Creating an Array from a Range in VBA
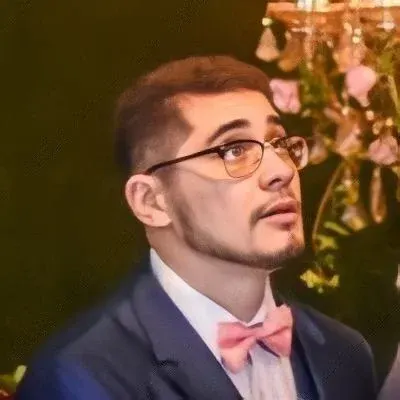

Creating an Array from a Range in VBA: A Hassle-Free Guide 😎📊💡
Are you struggling to create an array in VBA from a range of cells? 🤔 Don't worry, you're not alone! Many folks face this seemingly basic problem and struggle to find a straightforward solution. But fear not, because we've got you covered. In this guide, we'll address the common issues and provide you with easy-to-implement solutions. So let's dive right in! 💪
The Problem: Loading Range Contents into an Array 🔄📉
The primary issue arises when you attempt to create an array from a range of cells using the Array
function, like this:
DirArray = Array(Range("A1:A2"))
✋ Oops! This approach doesn't work as expected and often leads to frustration. Instead of loading the entire range into a single array, you end up with an array containing only one element that is the entire range.
The Solution: Looping Through the Range 🔄🔁
To properly load a range into an array without individually enumerating each cell, you need to loop through the range. Here's a snippet that demonstrates how you can achieve this:
Dim rng As Range
Dim DirArray() As Variant
Dim cell As Range
Dim i As Long
Set rng = Range("A1:A2") ' Replace with your actual range
ReDim DirArray(1 To rng.Cells.Count)
i = 1
For Each cell In rng
DirArray(i) = cell.Value
i = i + 1
Next cell
🚀 And there you go! By looping through the range, we assign each cell's value to the corresponding element of the array. We utilize the ReDim
statement to dynamically resize the array based on the count of cells in the range.
Handling Varying Range Lengths 📏🔀
If your range varies in length, don't fret! The above solution works regardless of the range's size. The array is dynamically resized to accommodate the number of cells in the range. You can now focus on your data, without worrying about the range length!
Verifying Array Length: The UBound
Function 📏🔍
To make sure your array has been correctly populated and obtain the array's length, you can use the UBound
function. Here's how:
MsgBox UBound(DirArray, 1)
🔔 The UBound
function returns the upper bound of the specified dimension (1 in this case), allowing you to determine the length of your array accurately. It's always a good idea to verify your array's length for better validation.
Your Turn: Share Your Experience! 🗣️💬
We hope this guide helped you effortlessly create an array from a range in VBA. 🎉 Have you encountered any other roadblocks while working with arrays or VBA? Share your experience in the comments section below! 👇 We'd love to hear from you and help you out. Let's learn from each other! 😉
So what are you waiting for? Give it a try and let us know your thoughts. Happy coding, and stay tuned for more exciting tech tutorials! 🙌💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
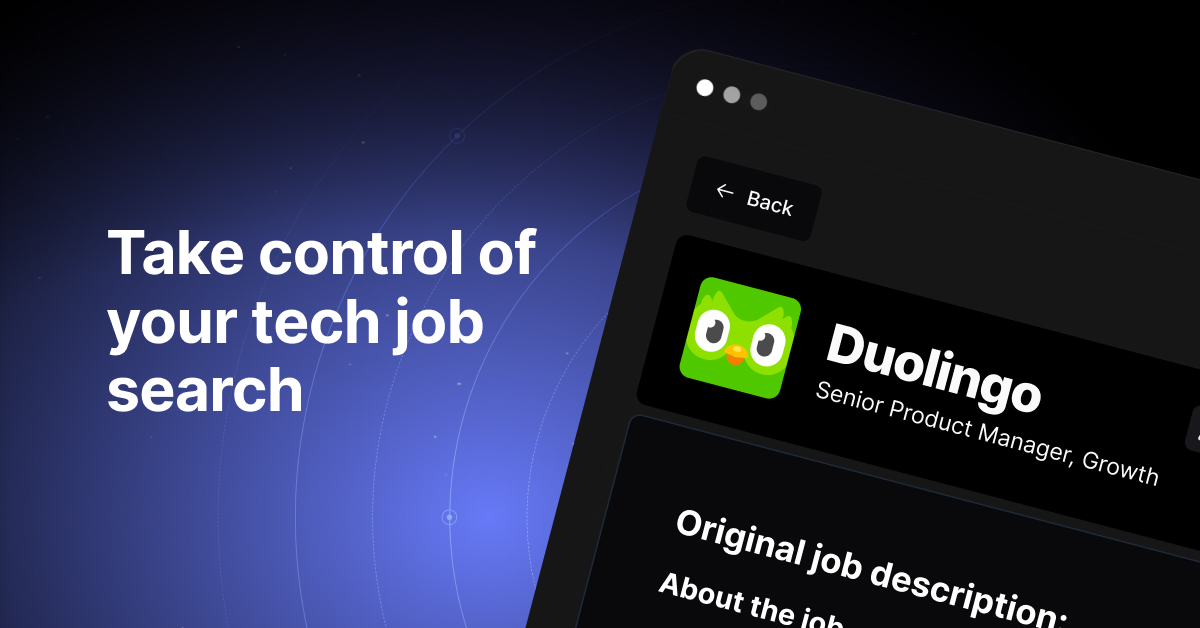