Check if a Bash array contains a value
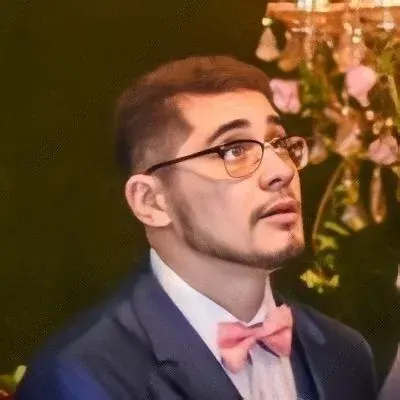
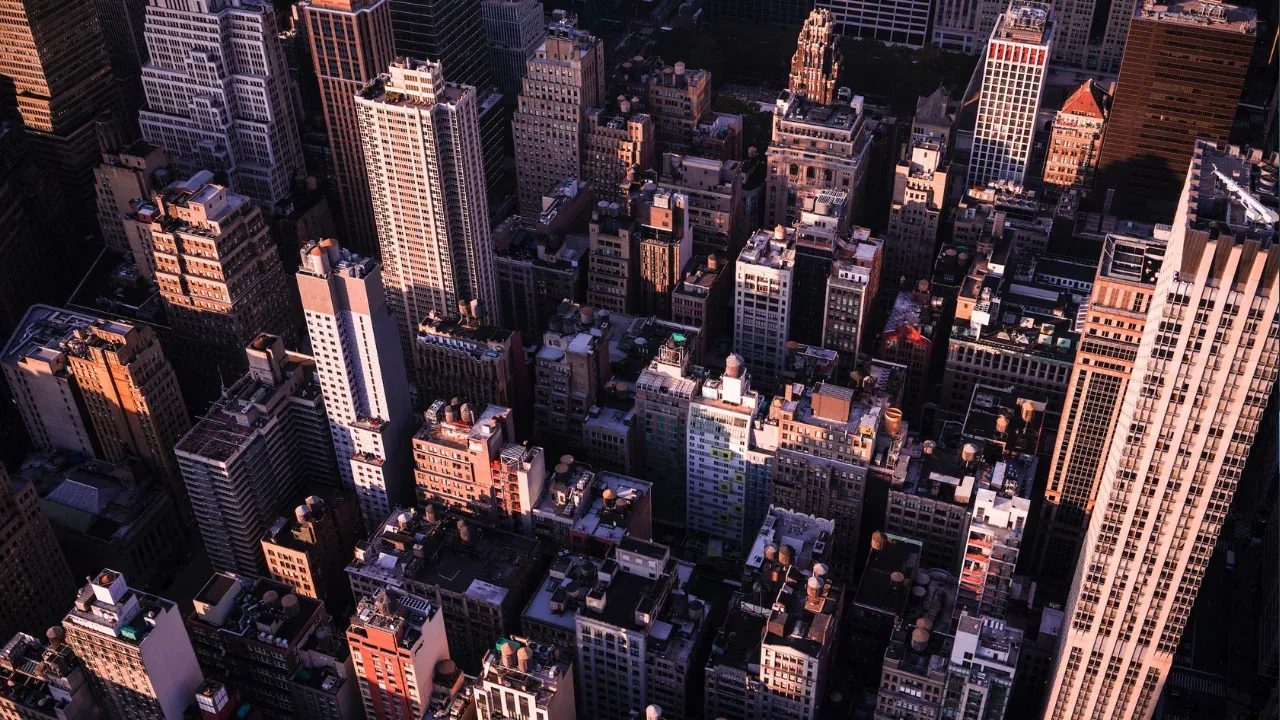
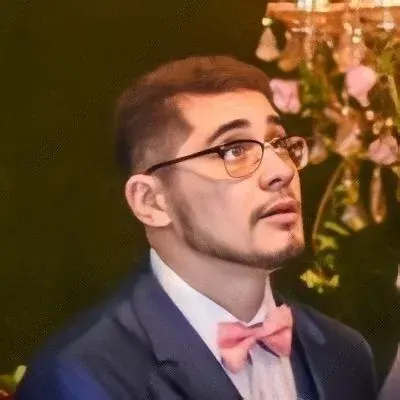
How to Check if a Bash Array Contains a Value 💭💡🖥️
If you are a Bash scripting enthusiast, you may have encountered the need to check if a certain value exists in an array. Whether you are manipulating data or performing conditional actions, this common issue can be resolved in a few easy steps. In this blog post, I'll guide you through the process of checking if a Bash array contains a value, providing you with simple solutions to accomplish this task. Let's dive in! 🏊♀️🚀
The Problem ⚠️🧐
The question that often arises is: "In Bash, what is the simplest way to test if an array contains a certain value?" 🤔
The Solution 🔍✅
To check whether a Bash array contains a specific value, we can utilize a loop to iterate over the array elements and compare each element with the value we are seeking. Here's an example of how you can do this:
#!/bin/bash
# Declare an array
my_array=("apple" "banana" "cherry" "date")
# Value to check
value="banana"
# Loop through array elements
for element in "${my_array[@]}"; do
# Check if element is equal to the desired value
if [ "$element" == "$value" ]; then
echo "Array contains $value!"
# Do additional actions if necessary
# ...
break
fi
done
In this example, we have an array called my_array
with four elements. We want to check if the array contains the value "banana". The loop iterates through each element, comparing it to the desired value using the conditional if [ "$element" == "$value" ];
. If a match is found, a message is displayed using echo
.
Alternative Solution using Bash Extended Globbing ✨🔍
Another elegant method to perform the check is by using Bash's extended globbing feature. This approach involves transforming the array elements into a string and using pattern matching to determine if the desired value exists. Here's how you can do it:
#!/bin/bash
# Enable extended globbing
shopt -s extglob
# Declare an array
my_array=("apple" "banana" "cherry" "date")
# Value to check
value="banana"
# Convert array to string joined by '|'
array_string=$(IFS="|" ; echo "${my_array[*]}")
# Check if array string contains value using extended globbing
if [[ $array_string == *"$value"* ]]; then
echo "Array contains $value!"
# Do additional actions if necessary
# ...
else
echo "Array does not contain $value!"
fi
In this solution, we enable extended globbing with shopt -s extglob
. We then convert the array elements into a string joined by the |
separator using array_string=$(IFS="|" ; echo "${my_array[*]}")
. Finally, we check if the array string contains the desired value using pattern matching with [[ $array_string == *"$value"* ]]
.
Call-to-Action 📢📝🙌
Now that you know how to check if a Bash array contains a value, put your newfound knowledge into action and solve all your array-related challenges! Experiment with different arrays and values, and let me know in the comments section if you encounter any difficulties or have any other interesting Bash-related questions. I'm here to help! 🤓💬💪
Remember, understanding Bash arrays and their functionalities can greatly enhance your scripting skills. Stay curious and keep exploring the vast possibilities it offers. Happy scripting! 🎉👨💻💥