What is the Angular equivalent to an AngularJS $watch?
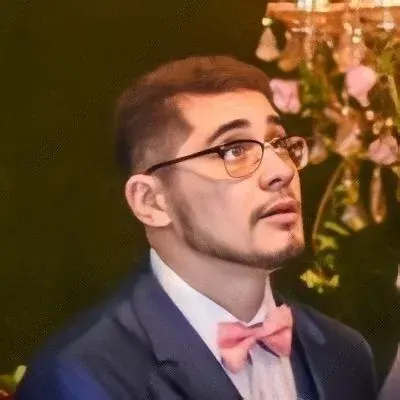
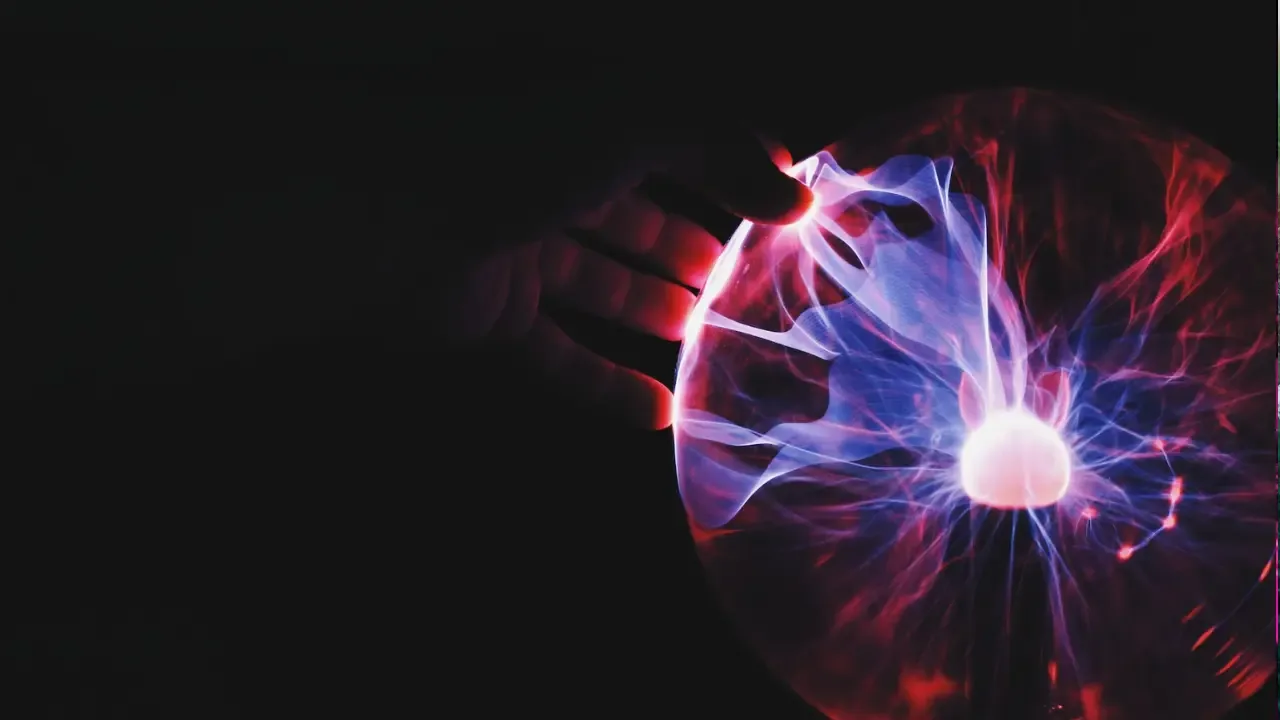
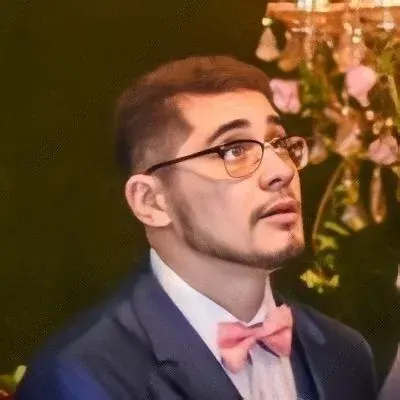
📝 Blog Post: The Angular Equivalent to an AngularJS $watch
Hey there, Angular enthusiasts! 👋
Are you an AngularJS developer venturing into the world of Angular? Wondering how to replicate the functionality of $watch
in Angular? Well, you've come to the right place! In this blog post, we'll delve into the Angular equivalent to an AngularJS $watch
and help you overcome any common issues you might encounter along the way. Let's get started! 🚀
The AngularJS $watch
in a Nutshell
In AngularJS, you could utilize the powerful $watch
function of the $scope
object to observe changes in scope variables. It allowed you to reactively track modifications to specific values and take actions accordingly. It was undoubtedly handy for implementing complex logic and updating the UI dynamically.
