File Upload using AngularJS
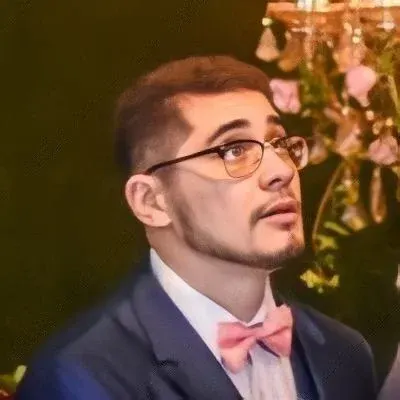
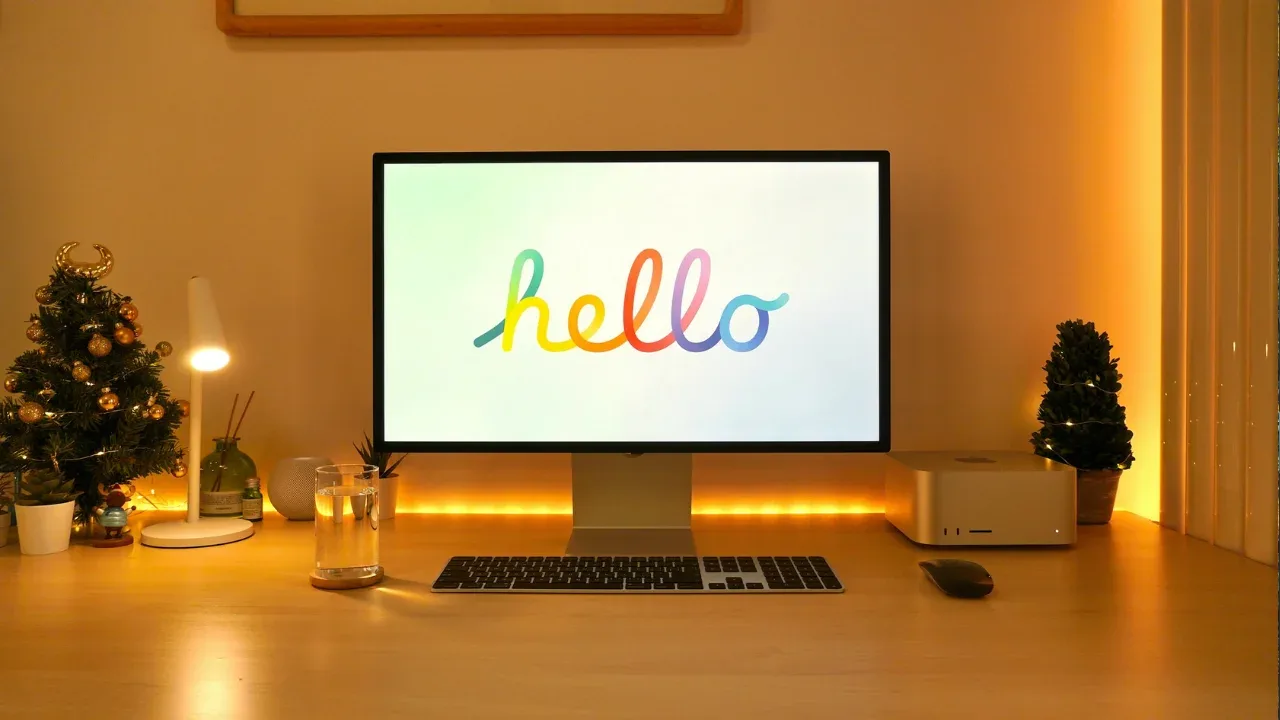
📁 File Upload using AngularJS: Easy Solutions and Common Issues
So, you want to upload a file from your local machine and read its content using AngularJS, eh? 📤📥 Don't worry, I’ve got your back! Let's dive into this common issue and find easy solutions! 💪
First, let's take a look at your HTML form: 👀
<form name="myForm" ng-submit="">
<input ng-model='file' type="file"/>
<input type="submit" value='Submit'/>
</form>
You mentioned that when you try to print the value of $scope.file
, it comes out as undefined
. Hmm, seems like we need to handle a few things here. 😬
1. Understanding the Problem
In AngularJS, the ng-model
directive is used to bind input elements to variables in the scope. However, ng-model
doesn't handle file uploads out of the box. 🙅♂️
2. The Solution: Using AngularJS directives and FileReader API
To get the file content, we need to use the FileReader API. This API allows us to read the contents of a file before we can use it in AngularJS.
