How to add custom validation to an AngularJS form?
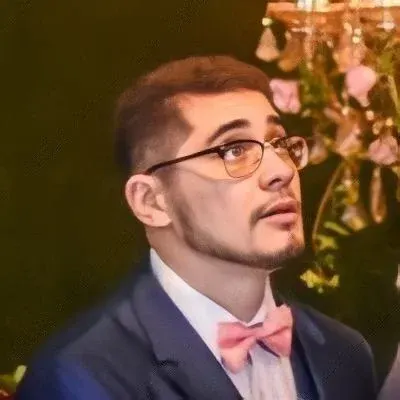
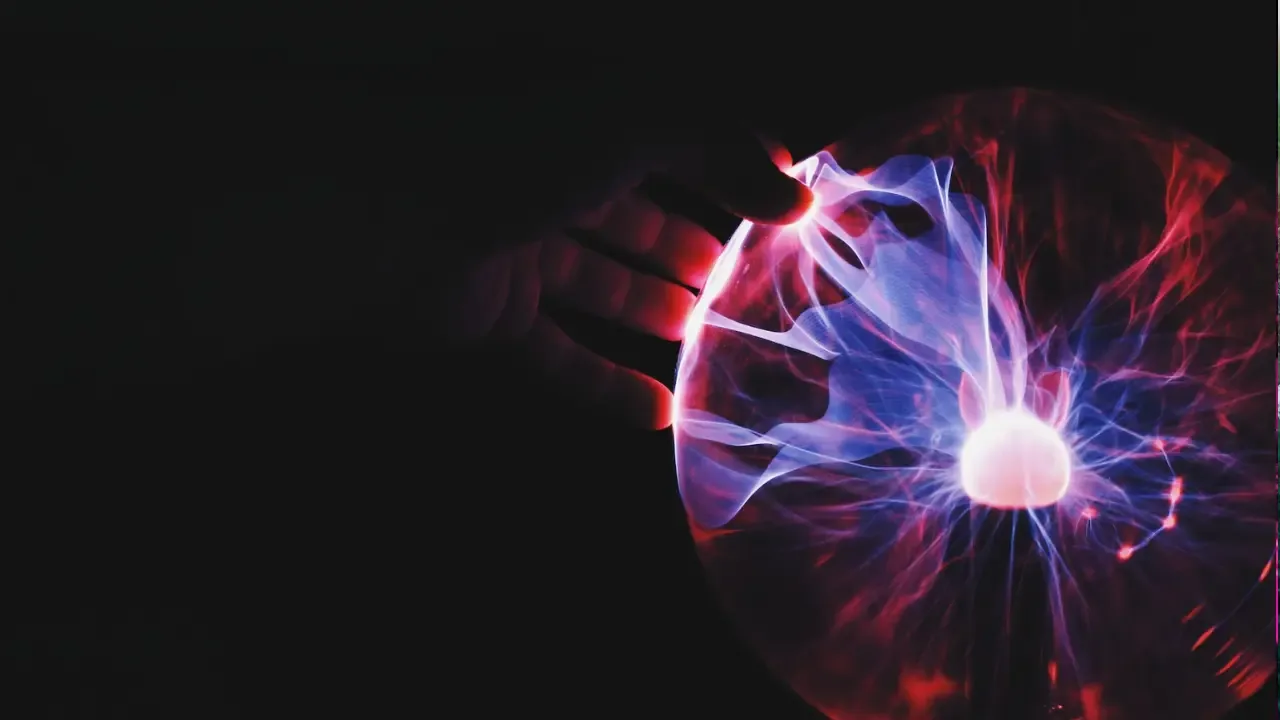
How to Add Custom Validation to an AngularJS Form 💪🔧💻
So, you've set up a form in AngularJS with validation using the required
attribute. But now you want to take it a step further and add some custom validation to certain fields. Fear not! In this guide, we'll walk you through some easy solutions to accomplish just that. Let's get started! 🚀✨
Understanding the Challenge 🤔❓
In the provided context, the user asks how to tap into the validation controls provided by the FormController
in AngularJS. They want to create custom validation rules for specific scenarios without creating separate directives for each rule. Additionally, they are interested in marking fields as invalid from the controller while keeping the FormController
in sync. These are valid concerns, and we've got you covered! 😉
Solution 1: Creating Custom Directives Using NgModelController
✅🔍📝
One approach that allows you to add custom validation without creating separate directives for each rule is by utilizing NgModelController
. By creating a custom directive, you can tap into the power of NgModelController
and define your specific validation logic.
