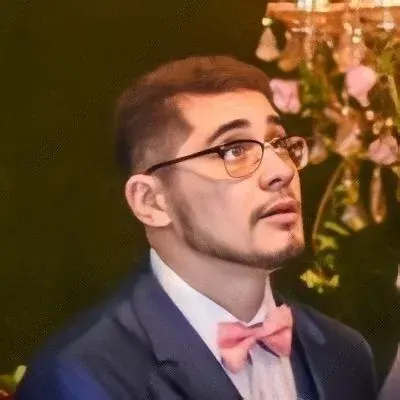
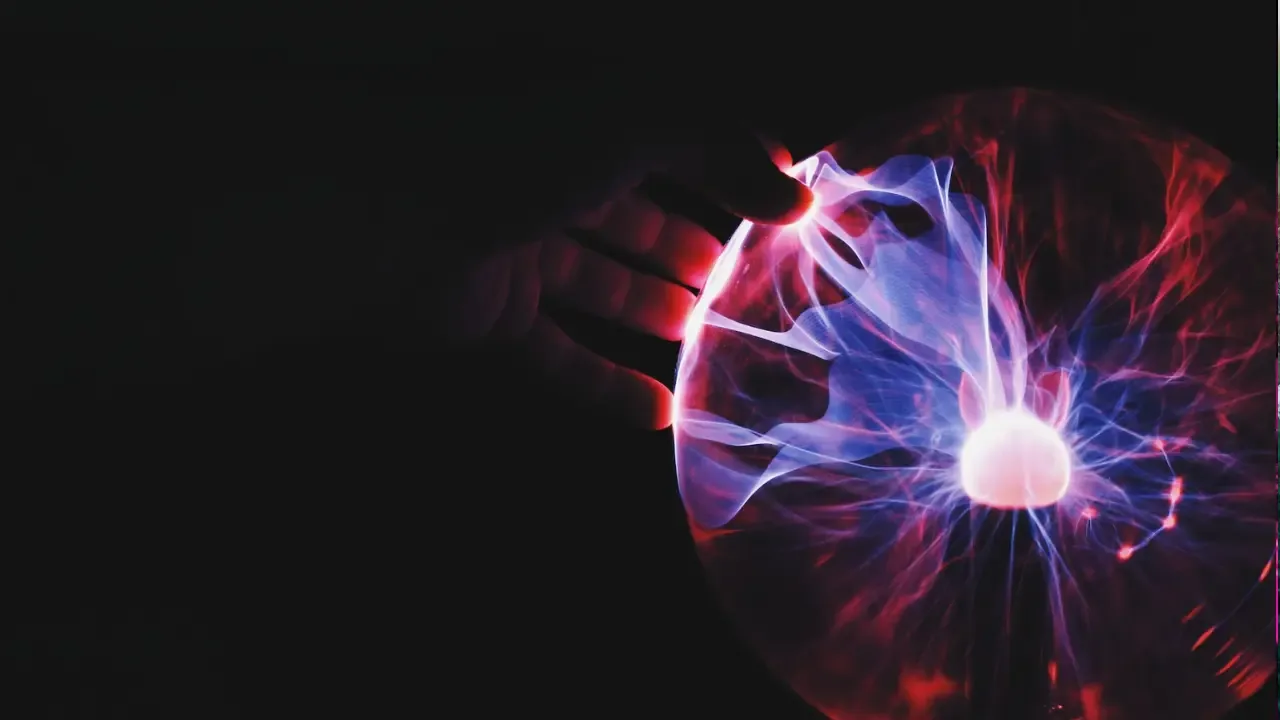
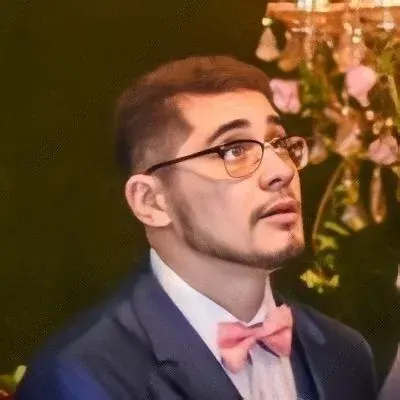
How to Shuffle an Array in Swift: A Simple Guide ๐ฒ
Do you want to add some randomness and excitement to your Swift programming? Imagine having a deck of playing cards and being able to shuffle them effortlessly. In this blog post, weโll explore how to shuffle an array in Swift, using both the .shuffle()
and .shuffled()
methods.
The Challenge ๐
So, you have an array (letโs call it myArray
) and you want to randomly rearrange its elements. Letโs take the example of a deck of 52 playing cards. You need to shuffle the deck, allowing for a fair and random distribution of cards.
The Solution: .shuffle()
Versus .shuffled()
๐ก
Fortunately, Swift provides two different methods that can help you achieve the desired result. But what's the difference between .shuffle()
and .shuffled()
? Let's find out!
1. Using .shuffle()
to Shuffle an Array ๐
The .shuffle()
method is used to shuffle the elements of an array in place. Letโs take a look at some code:
var myArray = [1, 2, 3, 4, 5]
myArray.shuffle()
print(myArray)
In the above example, the shuffle()
method is called on myArray
. It rearranges the elements randomly, ensuring a unique order each time the code is executed. The output may look something like this: [2, 4, 5, 1, 3]
.
2. Using .shuffled()
to Create a Shuffled Array ๐
Unlike .shuffle()
, the .shuffled()
method doesnโt modify the original array in place. Instead, it returns a new array with the elements shuffled. Hereโs an example:
let myArray = [1, 2, 3, 4, 5]
let shuffledArray = myArray.shuffled()
print(shuffledArray)
In this code snippet, myArray
remains unchanged, and a new array shuffledArray
is created with the shuffled elements. The output might look like this: [4, 5, 2, 1, 3]
.
Conclusion and Call-to-Action ๐
Now that you know how to shuffle an array in Swift using both the .shuffle()
and .shuffled()
methods, you can add randomness and unpredictability to your programs. Whether itโs shuffling a deck of cards or randomizing the order of items in an array, these methods will help you achieve your goal.
We hope you found this guide helpful and enjoyable! If you have any questions or would like to share your own experiences with array shuffling in Swift, feel free to leave a comment below. Happy coding! ๐๐