Global variables in AngularJS
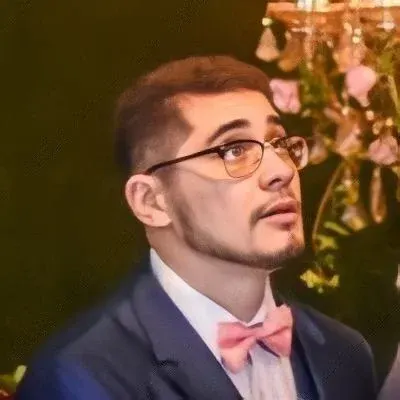
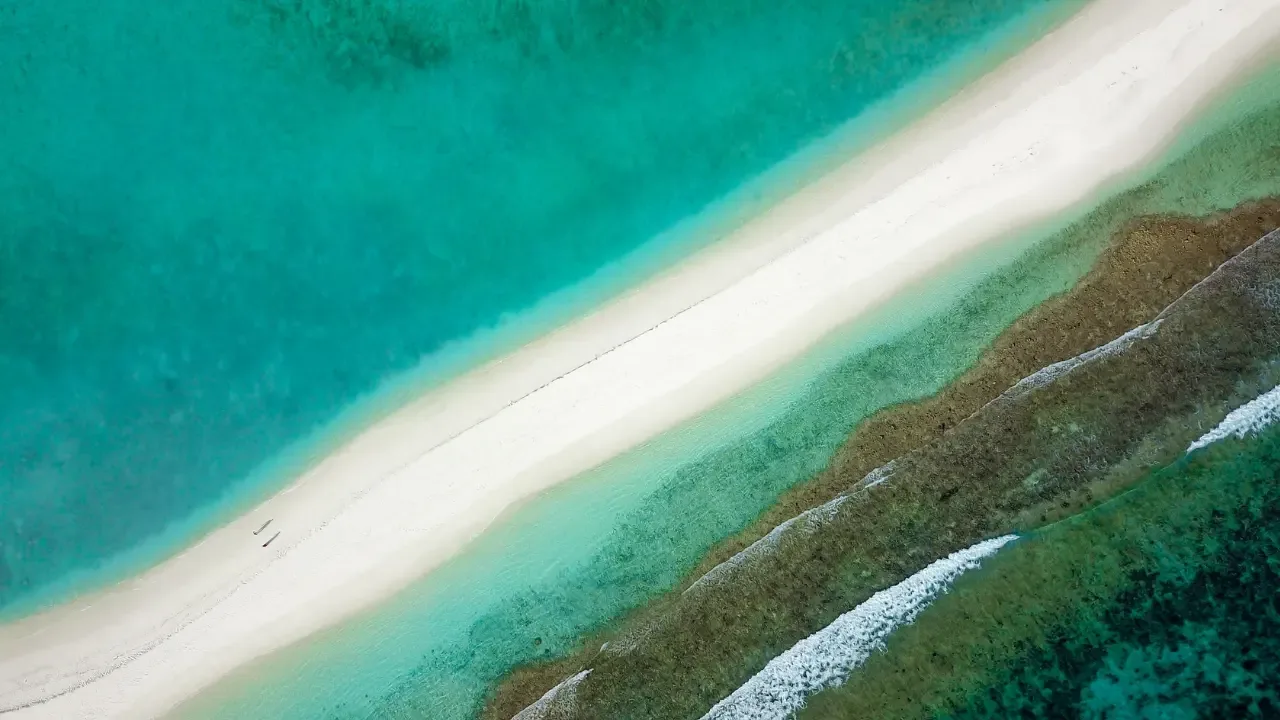
The Ultimate Guide to Global Variables in AngularJS 🌍💡
Have you ever encountered a situation where you initialized a variable in one controller, but then it unexpectedly gets reset by AngularJS? 🔄😕 In this blog post, we will explore the concept of global variables in AngularJS, common issues that arise, and provide easy solutions to maintain their values across controllers. Let's dive in! 💪🚀
Understanding the Problem 🤔🔍
The problem described by our fellow developer is quite common. They have initialized a variable on the $scope in one controller, but it gets overridden or reset by another controller. This variable plays a crucial role in managing various aspects of the site, such as the navigation bar and user access restrictions. Thus, it is crucial for it to retain its value. 😫❌
Why Global Variables are Not the Answer ❌🌐
Before we dive into the correct approach, let's understand why using global variables isn't recommended in AngularJS. AngularJS encourages modular, reusable, and maintainable code. By using global variables, you introduce unnecessary dependencies and tightly couple your code, making it difficult to test and maintain. Additionally, global variables can lead to unexpected side effects and make debugging a nightmare. 🙅♀️🔀🔖
The Correct Approach: Services and $rootScope ✅🏗️
To address this issue, AngularJS provides two powerful solutions: services and $rootScope.
