How can I dynamically add a directive in AngularJS?
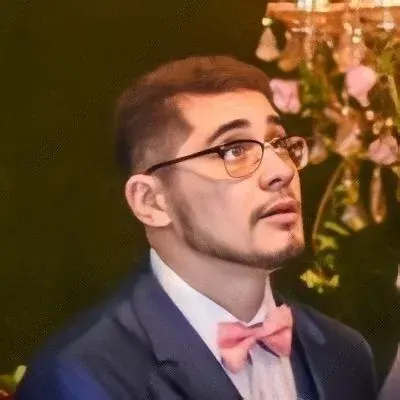
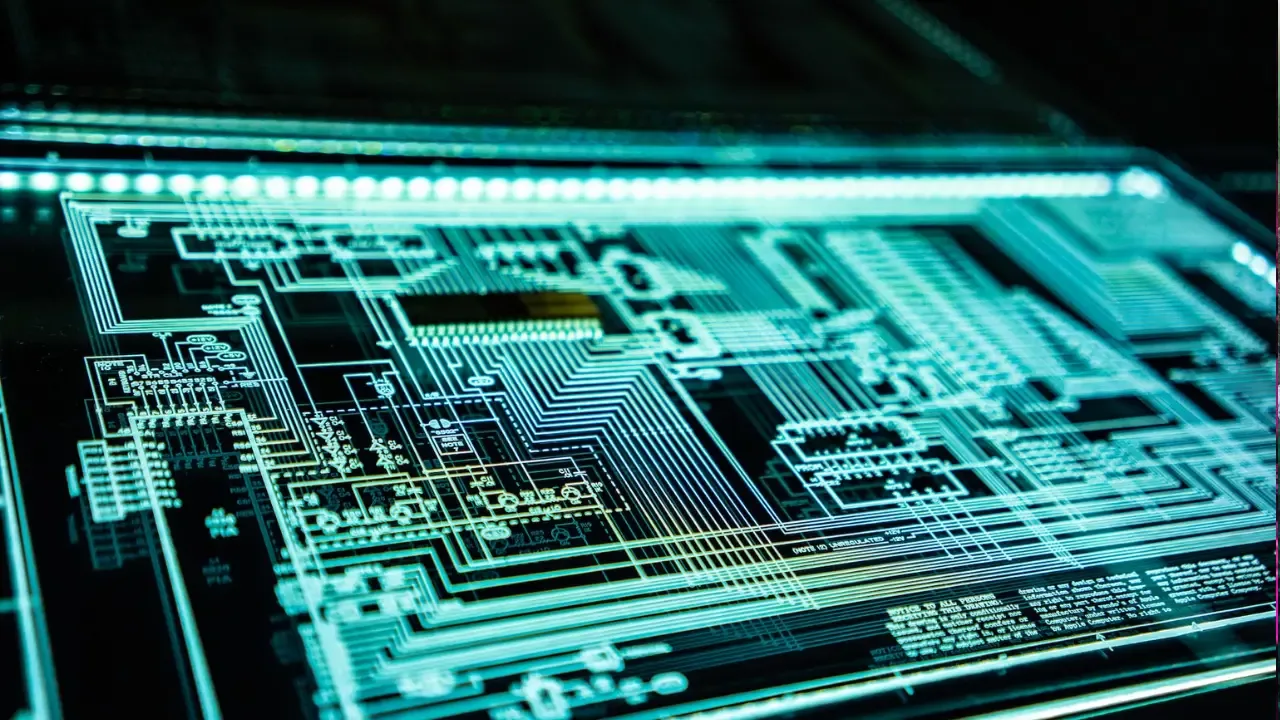
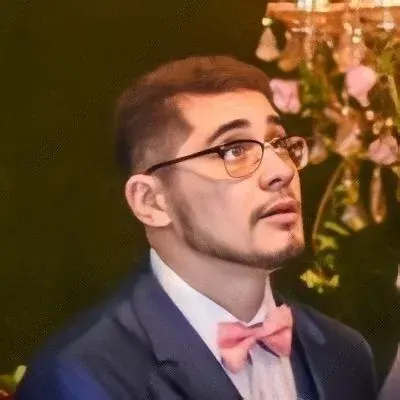
π‘ Wondering how to dynamically add a directive in AngularJS? You've come to the right place! π Here's a quick guide to help you tackle this common issue and find an easy solution. Let's dive in! π
π Understanding the Problem
You have a straightforward directive that adds another element whenever you click on it. However, you noticed that it needs to be compiled first for correct rendering. You've done some research and found that the $compile
service might be the way to go. But, the examples you've found seem a bit too complicated for your specific scenario.
ποΈββοΈ The Solution
Luckily, Josh David Miller has come to the rescue with a simplified solution! π¦ΈββοΈ Let's take a look at the modified code in the provided JSFiddle: http://jsfiddle.net/paulocoelho/fBjbP/2/
var module = angular.module('testApp', [])
.directive('test', ['$compile', function ($compile) {
return {
restrict: 'E',
template: '<p>{{text}}</p>',
scope: {
text: '@text'
},
link: function (scope, element) {
$(element).click(function () {
var compiledDirective = $compile("<test text='n'></test>")(scope);
$(this).parent().append(compiledDirective);
});
}
};
}]);
π§ Explanation
Josh's solution is pretty neat, but let's break it down to understand what's going on:
He added
'$compile'
as a dependency to the directive function so that he can use the$compile
service later.Inside the
link
function, the click event is bound to the directive's element using$(element).click(...)
.When the element is clicked, a new compiled version of the directive is created by calling
$compile("<test text='n'></test>")(scope)
. This ensures that the dynamically added directive is compiled and ready to be inserted into the DOM.Finally, the newly compiled directive is appended to the parent element using
$(this).parent().append(compiledDirective)
.
And voila! You can now dynamically add your directive without any rendering issues. π
π Take Action
Now that you have the solution at your fingertips, it's time to give it a try! Head over to the provided JSFiddle http://jsfiddle.net/paulocoelho/fBjbP/2/ and play around with it. Experiment, have fun, and see how it works for your specific case.
π€ Engage with the Community
Have any questions or comments? Found a different approach? We'd love to hear from you! Join our vibrant community of AngularJS enthusiasts by leaving a comment on this blog post or sharing your thoughts on social media using the hashtag #DynamicDirectivesMadeEasy. Let's learn and grow together! π
That's it, folks! We hope this guide helps you dynamically add directives in AngularJS with ease. Until next time, happy coding! π»β¨