How to unsubscribe to a broadcast event in angularJS. How to remove function registered via $on
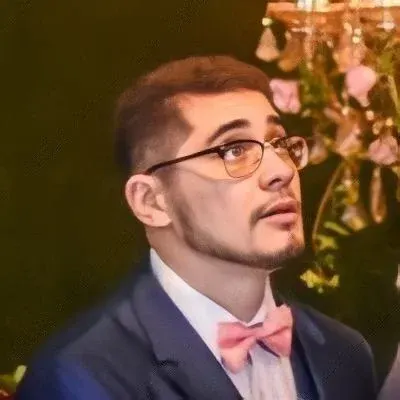
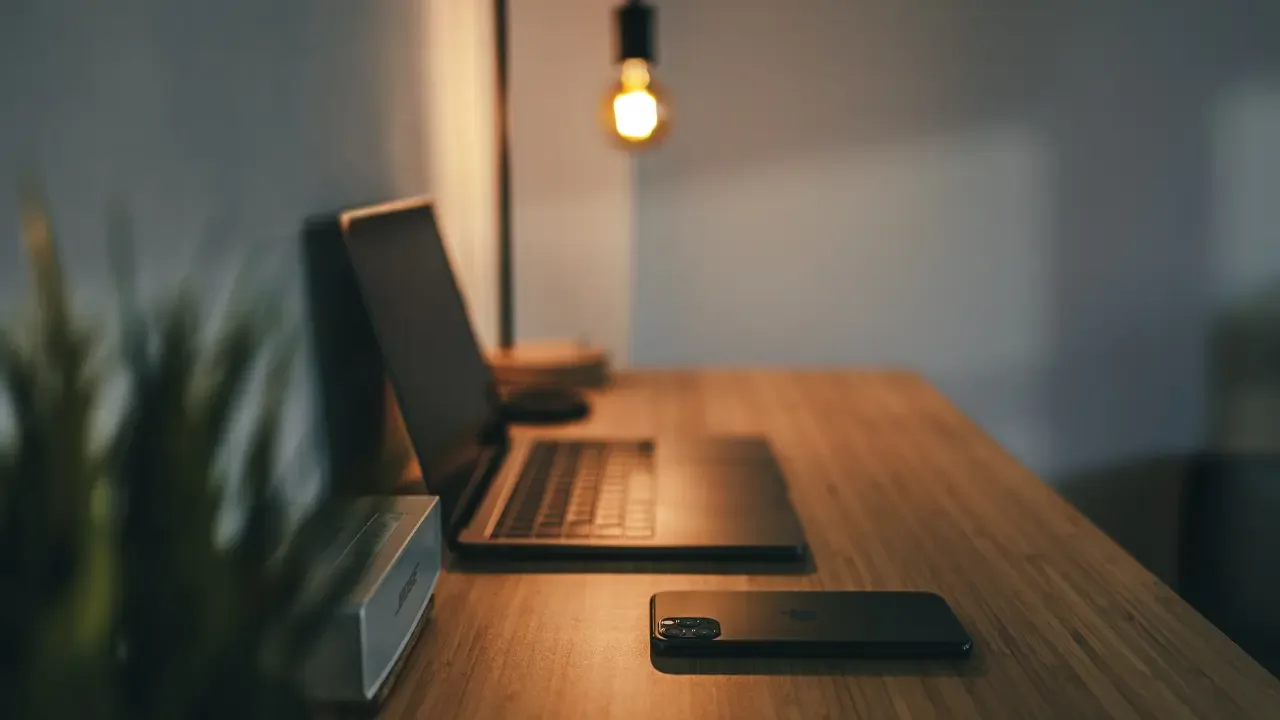
Unsubscribing to a Broadcast Event in AngularJS: The Ultimate Guide 👋🔌
So you've registered yourself to a $broadcast
event in AngularJS using the $on
function, but now you want to gracefully exit the event train. 🚂💔
Fear not, dear reader, as this blog post will guide you through the process of unsubscribing from a broadcast event and removing a registered function using the AngularJS magic. 🧙♂️✨
The $on Function
Before we dive into the unregistration process, let's briefly understand the $on
function. The $on
function is AngularJS's way of listening to custom events and broadcasting them throughout the application, ensuring proper communication between components. 📢👂
Here's an example of registering a listener to a $broadcast
event using $on
:
$scope.$on("onViewUpdated", this.callMe);
In this case, whenever the "onViewUpdated" event is broadcasted, the callMe
function will be invoked. 🎉
The Problem: Unregistering Listeners
Now, imagine a scenario where you need to unregister this listener based on a particular business rule. Here's the caveat: AngularJS doesn't provide a $off
method to neatly remove a specific listener function. 😱
But don't worry, we have a nifty workaround for you!
