How do I remove an array item in TypeScript?
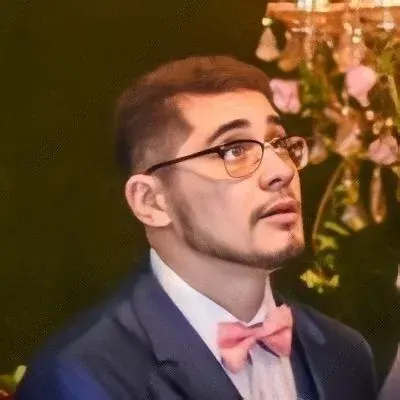
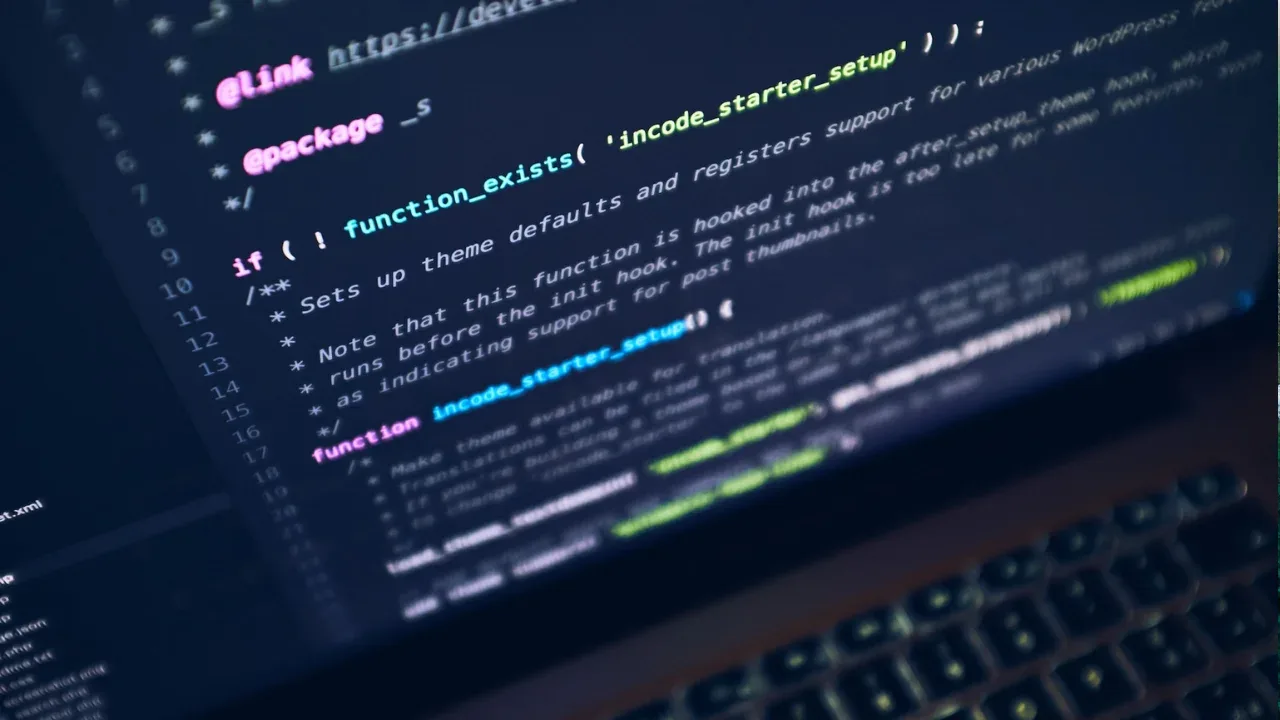
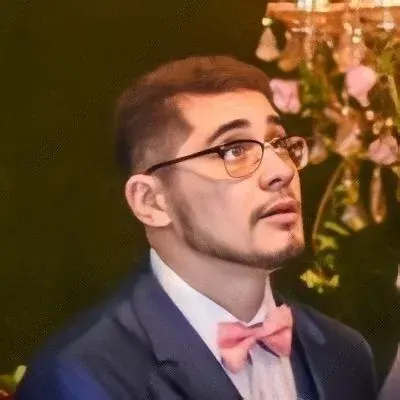
Hey there, tech enthusiasts! 😎 Are you facing a common conundrum in TypeScript? Wondering how to remove an array item when you already have the key? Fear not! 🚀 In this blog post, we'll tackle this challenge head-on, providing you with some easy solutions and expert tips to get the job done. Let's dive in! 💪
The Problem: Removing an Array Item with a Key in TypeScript
So, you've got an array, and each item in that array has a unique key. Now, you want to remove a specific item using its key. But how the heck do you do that in TypeScript? Well, fret not, my friend! We've got a few tricks up our sleeves to help you out.
Solution 1: Using the filter
Method
One way to remove an array item with a key is by using the filter
method. This method creates a new array, excluding the items that match your specified condition. Here's how you can use it:
const array = [
{ key: 'a', value: 'apple' },
{ key: 'b', value: 'banana' },
{ key: 'c', value: 'cherry' },
];
const keyToRemove = 'b';
const newArray = array.filter((item) => item.key !== keyToRemove);
In this example, we have an array of objects, where each object has a key
and a value
. We want to remove the item with the key 'b'
. By using the filter
method, we create a new array called newArray
that excludes the item with the specified key.
Solution 2: Utilizing the Spread Operator
Another nifty solution to remove an array item with a key is by using the spread operator. This operator allows us to create a new array while easily omitting the desired item. Take a look at this snippet:
const array = [
{ key: 'a', value: 'apple' },
{ key: 'b', value: 'banana' },
{ key: 'c', value: 'cherry' },
];
const keyToRemove = 'b';
const newArray = [...array.filter((item) => item.key !== keyToRemove)];
Here, we employ the spread operator (...
) to create a new array, copying all the items from the original array except the one with the specified key.
Solution 3: Using the splice
Method
Last but not least, we have the trusty splice
method to help us out. This method modifies the array in place, removing the desired item based on the provided key. Let's check it out:
const array = [
{ key: 'a', value: 'apple' },
{ key: 'b', value: 'banana' },
{ key: 'c', value: 'cherry' },
];
const keyToRemove = 'b';
for (let i = 0; i < array.length; i++) {
if (array[i].key === keyToRemove) {
array.splice(i, 1);
break;
}
}
In this example, we iterate over the array and use an if
condition to check if the current item's key matches the one we want to remove. Once we find a match, we use the splice
method to remove that item from the array.
Wrapping Up
And there you have it! 😃 Three simple solutions to remove an array item when you already have the key in TypeScript. Whether you prefer the versatility of the filter
method, the elegance of the spread operator, or the directness of the splice
method, you now have the tools to tackle this problem with confidence.
Remember, practice makes perfect! So, try out these solutions in your next TypeScript project and see which one fits your needs the best. Happy coding! 💻🎉
Got any questions or other cool TypeScript tricks up your sleeve? Share them in the comments below and let's keep the conversation flowing. 👇🤩