Finding sum of elements in Swift array
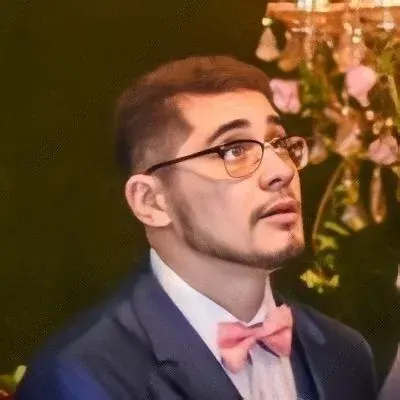
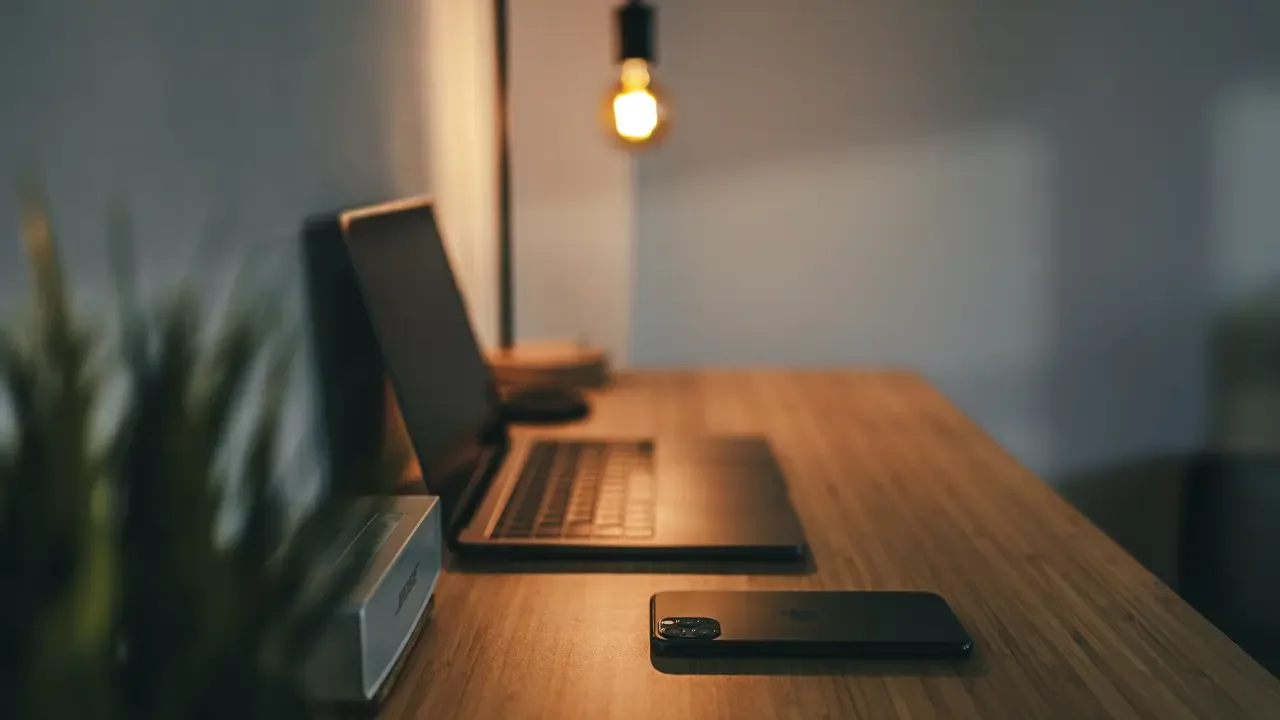
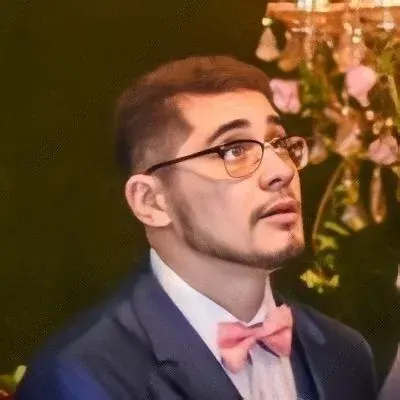
The Swift Sum of Elements Dilemma: Solving it the Easy Way! πͺ
So, you find yourself faced with the perplexing challenge of finding the sum of elements in a Swift array, huh? Fear not, my friend! We've got your back and we're here to guide you through this mathematical journey with ease. π
The Problem at Hand π€
Let's set the stage: you have an array called multiples
which holds a collection of integers, and your ultimate aim is to find the sum of these integers. Sounds simple enough, right? Well, sometimes things are not as straightforward as they seem in the world of Swift. π‘
Coming to the Rescue: Easy Solutions! π¦ΈββοΈ
Solution 1: Good ol' For Loop π
One classic approach is to use a for-in
loop to iterate through each element in the array and add it to a running total. Here's an example:
var sum = 0
for number in multiples {
sum += number
}
print("The sum of the multiples is: \(sum)")
Solution 2: The Power of Higher-order Functions π§ββοΈ
Alternatively, Swift offers us robust higher-order functions, like reduce
, that can make our lives a whole lot easier when it comes to calculating sums. Take a look at this concise solution:
let sum = multiples.reduce(0, +)
print("The sum of the multiples is: \(sum)")
By using reduce
, we pass an initial value of 0
(the starting point of our sum) and the +
operator to perform addition on the elements. Swift does all the heavy lifting for us, making this a super elegant solution. π
Dealing with Potential Pitfalls! π§
Issue 1: Empty Arrays π«
What happens if your multiples
array is empty? Well, in that case, both solutions we've suggested will give you a sum of 0
. So, make sure to handle this scenario in your code accordingly! π οΈ
if multiples.isEmpty {
print("The array is empty. Unable to find the sum.")
} else {
// Proceed with your chosen solution
}
Issue 2: Non-integer Values π’
If you encounter elements in your array that are not integers, you might end up with unexpected results or even runtime errors. Ensure that all elements in your multiples
array are indeed integers, or handle any non-integer values explicitly. Safety first! π§ͺ
Join the Swift Sum Community! π₯
We hope this guide has helped you find clarity amidst the array-summing chaos in Swift! Remember, there's always more to learn, so why not join our engaging community of Swift enthusiasts?
π Share your thoughts and experiences in the comments below. π Follow us on social media for more Swift hacks and tricks. π Stay tuned for future blog posts to expand your Swift knowledge!
Now, go forth and conquer those arrays! π