But, hold on! What about Angular? How do you achieve the same functionality in a more modern framework? 🤔
The Angular Solution: Property Binding and RxJS
In Angular, we bid adieu to the concept of $scope
and embrace component-based architecture. To achieve functionality similar to $watch
, we rely on two fundamental concepts: property binding and RxJS.
Property Binding
Angular's property binding is a simple yet powerful mechanism that allows you to bind component properties to template expressions. It establishes a connection between the component and its template, ensuring that changes in one are reflected in the other.
To mimic the behavior of $watch
, all you need to do is bind your component property to a variable and let Angular handle the rest. Here's an example:
import { Component } from '@angular/core';
@Component({
selector: 'app-my-component',
template: `
<h1>{{ myProperty }}</h1>
<button (click)="updateProperty()">Update Property</button>
`
})
export class MyComponent {
myProperty = 'Hello, Angular!';
updateProperty() {
this.myProperty = 'Hello, Angular 2+!';
}
}
In the example above, we've bound the myProperty
component property to the myProperty
template expression. Any changes made to myProperty
will automatically be reflected in the template. So when you click the "Update Property" button, Angular ensures the UI dynamically updates with the new value.
RxJS Observables
But what if we need to track changes in more complex scenarios, such as asynchronous operations or multiple variables? This is where the power of RxJS comes into play. Angular leverages RxJS observables to observe and react to changes, delivering a more robust and flexible solution compared to AngularJS $watch
.
By utilizing RxJS operators like map
, filter
, and debounceTime
, you can transform and filter the emitted values from observables, allowing you to respond to specific events precisely. Here's a taste of how it can be used:
import { Component } from '@angular/core';
import { Subject } from 'rxjs';
import { debounceTime, distinctUntilChanged } from 'rxjs/operators';
@Component({
selector: 'app-my-component',
template: `
<input type="text" [(ngModel)]="searchQuery" placeholder="Search" />
<div>{{ searchResults }}</div>
`
})
export class MyComponent {
searchQuery = '';
searchSubject = new Subject<string>();
searchResults = '';
constructor() {
this.searchSubject.pipe(
debounceTime(300),
distinctUntilChanged()
).subscribe((query: string) => {
this.getSearchResults(query);
});
}
onSearch() {
this.searchSubject.next(this.searchQuery);
}
getSearchResults(query: string) {
// Perform search and update searchResults
}
}
In this example, we use the ngModel
directive to bind the searchQuery
component property to an input field. Every time the user types, the searchSubject
emits the latest value after a debounce time of 300 milliseconds. This ensures the search is triggered only after the user finishes typing, improving performance. Finally, we subscribe to the observable, invoking the getSearchResults
method to perform the actual search operation.
📣 Your Turn: Engage and Share!
Now that you're equipped with the knowledge of Angular's equivalent to an AngularJS $watch
, it's time to put that knowledge to use! We encourage you to experiment, build amazing things, and share your experiences with the community.
Do you have any questions, feedback, or additional tips on this topic? We'd love to hear from you! Drop your thoughts in the comments below and let's start a conversation. Together, we can master Angular's powerful features! 💪
And don't forget to share this blog post with your fellow Angular developers who might find it helpful. Happy coding! 😄✨