Show spinner GIF during an $http request in AngularJS?
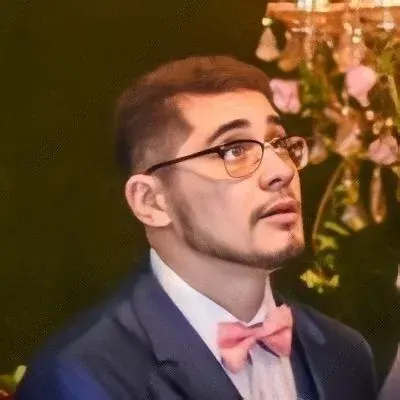
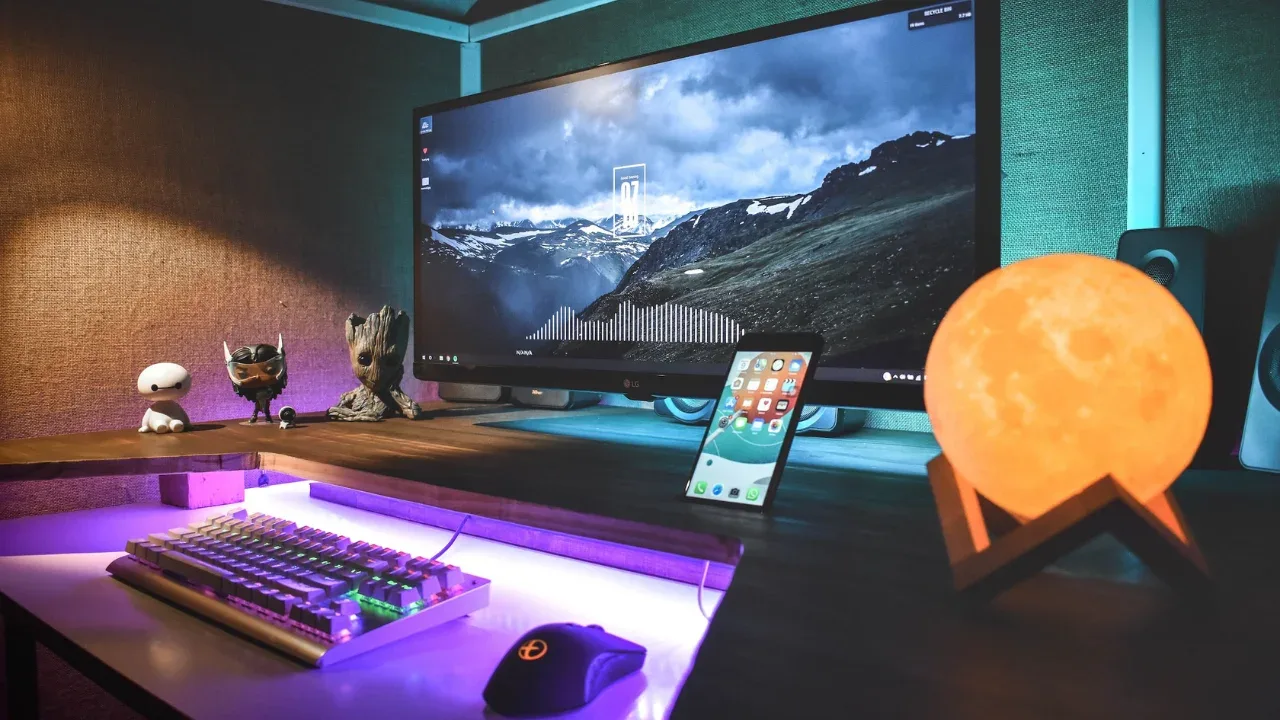
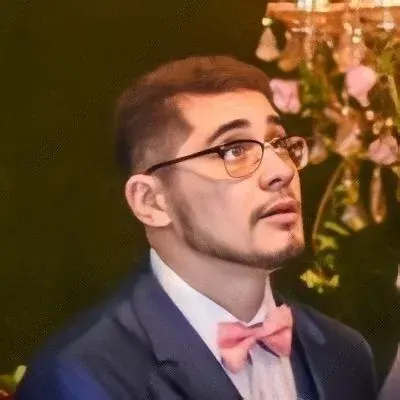
How to Show a Spinner GIF During an $http Request in AngularJS
So, you're using AngularJS and the $http
service to make an Ajax request, but you want to provide a better user experience by showing a spinner GIF or another type of busy indicator while the request is executing. 🌀
Unfortunately, there isn't an ajaxstartevent
like in traditional jQuery Ajax requests. But fear not! 😎 There are a few simple ways you can achieve this in AngularJS. Let's dive in!
Method 1: Using ng-if Directive and a Loading Flag
One way to show a spinner GIF during an $http
request is by using the ng-if
directive along with a loading flag. Here's how you can do it:
Step 1: Declare a Loading Flag in your Controller
In your controller, add a loading flag variable. This variable will be used to control the visibility of the spinner GIF.
$scope.loading = false;
Step 2: Update the Flag During the Request
When starting the Ajax request, set the loading flag to true
. Once the request completes, set it back to false
. You can do this using the .then()
function provided by $http
.
$scope.loading = true;
$http.get('/api/data')
.then(function(response) {
// Handle the response
})
.finally(function() {
$scope.loading = false;
});
Step 3: Show the Spinner GIF Using ng-if
In your HTML template, wrap the spinner GIF or your preferred busy indicator with an ng-if
directive, using the loading flag as the condition.
<div ng-if="loading">
<img src="spinner.gif" alt="Loading..." />
</div>
Method 2: Using ng-show Directive and CSS Styles
Another way to show a spinner GIF during an $http
request is by using the ng-show
directive and CSS styles. Here's how you can do it:
Step 1: Declare a Loading Flag in your Controller
Similar to Method 1, start by declaring a loading flag variable in your controller.
$scope.loading = false;
Step 2: Update the Flag During the Request
In this method, we'll use the .finally()
function provided by $http
to update the loading flag.
$scope.loading = true;
$http.get('/api/data')
.then(function(response) {
// Handle the response
})
.finally(function() {
$scope.loading = false;
});
Step 3: Set up CSS Styles
Create CSS styles to hide or display the spinner GIF based on the value of the loading flag.
.spinner {
display: none;
}
.spinner.show {
display: block;
}
Step 4: Use ng-show and CSS Classes
In your HTML template, add the ng-show
directive to the spinner GIF element, binding it to the loading flag. Also, apply the CSS classes to show or hide the spinner GIF.
<img src="spinner.gif" alt="Loading..." ng-show="loading" class="spinner show" />
Get Creative!
Feel free to get creative and customize these methods to suit your project's needs. You can change the spinner GIF, add transition effects, or even replace the spinner with a fancy animated loader icon. The possibilities are endless! 🎨
Remember, providing a loading indicator during Ajax requests can greatly improve the user experience, letting your users know that something is happening in the background.
That's it! You now have two simple methods to show a spinner GIF during an $http
request in AngularJS. Give them a try and let us know how it goes!
If you have any questions or other AngularJS topics you'd like us to cover, leave a comment below. Happy coding! 💻
Want to level up your AngularJS skills? Check out our AngularJS Masterclass and become an AngularJS guru!