How to deep watch an array in angularjs?
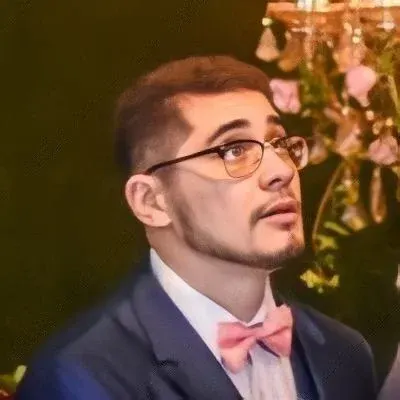
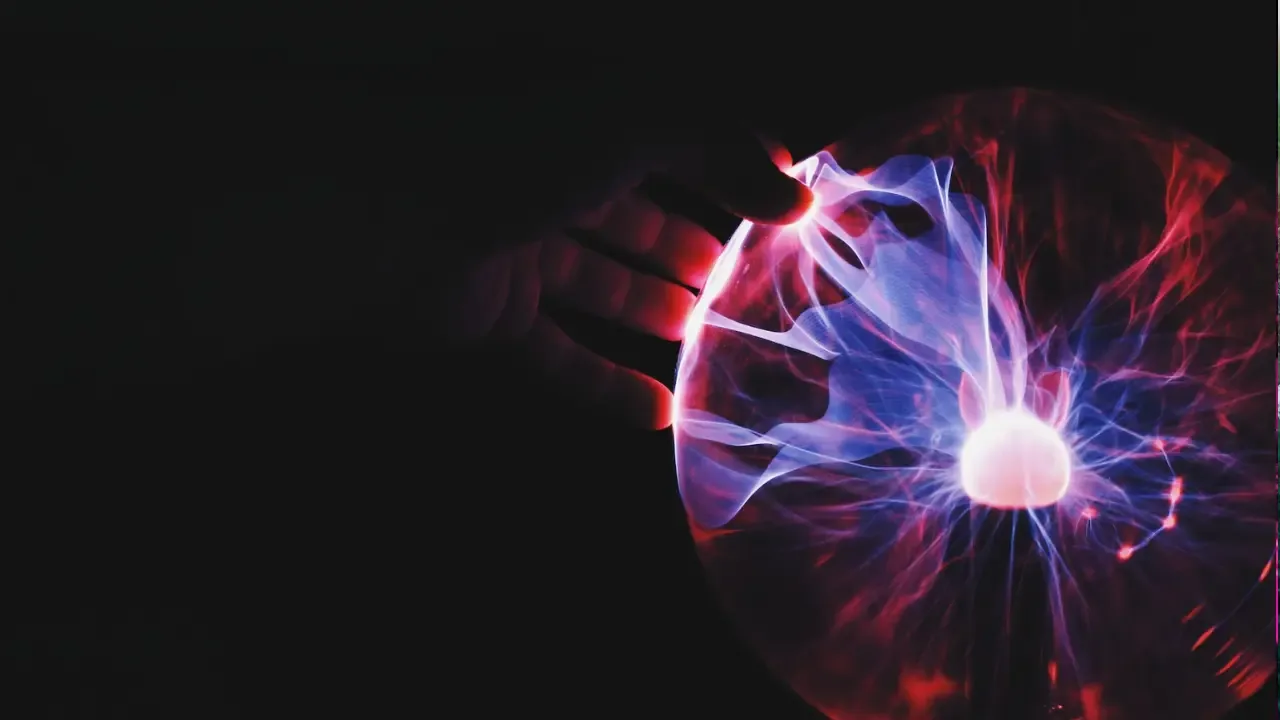
Deep Watching an Array in AngularJS: The Ultimate Guide
π Hey there, fellow AngularJS enthusiasts! Have you ever found yourself struggling with watching the values of objects inside an array using AngularJS? Have no fear, because I'm here to help you out! In this guide, we'll tackle this common issue and provide you with easy solutions to deep watch an array in AngularJS.
The Problem
The problem arises when you have an array of objects in your scope and you want to watch all the values of each object. Sounds simple, right? But when you try to watch the array, you realize that AngularJS's default $watch
function only checks if the reference to the array has changed. It doesn't go deep into the objects inside the array, thus failing to detect any changes in their values.
The Solution
To deep watch the objects inside an array, we need to leverage AngularJS's $watchCollection
function, which performs a deep comparison on the objects and their properties.
