How do I concatenate or merge arrays in Swift?
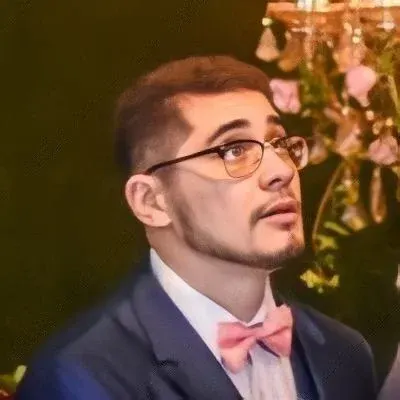
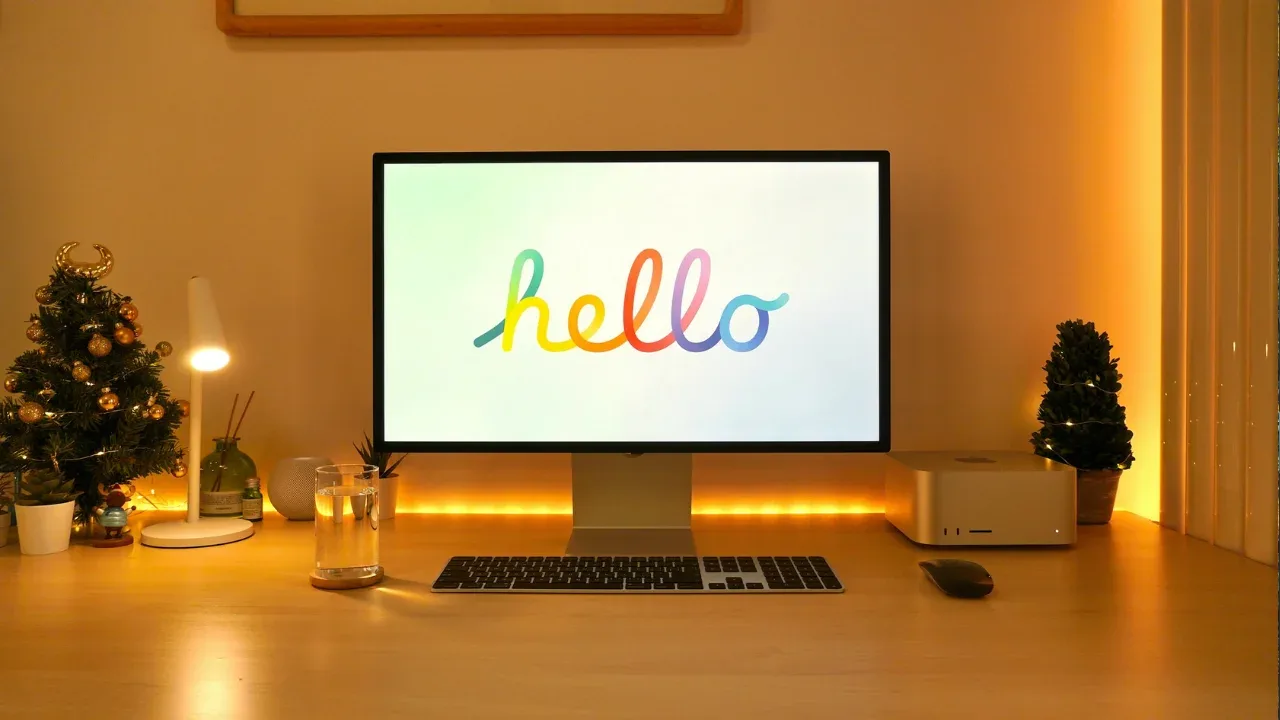
Concatenating Arrays in Swift: A Quick and Easy Guide! 🧩
As a Swift developer, you might often find yourself needing to merge or concatenate arrays. Whether you're working on an iOS app or a macOS project, combining arrays is a common operation that can be a bit tricky if you're new to the language. But don't worry! In this blog post, we'll explore different techniques and provide simple solutions to help you conquer this challenge. Let's dive in! 🚀
The Challenge 💥
Imagine you have two arrays in Swift:
var a: [CGFloat] = [1, 2, 3]
var b: [CGFloat] = [4, 5, 6]
Your goal is to merge these arrays into a single array: [1, 2, 3, 4, 5, 6]
.