The Solution: Using $on's Return Value
To unregister a listener, you can utilize the return value of $on
, which is a deregistration function. 💡🔌
To demonstrate, check out the modified code snippet below:
var deregister = $scope.$on("onViewUpdated", this.callMe);
By assigning the return value of $on
to the deregister
variable, you now have the power to remove the listener at a later point in your code. 🕒✌️
To unregister the listener, simply invoke the deregister
function like this:
deregister();
Voila! 🎩 The listener function is now unregistered, and it will no longer be invoked when the "onViewUpdated" event is broadcasted. You have successfully hopped off the event train! 🚂❌
Unregistering from Another Function
But hold on, you might be wondering how you can unregister the listener from a different function, rather than the one where you registered it. Fear not, we have a solution for that too! 💪🙌
You can store the deregister
function in a shared scope or a globally accessible variable so that you can invoke it from any function. Here's an example:
var deregister; // Store the deregistration function
function registerListener() {
deregister = $scope.$on("onViewUpdated", this.callMe);
}
function unregisterListener() {
if (deregister) {
deregister(); // Invoke the deregistration function
}
}
Now, when you call the unregisterListener
function, it will gracefully unregister the listener, regardless of where you registered it.
Conclusion and Call-to-Action
Congratulations, my friend! You've learned how to unsubscribe from a broadcast event in AngularJS and remove a registered function. 🎉🔌
Remember, the secret sauce lies in capturing the return value of $on
and invoking it when you need to say goodbye to the event. If you want to unregister the listener from a different function, simply store the deregistration function in a shared variable.
Now it's time to put your newfound knowledge into action and unsubscribe to those events with confidence! Go ahead and give it a try in your AngularJS application. 💪📢
If you found this blog post helpful, please share it with your fellow AngularJS enthusiasts. Also, feel free to drop a comment below sharing your thoughts, questions, or any additional tips on working with broadcast events in AngularJS.
Happy coding! 😄👨💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
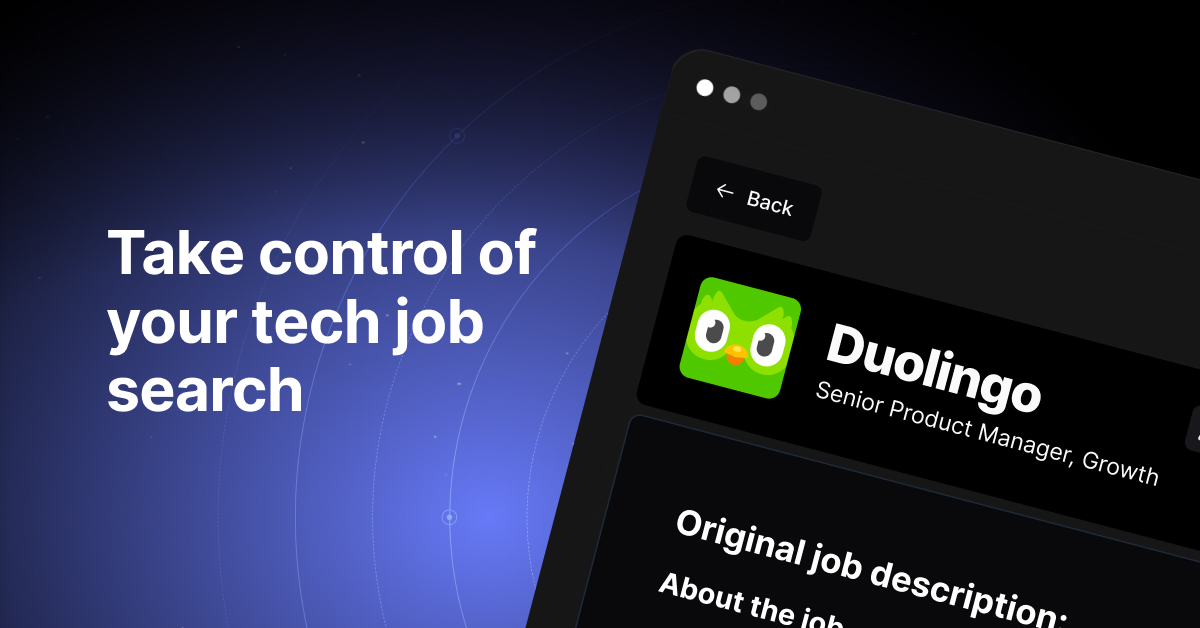