Here's an updated version of your HTML form:
<form name="myForm" ng-submit="uploadFile()">
<input type="file" file-model="file" />
<input type="submit" value="Submit"/>
</form>
You might notice the addition of the file-model
attribute. This custom directive will help us bind the file to our model variable.
3. Implementing the file-model Directive
Now, let's add the custom directive to our AngularJS module:
app.directive('fileModel', ['$parse', function ($parse) {
return {
restrict: 'A',
link: function (scope, element, attrs) {
let model = $parse(attrs.fileModel);
let modelSetter = model.assign;
element.bind('change', function () {
scope.$apply(function () {
modelSetter(scope, element[0].files[0]);
});
});
}
};
}]);
This custom directive enables us to capture the file selected by the user and bind it to the file
model variable.
4. Reading the File Content
Finally, let's update our controller to read the content of the uploaded file:
app.controller('myController', ['$scope', function($scope) {
$scope.uploadFile = function() {
let reader = new FileReader();
reader.onload = function(e) {
$scope.$apply(function(){
$scope.fileContent = e.target.result;
});
};
// Read the content as a data URL
reader.readAsDataURL($scope.file);
};
}]);
🎉 And voilà! Now, when you submit the form, the content of the uploaded file will be available in the $scope.fileContent
variable.
📣 Call-to-Action: Share Your Experience!
I hope this guide helped you solve your file upload issue with AngularJS! If you found it useful, don't hesitate to share this post with your fellow developers. Let's spread the knowledge! 🌟
Also, if you have any questions or faced any issues while implementing the solution, drop a comment below. I'd love to hear your experience and help you out! 🤝💬
Happy coding! ✨🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
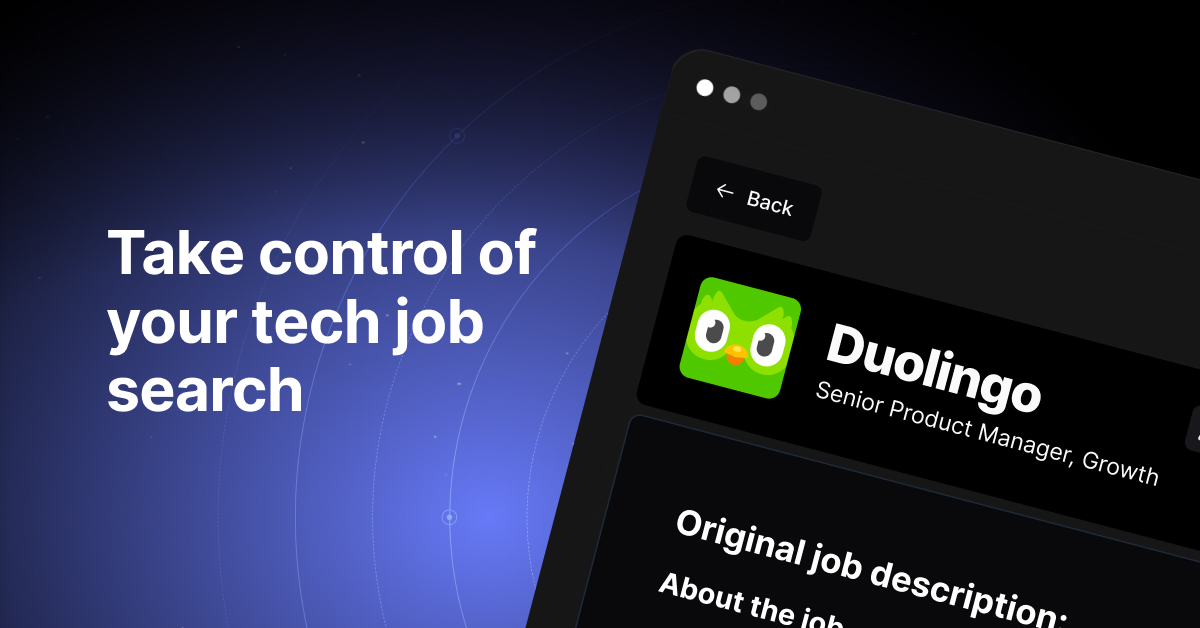