How can I extend typed Arrays in Swift?
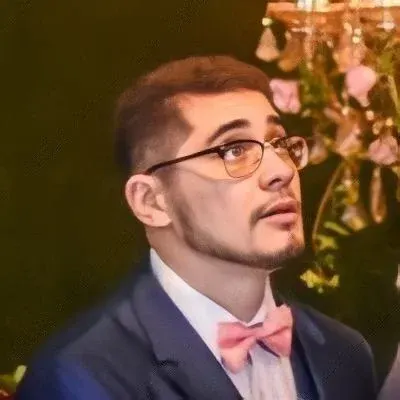
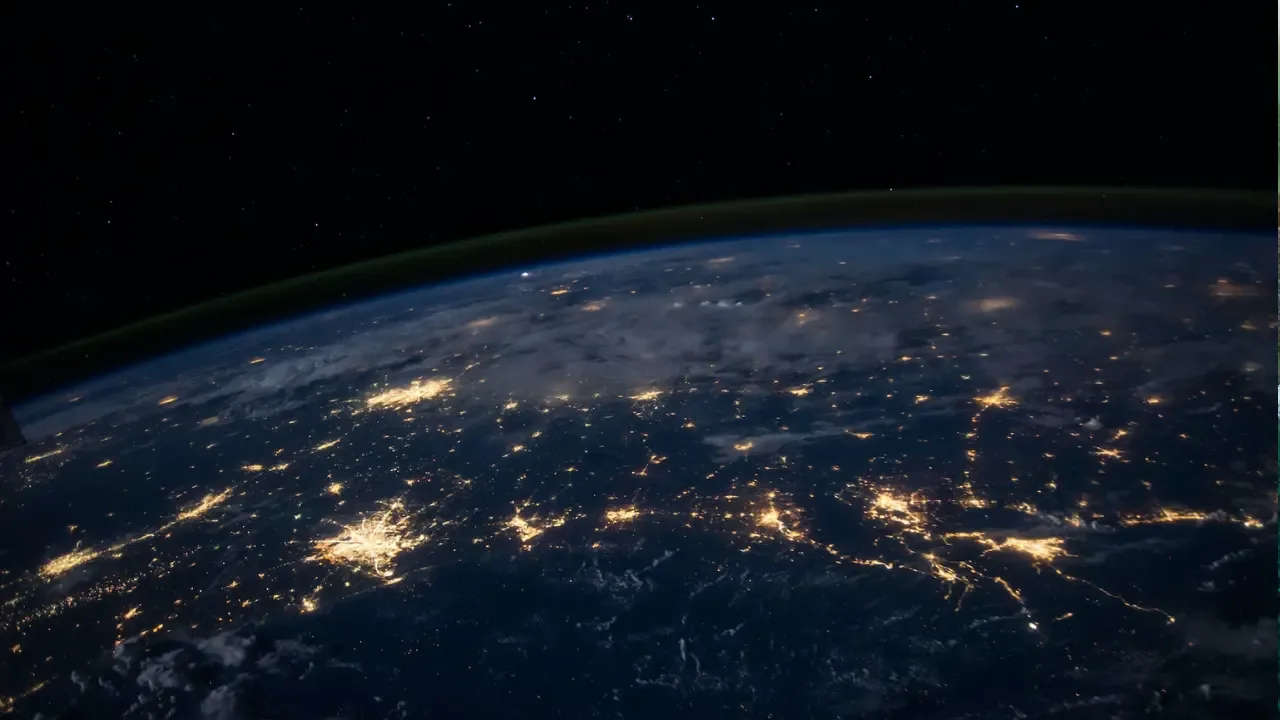
How to Extend Typed Arrays in Swift
So you want to extend Swift's Array<T>
or T[]
type with your own custom functional utilities? You've come to the right place! In this guide, we'll address the common issues you might face and provide easy solutions to extend typed arrays in Swift.
The Error Message: "Nominal type T[]
can't be extended"
You might have tried copying and pasting the code snippets mentioned in the question, but encountered the error message: "Nominal type T[]
can't be extended." This is because Swift's type inference system is struggling to interpret the generic type T
.
Solution: Extend Typed Arrays using a Protocol
Instead of directly extending the Array<T>
type, we can extend a protocol that represents Array<T>
. Swift's API docs reveal that Array methods are an extension of the ArrayType
. We can create our own extension of ArrayType
to add our custom functional utilities.
extension ArrayType {
// Add your custom functional utilities here
}
Now, you can define your own utility methods inside this extension, ensuring that they work with any array type T[]
.
The Error Message: "Use of undefined type 'T'"
If you tried extending the Array<T>
type directly, using the full type definition extension Array<T>
, you may have encountered the error message: "Use of undefined type 'T'."
Solution: Use a Concrete Type Instead of Generic Type
To resolve this issue, you can try using a concrete type instead of a generic type. Instead of Array<T>
, you can extend Array<Int>
, Array<String>
, or any other specific array type. This way, the compiler can infer the type correctly, and the extension will work flawlessly.
extension Array<Int> {
// Add your custom functional utilities here
}
Remember, this solution works if you want to extend specific types of arrays. If you need a generic extension that works with any array type T
, continue reading for an alternative solution.
