How do I use $rootScope in Angular to store variables?
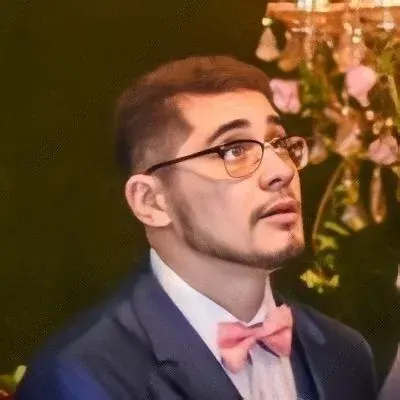
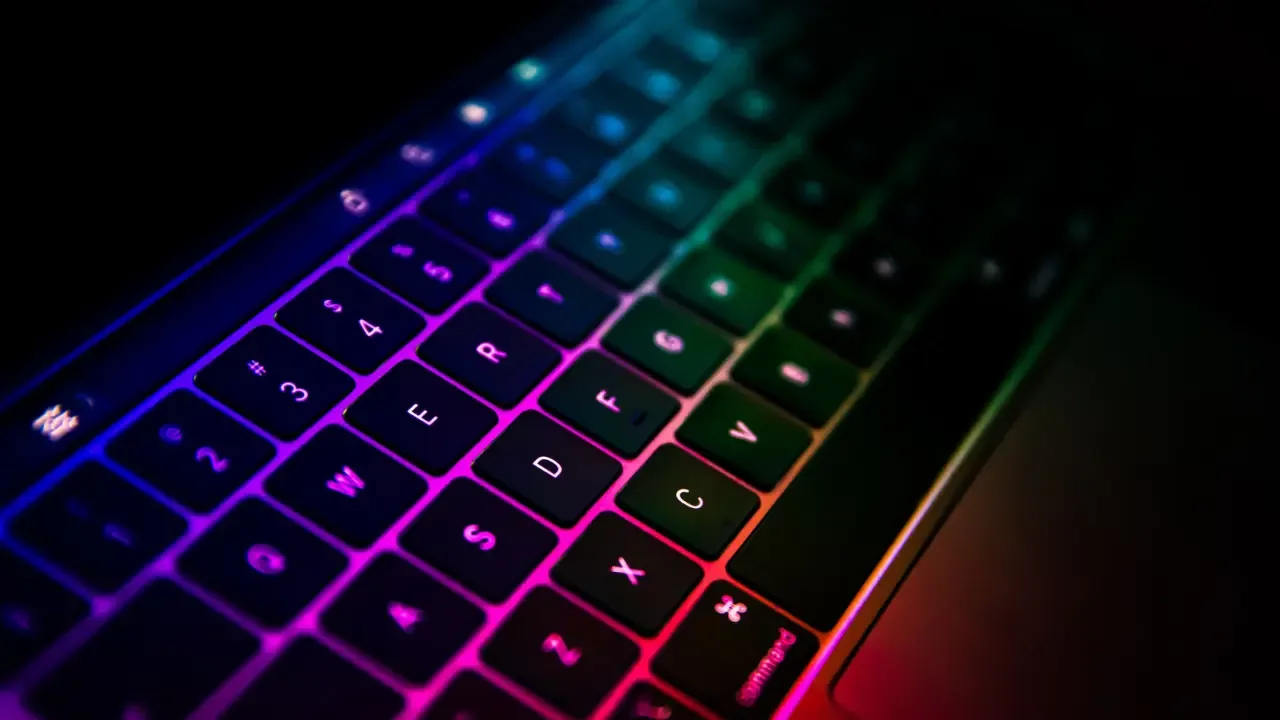
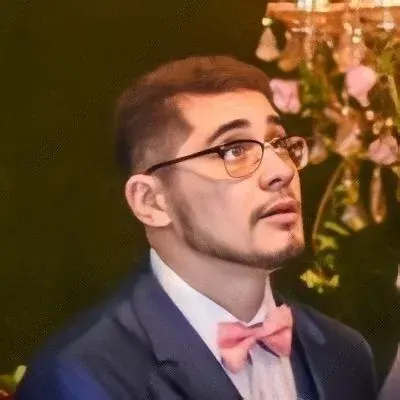
š Blog Post
š Hey there, Angular enthusiasts! Are you struggling with using $rootScope
in Angular to store variables and access them in different controllers? š¤ Don't you worry, we've got you covered! In this guide, we'll explore this common issue and provide you with easy solutions.
š Let's take a look at the context around the question. A developer reached out and asked, "How do I use $rootScope
to store variables in a controller that I want to later access in another controller?" They provided an example code snippet:
angular.module('myApp').controller('myCtrl', function($scope) {
var a = //something in the scope
//put it in the root scope
});
angular.module('myApp').controller('myCtrl2', function($scope) {
var b = //get var a from root scope somehow
//use var b
});
š¤ The questioner wanted to store the value of variable a
in $rootScope
within the myCtrl
controller and then access the stored value in the myCtrl2
controller. So, how can we make this work?
š§ Solution: We need to utilize Angular's $rootScope
to achieve variable storage across different controllers. Here's how you can do it:
1ļøā£ Inject $rootScope
into both controllers:
angular.module('myApp').controller('myCtrl', function($scope, $rootScope) {
// ...
});
angular.module('myApp').controller('myCtrl2', function($scope, $rootScope) {
// ...
});
2ļøā£ Assign the value of a
to $rootScope
:
angular.module('myApp').controller('myCtrl', function($scope, $rootScope) {
var a = // something in the scope
$rootScope.a = a; // assign to $rootScope
});
3ļøā£ Access and use the value of a
from $rootScope
in myCtrl2
:
angular.module('myApp').controller('myCtrl2', function($scope, $rootScope) {
var b = $rootScope.a; // get var a from $rootScope
// use var b
});
š That's it! You can now store the value of a
in $rootScope
and then access it in the myCtrl2
controller using $rootScope.a
.
š” Keep in mind that using $rootScope
to store variables should be done judiciously as it can lead to potential code complexity and scope pollution. Consider alternative approaches like using services to share data between controllers.
š£ Call-to-Action: Now that you've learned how to use $rootScope
to store variables in Angular, why not share this knowledge with your fellow developers? Click that share button below and spread the word. Also, don't hesitate to drop your questions or feedback in the comments section. Happy coding! šāØ