Solution 1: Using the +
Operator 🤝
One straightforward approach to concatenate arrays in Swift is by using the +
operator. This is similar to how you concatenate strings. You can simply add the two arrays together to create a new array that contains all the elements from both arrays:
let mergedArray = a + b
print(mergedArray) // Output: [1, 2, 3, 4, 5, 6]
In this example, we created a new array called mergedArray
by combining the contents of arrays a
and b
. It's as easy as that! 😎
Solution 2: Using the +=
Operator 🆙
Alternatively, you can use the +=
operator to merge arrays in place. This means that the original array will be modified to include the elements from the second array. Here's how you can achieve this:
a += b
print(a) // Output: [1, 2, 3, 4, 5, 6]
In this example, we modified the array a
directly by appending the contents of array b
to it. This approach can be useful when you want to save memory or don't need to keep the original arrays intact.
Solution 3: Using the append(contentsOf:)
Method 🧩
Another approach to concatenate arrays in Swift is by using the append(contentsOf:)
method. This method allows you to add the elements from one array to another, preserving the original arrays. Here's how you can do it:
var mergedArray = a
mergedArray.append(contentsOf: b)
print(mergedArray) // Output: [1, 2, 3, 4, 5, 6]
In this example, we created a new array called mergedArray
and added the contents of array b
to it using the append(contentsOf:)
method. This technique can be useful when you want to keep the original arrays untouched while creating the merged array.
🎉 Let's Step Up Your Swift Game! 🎉
Congratulations on learning different techniques to concatenate or merge arrays in Swift! Now you can efficiently combine arrays and make your code more efficient and readable. But don't stop here, dive deeper into the fascinating world of Swift and explore other exciting topics.
If you found this blog post helpful, feel free to share it with your fellow Swift enthusiasts. And don't forget to subscribe to our newsletter for more delightful content delivered straight to your inbox! Happy coding! 😊💻
Do you have any other tips or tricks for merging arrays in Swift? Share your thoughts in the comments below! Let's learn from each other! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
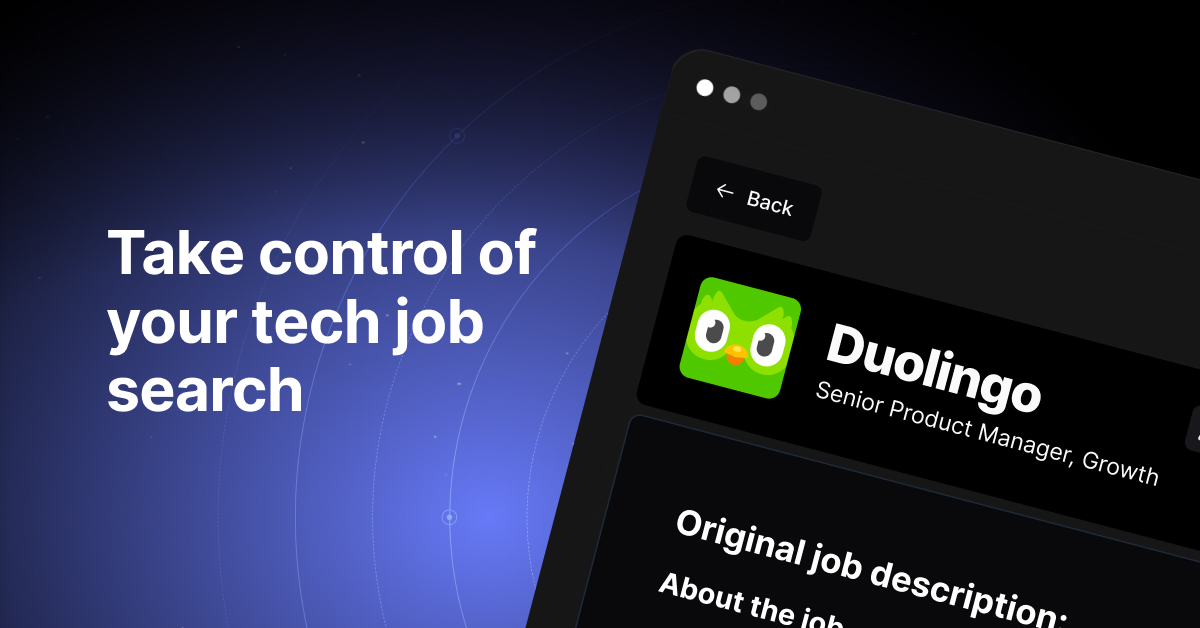