The Error Message: "Cannot specialize non-generic type 'Array'"
You might have tried to create a generic extension by using Array<T>
and encountered the error message: "Cannot specialize non-generic type 'Array'."
Solution: Extend the Non-generic Array
Type
The Array
type in Swift is not itself generic. Instead, it becomes generic based on the type of its elements (T
). So, to create a generic extension for all array types, you need to extend the non-generic Array
type.
extension Array {
// Add your custom functional utilities here
}
This solution allows you to write utility methods that work with any array type, like [Int]
, [String]
, or even [Any]
. However, keep in mind that you won't have direct access to the generic type T
inside the extension.
The Challenge: Maintaining Type Information Inside Generic Extensions
Now, let's address an interesting challenge faced when trying to maintain type information inside generic extensions. You might have tried to create a generic extension that behaves like Swift's built-in extensions, but faced issues with type casting and accessing elements.
Solution: Preserve Type Information Through Type Erasure
To preserve type information inside a generic extension, you can use a technique called type erasure. The idea is to wrap the generic type T
into a type-erased container, so you can maintain the type information even when flowing through methods.
Here's an example of how you can implement a type-erased container and a find
method that works with any array element type:
struct AnyElement {
let value: Any
}
extension Array {
func find<T>(fn: (T) -> Bool) -> [T] {
var result = [T]()
for element in self {
if let typedElement = element as? T, fn(typedElement) {
result.append(typedElement)
}
}
return result
}
}
In this example, we wrap each element of the array in an AnyElement
struct, preserving its type information. Inside the find
method, we can then safely cast the elements back to their original type (T
) and perform any required operations.
Now you can call the find
method on arrays with different element types:
let array = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
let filteredArray = array.find { $0 > 5 }
print(filteredArray) // Output: [6, 7, 8, 9, 10]
let stringArray = ["A", "B", "C", "D", "E"]
let filteredStringArray = stringArray.find { $0 > "B" }
print(filteredStringArray) // Output: ["C", "D", "E"]
Feel free to customize the find
method or add more utility methods to the generic extension based on your requirements.
Conclusion
Extending typed arrays in Swift is possible by extending the corresponding protocols or the non-generic Array
type. By using type erasure, you can maintain type information inside generic extensions and create utility methods that work with any array element type.
Now that you've learned how to extend typed arrays in Swift, it's time to put your new knowledge into action. Start experimenting, creating your own functional utilities, and making your Swift code more expressive and powerful.
If you found this guide helpful or have any additional insights or questions, we would love to hear from you! Leave a comment below and let's continue the conversation. Happy coding! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
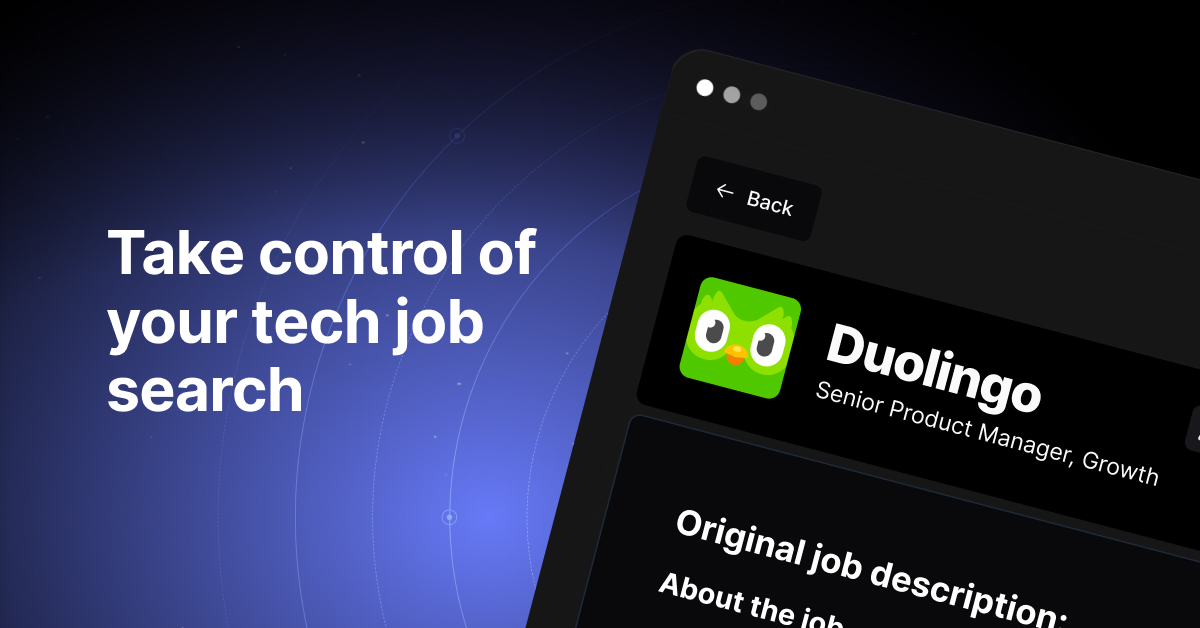