inline conditionals in angular.js
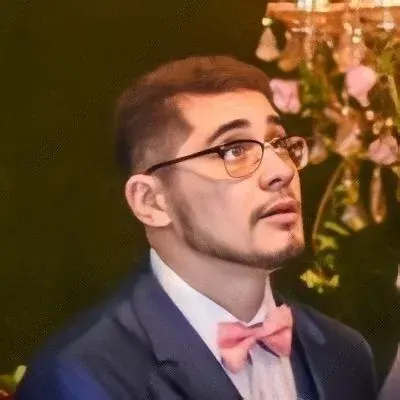
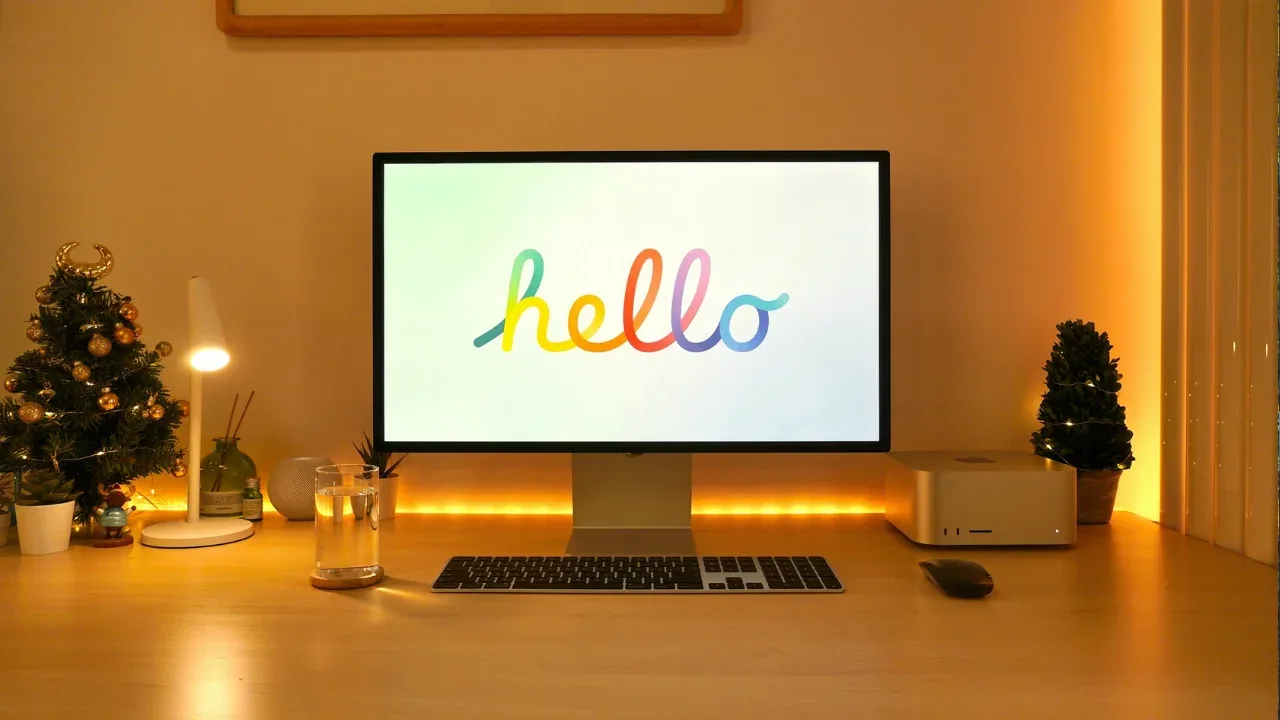
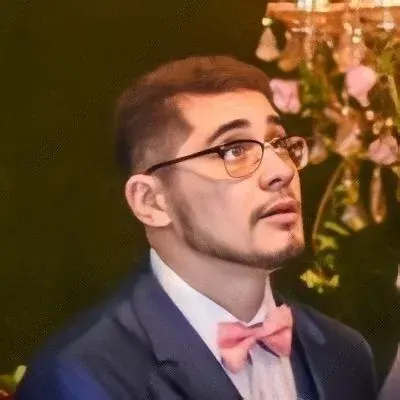
Easy and Clean Inline Conditionals in Angular.js 😎
Are you tired of the limitations of showing and hiding inline content in Angular.js? Do you wish there was a more flexible way to conditionally display content without having to wrap it in HTML tags? Well, you're in luck! In this blog post, we will explore how to achieve inline conditionals in Angular.js using the power of Angular's expressions.
Understanding the Problem 🤔
The problem at hand is that Angular.js, by design, encourages us to use directives like ng-show
and ng-hide
to conditionally display content. While these directives work perfectly fine, they require us to wrap the content in HTML tags, which might not always be the best solution. But fear not, there is a recommended way to achieve inline conditionals in Angular.js without resorting to complex workarounds.
The Solution 💡
To conditionally show and hide inline content in Angular.js using expressions, we can make use of the ng-if
directive along with curly braces {{}}
. Here's how it works:
{{ myVar === 'two' ? 'show this' : '' }}
In the above example, we are using the ternary operator (? :
) inside the curly braces. The expression myVar === 'two'
checks if the variable myVar
is equal to the string 'two'
. If it is, the expression evaluates to 'show this'
, and if it's not, it evaluates to an empty string.
This approach gives you the flexibility to conditionally display inline content without the need to wrap it in HTML tags. You can freely use this expression within any HTML element, as long as it's within the Angular.js scope.
Example Usage 🌟
Let's see the inline conditionals in action with a practical example. Suppose we have a variable isLoggedIn
that determines if a user is logged in or not. We can conditionally display a welcome message based on this variable:
<p>Welcome, {{ isLoggedIn ? 'User' : 'Guest' }}!</p>
In this example, if isLoggedIn
is true
, it will display "Welcome, User!". Otherwise, it will display "Welcome, Guest!".
Engage with Us! 📢
We hope this guide has helped you understand how to achieve inline conditionals in Angular.js easily and cleanly. If you have any questions or run into any issues, feel free to leave a comment below. We would love to hear from you and help you out!
Do you use inline conditionals in Angular.js in a different way? Share your approach in the comments section! Let's learn from each other and make our Angular.js code even better.
Until next time, happy coding! 👩💻👨💻