Can an AngularJS controller inherit from another controller in the same module?
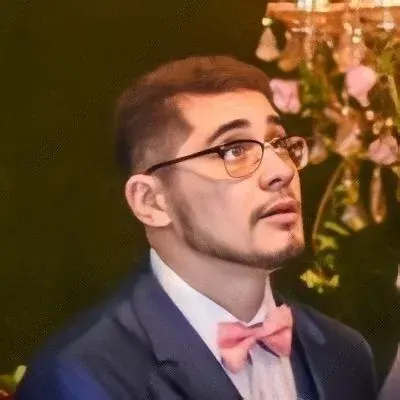
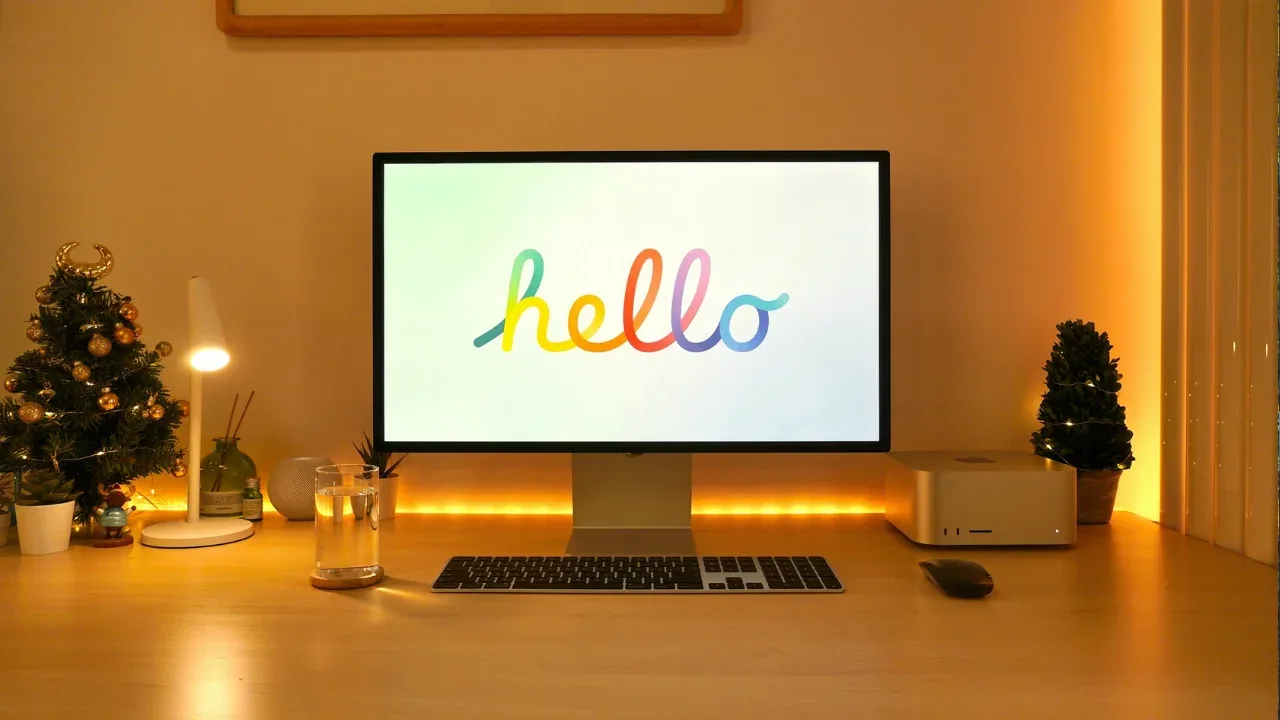
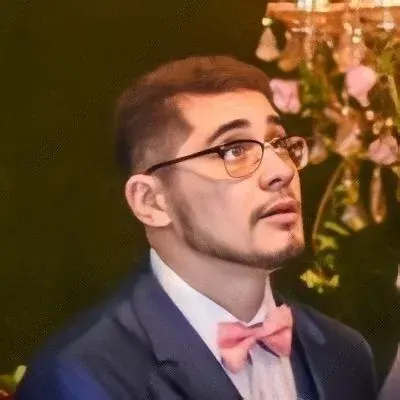
🔍 Understanding Controller Inheritance in AngularJS
AngularJS is a powerful JavaScript framework that allows developers to build dynamic web applications. One of the key features of AngularJS is the concept of controllers, which are responsible for handling the logic and data of a particular view.
But what happens when you want one controller to inherit properties from another controller within the same module? Can it be done? 🤔
🔀 Inheriting from an Outside Controller
AngularJS does provide a way for a controller to inherit properties from an outside controller. Here's an example:
var app = angular.module('angularjs-starter', []);
var ParentCtrl = function ($scope, $location) {
// Parent controller logic here
};
app.controller('ChildCtrl', function($scope, $injector) {
$injector.invoke(ParentCtrl, this, {$scope: $scope});
});
In this example, the ChildCtrl
controller is inheriting properties from the ParentCtrl
controller using the $injector.invoke
function. By passing in the ParentCtrl
function as the first parameter, we're able to inherit its properties and functionality within the ChildCtrl
.
💡 Common Problem: Inheriting from a Sibling Controller
However, what if you want a controller inside a module to inherit from a sibling controller? Here's an example:
var app = angular.module('angularjs-starter', []);
app.controller('ParentCtrl', function($scope) {
// Sibling acting as parent logic here
});
app.controller('ChildCtrl', function($scope, $injector) {
$injector.invoke(ParentCtrl, this, {$scope: $scope}); // This does not work
});
In this case, the second code block does not work as expected. The $injector.invoke
function requires a function as the first parameter, but it's unable to find the reference to the ParentCtrl
controller since it's defined as a sibling within the same module.
🚀 The Solution
To overcome this problem, we can utilize AngularJS's resolve feature. By leveraging the resolve
property of the $routeProvider
, we can ensure that the sibling controller is instantiated before the child controller.
app.config(function($routeProvider) {
$routeProvider
.when('/child', {
templateUrl: 'child.html',
controller: 'ChildCtrl',
resolve: {
parentCtrl: function($controller, $rootScope) {
$controller('ParentCtrl', {
$scope: $rootScope.$new()
});
}
}
});
});
In this example, the ParentCtrl
controller is being instantiated within the resolve
property of the $routeProvider
. By doing so, we guarantee that the ParentCtrl
is available before the ChildCtrl
is instantiated, allowing for inheritance between sibling controllers.
😍 Let's Recap
Within a module, a controller CAN inherit properties from an outside controller using
$injector.invoke
.Inheriting from a sibling controller requires using the
resolve
property of the$routeProvider
.
Now that you understand controller inheritance in AngularJS, you can take your web applications to the next level! 💪
💌 Call-to-Action: Engage with Us!
We hope you found this guide helpful! If you have any questions or would like more insights into AngularJS or any programming topic, please let us know in the comments below. We love hearing from our readers and are dedicated to providing the best content to help you become a better developer. Don't forget to share this blog post with your friends and colleagues who might find it useful too! 🌟