How to access parent scope from within a custom directive *with own scope* in AngularJS?
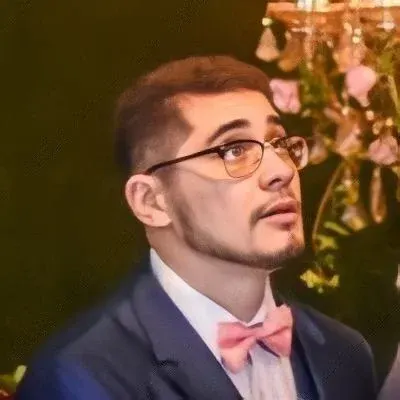
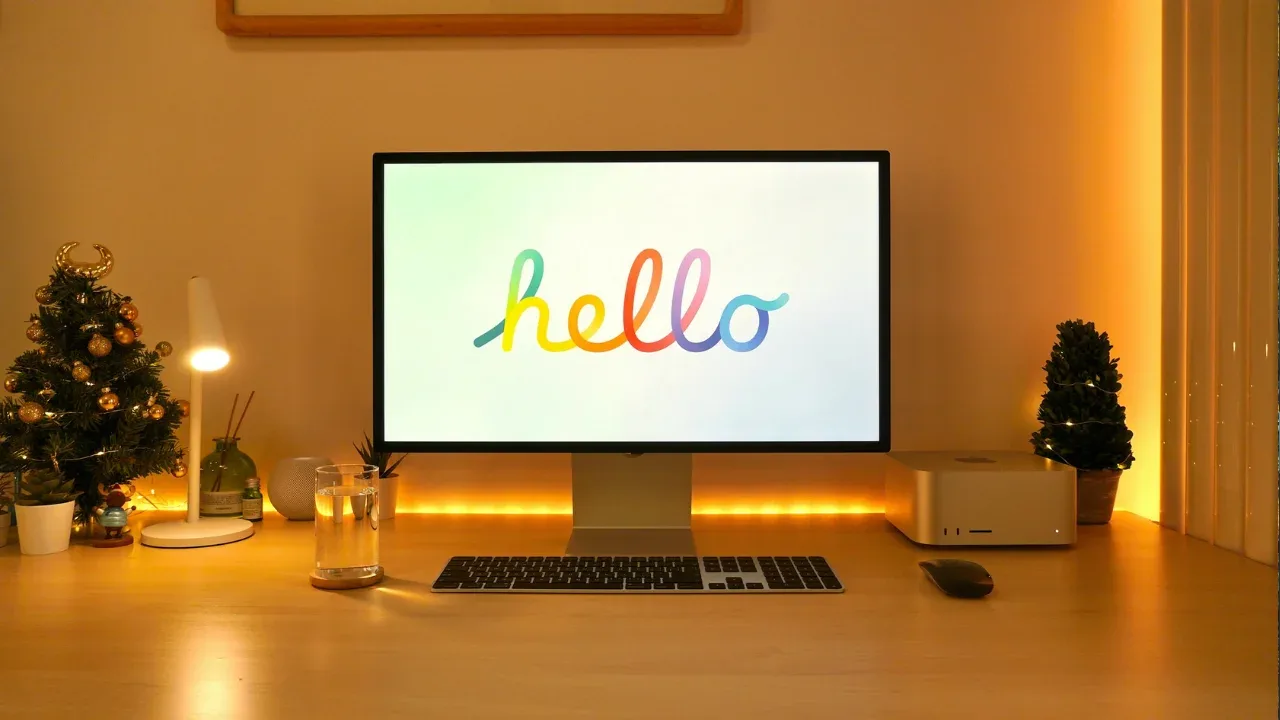
šTitle: How to Access Parent Scope from within a Custom Directive with Own Scope in AngularJS
š Hey there, AngularJS enthusiasts! š Are you struggling to access the parent scope from within a custom directive with its own scope? Don't worry, you're not alone! Many developers have faced this challenge and asked this question. š¤
The good news is that there are simple and maintainable solutions to help you achieve this without resorting to hacky techniques. In this blog post, I'll guide you through the process step-by-step, providing easy-to-follow examples and explanations. Let's dive right in!
Understanding the Context
Before we jump into the solutions, let's understand the context of the question. The developer is seeking a way to access the parent scope within a directive. They want to avoid any unmaintainable or hacky solutions while achieving their goal of watching an expression in the parent scope. The directive needs to be reusable within the same parent scope, and each instance of the directive should have its own scope.