1. Services 🛠️💼
Services in AngularJS are singletons that can be injected into different controllers. They provide a clean way to share data and functionalities across multiple controllers while ensuring modularity and separation of concerns. By creating a service to hold your global variable, you can easily share its value and maintain consistency across controllers. Here's a brief example:
angular.module('myApp').service('GlobalVariableService', function() {
var globalVariable = '';
return {
getGlobalVariable: function() {
return globalVariable;
},
setGlobalVariable: function(value) {
globalVariable = value;
}
};
});
In the above code snippet, we define a service called "GlobalVariableService" that holds our global variable. The service provides getter and setter methods to get and set the value.
Now, in any controller where we want to access or modify the global variable, we just need to inject the "GlobalVariableService" and use its methods:
angular.module('myApp').controller('Controller1', function($scope, GlobalVariableService) {
$scope.globalVariable = GlobalVariableService.getGlobalVariable();
// Update the global variable
GlobalVariableService.setGlobalVariable('new value');
});
By utilizing services, we ensure that the global variable maintains its value across different controllers.
2. $rootScope 🌳💁♂️
Another approach is to leverage the $rootScope, which is the top-most scope accessible in an AngularJS application. Anything attached to $rootScope becomes accessible throughout the application. However, using $rootScope excessively can lead to performance issues and code readability problems. Hence, it should be used sparingly and judiciously.
To set a global variable using $rootScope:
angular.module('myApp').controller('Controller1', function($rootScope) {
$rootScope.globalVariable = 'initial value';
// Update the global variable
$rootScope.globalVariable = 'new value';
});
To access the global variable in another controller:
angular.module('myApp').controller('Controller2', function($rootScope) {
var globalValue = $rootScope.globalVariable;
});
While $rootScope can be handy for simple scenarios, using services is generally considered a better practice for maintaining global variables.
Call-to-Action: The Power is in Your Hands! 🙌💪
Now that you have learned the correct approach to handling global variables in AngularJS, it's time to put it into practice! Start by refactoring your controllers and using services or $rootScope to ensure your global variables retain their values.
Let us know your experience in the comments below! 🔽✍️ If you have any questions or encounter any issues, our community is here to help you out! 🤝🌟
Happy coding! 💻❤️🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
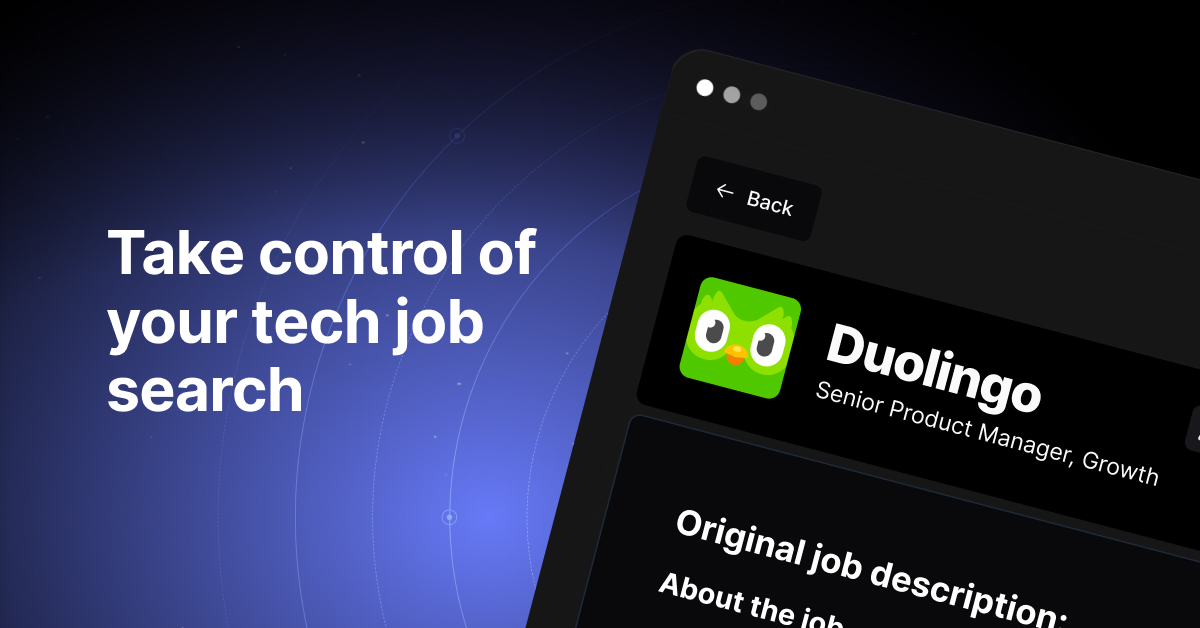