Can I inject a service into a directive in AngularJS?
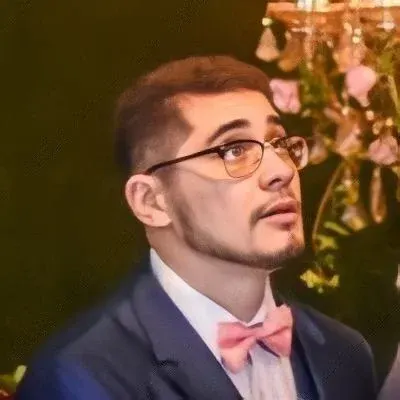
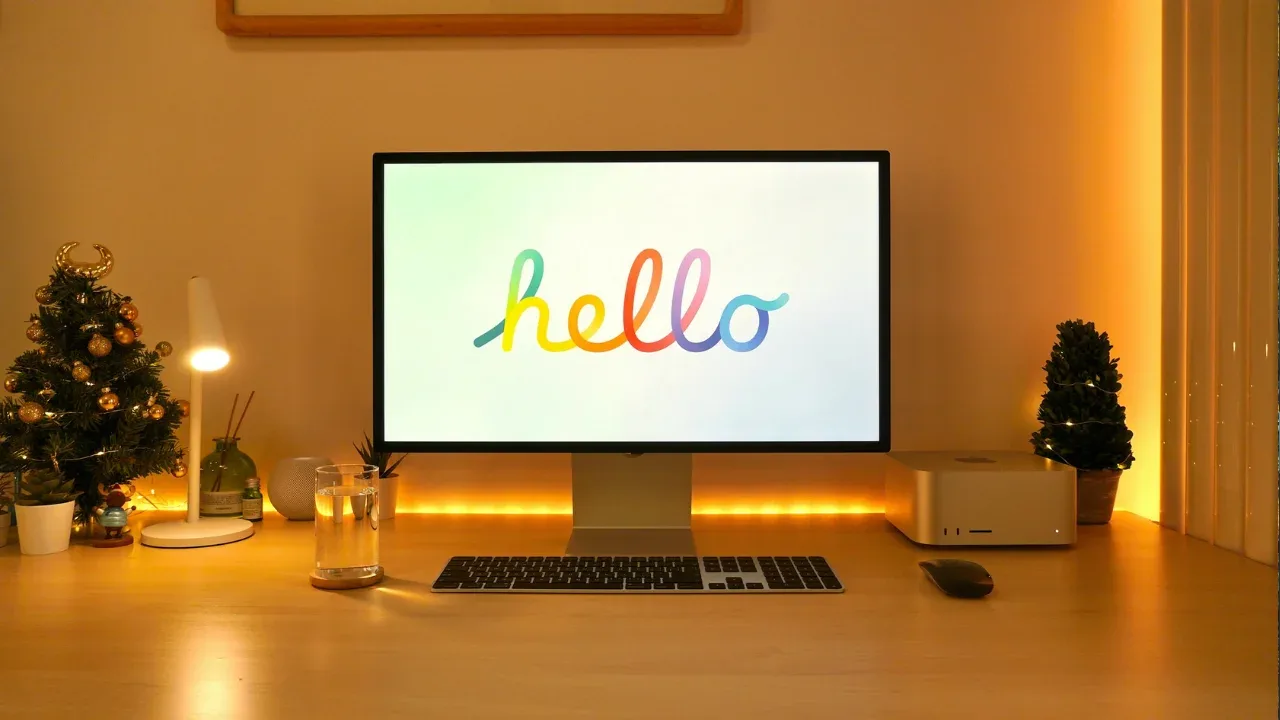
Can I inject a service into a directive in AngularJS? 😕💉
AngularJS provides us with the ability to inject services into various components, including directives. However, there seems to be an issue with the code snippet provided in the question.
Let's break it down and figure out what might be going wrong, and then we'll provide an easy solution to fix the problem. 💪🚀
The Error: Unknown provider: myDataProvider ❌
The error message "Unknown provider: myDataProvider" indicates that AngularJS could not find the specified provider, myData
, which is needed to inject into the changeIt
directive.