Here's how you can modify your code to successfully deep watch the columns
array:
function TodoCtrl($scope) {
$scope.columns = [
{ field:'title', displayName: 'TITLE'},
{ field: 'content', displayName: 'CONTENT' }
];
// Deep watch the columns array
$scope.$watchCollection('columns', function(newVal) {
alert('columns changed');
});
}
By using $watchCollection
instead of $watch
, AngularJS will detect changes not only in the array reference but also in the properties of the individual objects inside the array. Now, whenever you modify the values in the columns
array, the alert message will pop up, indicating that the columns have indeed changed.
Example
To provide you with a better understanding, let's take a look at an example that illustrates the concept.
$scope.products = [
{ name: 'Apple', price: 0.99 },
{ name: 'Banana', price: 0.5 },
{ name: 'Orange', price: 0.75 }
];
$scope.$watchCollection('products', function(newVal) {
console.log('products changed:', newVal);
});
In the above code snippet, we have an array of products
containing objects with name
and price
properties. By using $watchCollection
, we can keep track of changes in both the array and the properties of the objects inside it. Whenever a product's name or price changes, the callback function will be triggered, logging the updated products
array to the console.
Wrapping Up
Congratulations! You've successfully learned how to deep watch an array in AngularJS. By understanding the limitations of the default $watch
function and utilizing the power of $watchCollection
, you can now effortlessly keep track of changes in arrays of objects. π
Make sure to check out the live demo here to see deep watching in action. Feel free to experiment with different scenarios and see how AngularJS handles the changes.
Still have questions? Don't hesitate to leave a comment below or reach out to the AngularJS community for further assistance. And remember, happy coding! β¨
π’CALL TO ACTION: Share this guide with your fellow AngularJS developers who might be struggling with deep watching arrays. Let's spread the knowledge and make their lives easier too! πͺ
Let me know your thoughts, and if you found this guide helpful, share it on social media and tag your developer friends. Together, we can conquer AngularJS challenges! π
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
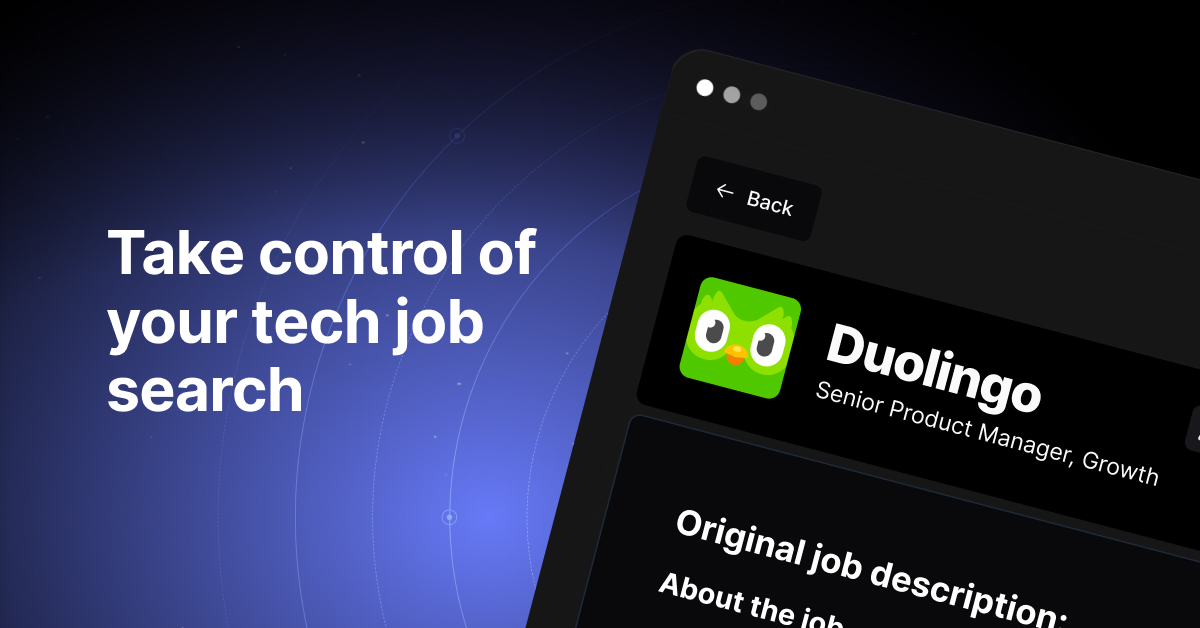