Here's an example:
<input type="text" ng-model="myField" my-custom-validation>
In the above code snippet, we've added a directive called my-custom-validation
to the input field. Let's see how to define this directive:
app.directive('myCustomValidation', function() {
return {
require: 'ngModel',
link: function(scope, element, attrs, ngModelCtrl) {
ngModelCtrl.$parsers.unshift(function(viewValue) {
// Add your custom validation logic here
if (viewValue === 'yourCustomValue') {
ngModelCtrl.$setValidity('customValidation', true);
} else {
ngModelCtrl.$setValidity('customValidation', false);
}
return viewValue;
});
}
};
});
In this example, we're using the link
function of the directive to access the ngModelCtrl
and add custom validation logic. The customValidation
is the name we use to set the validity of the field.
Solution 2: Marking a Field as Invalid from the Controller ✅🚫🖐️
If your scenario requires marking a field as invalid from the controller while keeping the FormController
in sync, you can achieve that by utilizing the $setValidity
method.
Here's an example:
app.controller('MyController', function($scope) {
$scope.markFieldAsInvalid = function() {
$scope.myForm.myField.$setValidity('customValidation', false);
};
});
In this example, we have a controller called MyController
, and when the markFieldAsInvalid
function is called, it marks the field myField
as invalid.
Conclusion and Call to Action 🎉📢💬
Adding custom validation to your AngularJS forms is easier than you might think! By creating custom directives using NgModelController
or marking fields as invalid from the controller, you can tailor your validation to meet your specific needs.
Now that you have the knowledge, it's your turn to apply it to your own projects. Don't forget to share your thoughts and experiences in the comments below! 💬👇
Happy coding! 😄💻🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
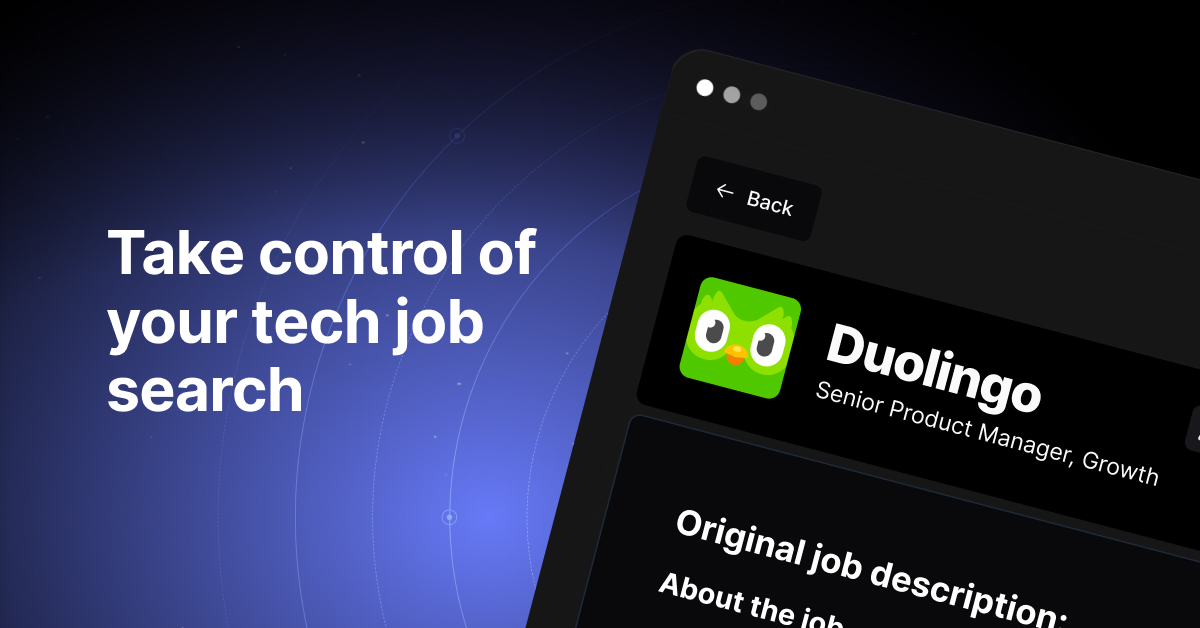