Understanding the Code Snippet 📃
Here is the code snippet that is causing the error:
var app = angular.module('app',[]);
app.factory('myData', function(){
return {
name : "myName"
}
});
app.directive('changeIt',function($compile, myData){
return {
restrict: 'C',
link: function (scope, element, attrs) {
scope.name = myData.name;
}
}
});
Analyzing the Issue 🕵️♀️
The problem lies in the order of injection. In AngularJS, when you define a directive, you also define the dependencies to be injected, if any. In this case, we want to inject the myData
service. However, the $compile
and myData
dependencies are not in the correct order.
The Solution: Reordering the Dependencies 🔄
To fix this issue, we need to ensure that the dependencies are in the correct order when defining the directive. In our case, we want to inject myData
as the second dependency. Here's the updated code:
var app = angular.module('app',[]);
app.factory('myData', function(){
return {
name: "myName"
}
});
app.directive('changeIt', ['$compile', 'myData', function($compile, myData){
return {
restrict: 'C',
link: function (scope, element, attrs) {
scope.name = myData.name;
}
}
}]);
By passing '$compile'
and 'myData'
as strings in an array before the directive function, we explicitly define the dependency order.
Conclusion and Call-to-Action 📣📝
And there you have it! By fixing the dependency order, the 'Unknown provider' error should no longer occur. 👍
Keep in mind that this solution applies to this specific problem but may also help in similar cases where you need to inject a service into a directive in AngularJS.
If you found this guide helpful, make sure to share it with your fellow AngularJS enthusiasts! And if you have any further questions or suggestions, feel free to leave a comment below. Let's learn, explore, and solve programming challenges together! 💡🌟
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
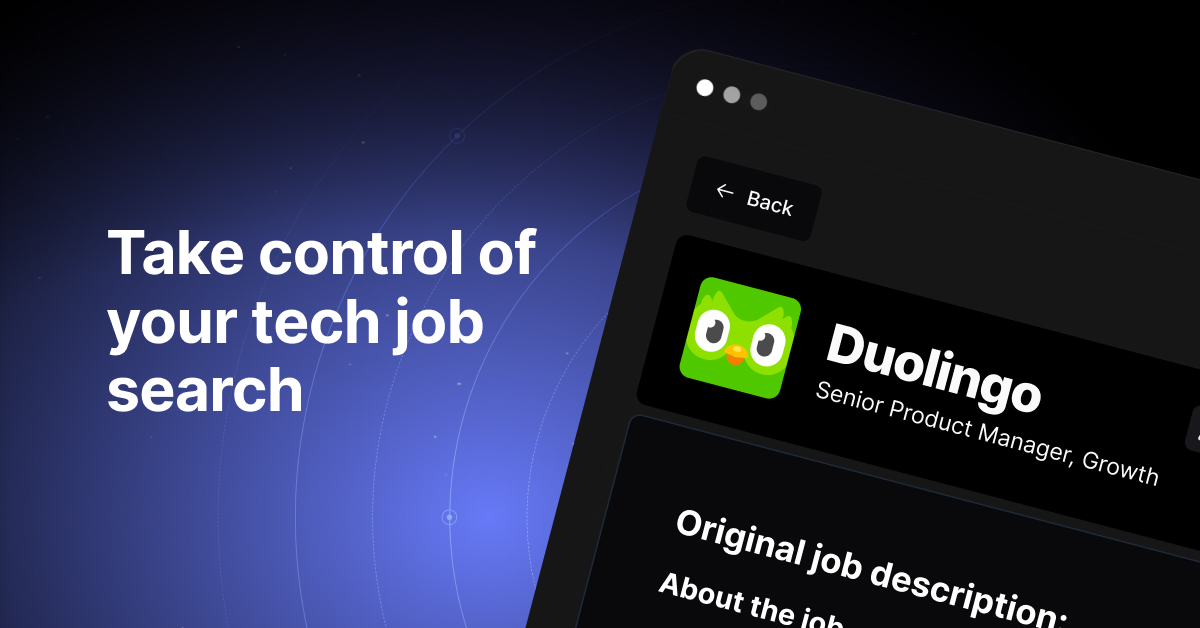