Math functions in AngularJS bindings
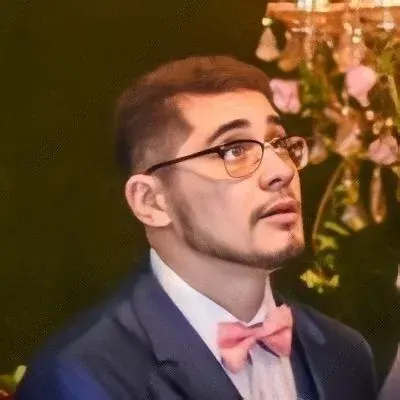
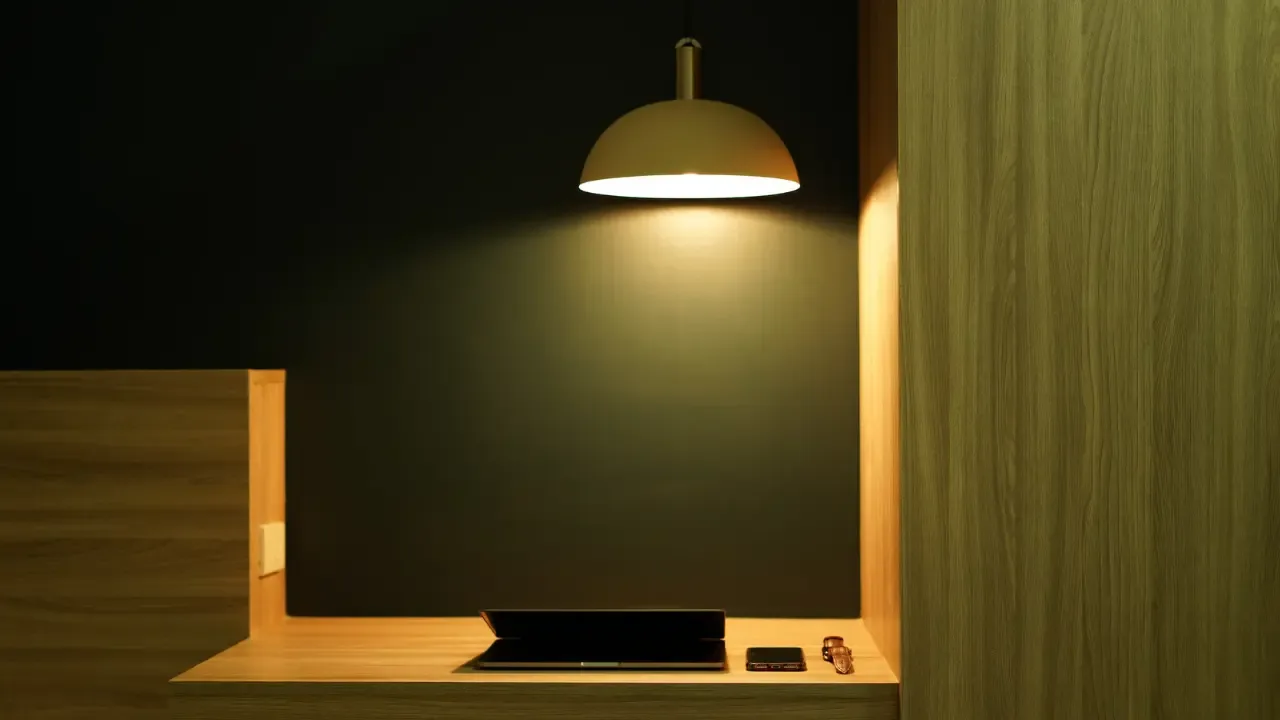
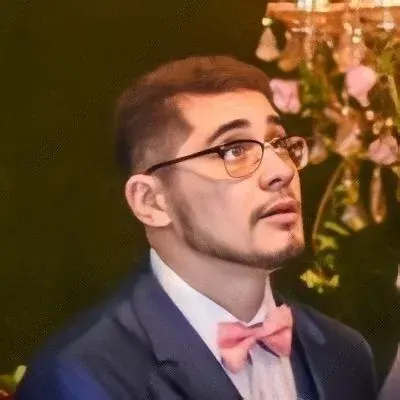
Math Functions in AngularJS Bindings: A Complete Guide
Are you struggling with using math functions in AngularJS bindings? Don't worry, we've got you covered! In this blog post, we'll address this common issue and provide easy solutions to help you out. So, let's dive in and solve this problem together! 💪
The Problem: Using Math Functions in AngularJS Bindings
The question at hand is whether it's possible to use math functions in AngularJS bindings. For example, let's say we want to display the percentage of a count out of a total using the Math.round()
function. We might try something like this:
<p>The percentage is {{Math.round(100*count/total)}}%</p>
However, when we try to implement this code, we run into issues. The result doesn't show up as expected, leaving us scratching our heads. 😕
Understanding the Issue: Expressions in AngularJS Bindings
To understand this problem, we need to dive into how AngularJS handles expressions in bindings. AngularJS uses a special syntax to evaluate expressions within double curly braces ({{ }}
) in HTML templates.
While basic arithmetic operations and variable references are supported, AngularJS doesn't allow the execution of JavaScript functions directly in the bindings. This is why we're facing issues when trying to use the Math.round()
function in our example.
The Solution: Creating a Custom Filter
To overcome this limitation, we can leverage AngularJS filters. Filters are a powerful way to transform data within AngularJS templates. We can create a custom filter that utilizes the Math.round()
function to achieve the desired outcome.
Let's see how we can create a custom filter to calculate the percentage using the Math.round()
function:
angular.module('yourApp', []).filter('percentage', function() {
return function(input, total) {
return Math.round((input / total) * 100);
};
});
In this example, we define a new filter called 'percentage'
. It takes an input
value (e.g., count
) and the total
value as inputs and returns the calculated percentage using the Math.round()
function.
Now, let's update our HTML code to utilize the custom filter:
<p>The percentage is {{ count | percentage:total }}%</p>
By applying the percentage
filter to the count
variable with total
as an argument, we can now display the correctly calculated percentage within our AngularJS bindings. 🎉
Try it out yourself!
To play around with this solution and see it in action, you can check out this live example on JSFiddle. Feel free to tweak the code and experiment with different values to see the results!
Conclusion
Using math functions in AngularJS bindings might seem like a roadblock, but with the help of custom filters, we can easily overcome this limitation and achieve the desired outcome. By creating a custom filter that incorporates the necessary math functions, we can transform our data and display it dynamically within our AngularJS templates.
So, the next time you find yourself facing this problem, remember the power of custom filters and how they can unlock new possibilities in your AngularJS applications. Happy coding! 😊🚀
If you found this guide helpful, don't forget to share it with your fellow developers! And if you have any questions or suggestions, feel free to leave a comment below. We love engaging with our readers! 👇💬