Solution Approach
To address this problem, we need to define our directive in a way that allows us to access the parent scope while maintaining reusability. Let's look at the code sample provided to better understand the approach:
app.directive('watchingMyParentScope', function() {
return {
require: /* ? */,
scope: /* ? */,
transclude: /* ? */,
controller: /* ? */,
compile: function(el,attr,trans) {
// Implementation details
}
};
});
Solution Steps
Here are the steps we'll follow to solve this problem:
Set
scope: true
in the directive's definition. This ensures that a new child scope is created for each instance of the directive.Use the
require
option to access the parent controller. This allows us to interact with the parent scope from within the directive.Implement the directive's logic within the
link
function, as it provides access to both the directive's scope and the parent controller's scope.Utilize the
$watch
function to monitor changes in the parent scope's variable. This enables us to accomplish our objective effectively.
Now, let's apply these steps to the code sample provided:
app.directive('watchingMyParentScope', function() {
return {
require: '^parentController',
scope: true,
link: function(scope, element, attrs, parentCtrl) {
scope.$watch('parentCtrl.parentVariable', function(newVal, oldVal) {
// React to changes in the parent scope's variable
});
}
};
});
In this example, we have modified the directive to incorporate the previously mentioned steps to access the parent scope. The directive now creates its own child scope (scope: true
) and uses the require
option to request access to the parent controller using the ^
prefix.
Once we have access to the parent controller (parentCtrl
), we can utilize the $watch
function to observe changes in the parentVariable
. Feel free to modify the logic within the $watch
callback to accomplish your specific requirements.
That's it! By following these steps, you can successfully access the parent scope from within your custom directive with its own scope.
š„Call-to-Action: Congratulations, you've learned how to effectively access the parent scope within a custom directive with its own scope in AngularJS! š Apply this knowledge to enhance your projects and streamline your development process. Should you have any questions or want to share your experiences, leave a comment below. Let's engage in a discussion! š
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
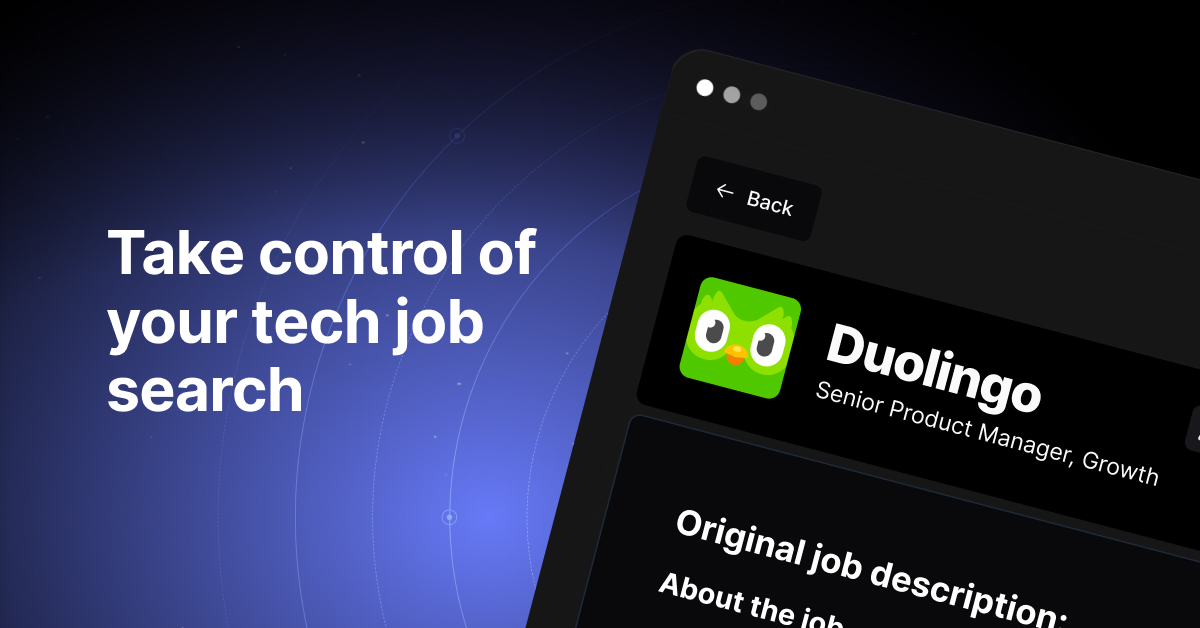