Cache an HTTP "Get" service response in AngularJS?
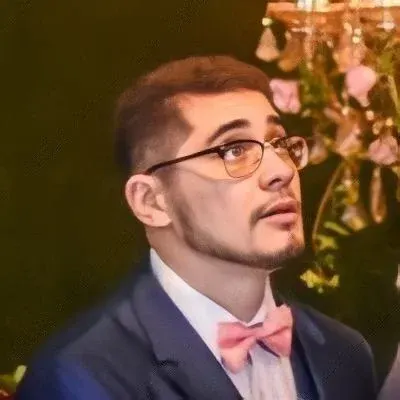
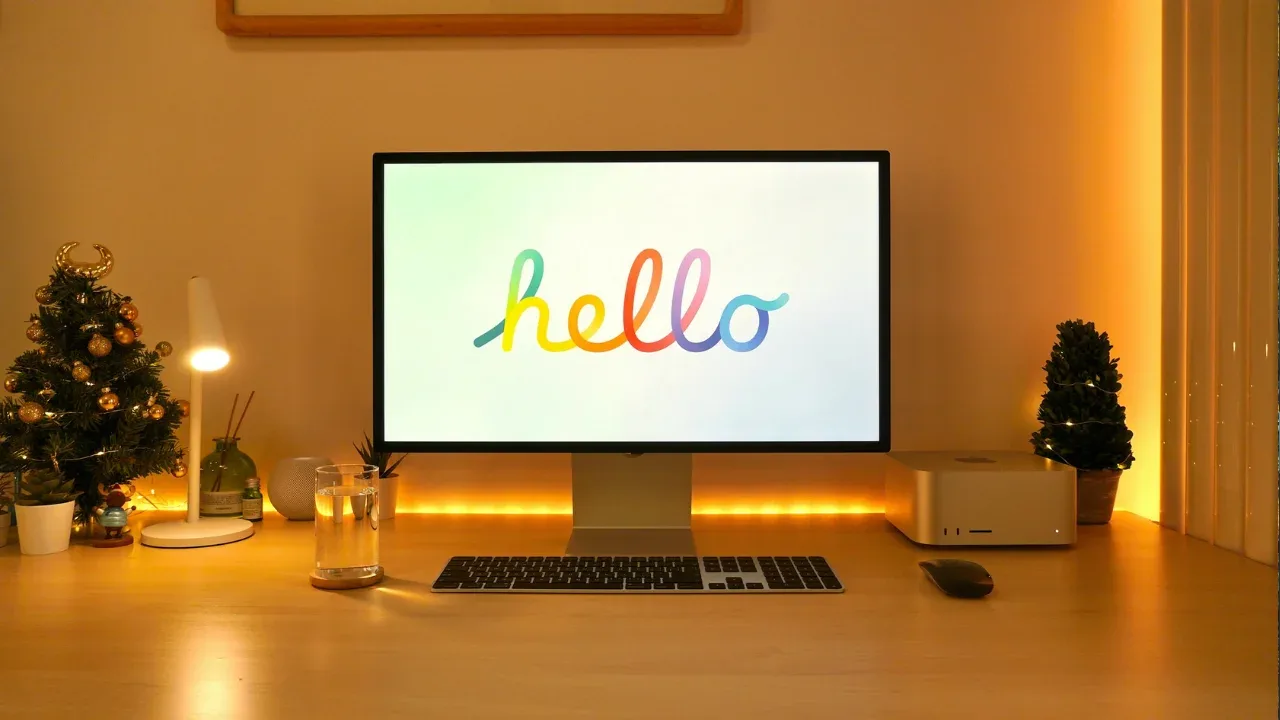
How to Cache an HTTP 'Get' Service Response in AngularJS 🔄
Are you tired of making repetitive HTTP 'Get' requests in your AngularJS application? 🤔 Imagine if you could simply cache the response data and avoid the overhead of making unnecessary server calls. Well, you're in luck! In this blog post, we'll discuss how you can implement caching in your AngularJS service to improve performance and reduce network traffic. Let's get started! 💪
The Problem: Making Unneeded HTTP Requests 😫
Consider a scenario where you have a custom AngularJS service that makes an HTTP 'Get' request to fetch some data. Each time the service is called, it sends an HTTP request to the server, even if the data object is already populated. This results in unnecessary network traffic and slow response times. 😥
As an AngularJS developer, you want to optimize this process and cache the response data, so subsequent calls to the service can quickly return the cached data without hitting the server again. But is it possible to achieve this? 🤔
The Solution: Caching the Response Data with $http and $cacheFactory 🚀
Fortunately, AngularJS provides us with the necessary tools to implement caching in our HTTP 'Get' service. We can make use of the $http
service to make the initial request, and the $cacheFactory
service to store the response data for future use. Here's a step-by-step guide:
Create a Caching Service: Start by creating a separate AngularJS service that will handle the caching functionality. Let's call it
DataCacheService
. Within this service, create an instance of$cacheFactory
, which will serve as our cache container.Make the Initial Request: In your custom service, use the
$http
service to make the HTTP 'Get' request and fetch the data from the server. Once the request is successful, store the response data in the cache usingDataCacheService
.Checking for Cached Data: Before making a subsequent request, add a check to your service to verify if the response data is already present in the cache. If so, return the cached data instead of making another server call.
Refreshing the Cache: If you need to update the data in your cache, you can implement a refresh mechanism. Simply make a new HTTP 'Get' request to fetch the updated data and save it in the cache. This way, you ensure that you always have the most recent data available.
Example Implementation: 📝
Let's take a look at a simplified example of how this caching mechanism can be implemented in an AngularJS service:
angular.module('app').service('CustomService', function($http, DataCacheService) {
// Make the initial HTTP 'Get' request and cache the response data
this.getData = function() {
if (!DataCacheService.get('cachedData')) {
$http.get('/api/data').then(function(response) {
DataCacheService.put('cachedData', response.data);
});
}
return DataCacheService.get('cachedData');
};
// Refresh the cache with updated data
this.refreshData = function() {
$http.get('/api/data').then(function(response) {
DataCacheService.put('cachedData', response.data);
});
};
});
Engage with Us! 📩
Are you excited to implement caching in your AngularJS application now? 💡 We encourage you to try out this approach and see the difference it can make in terms of performance and network efficiency. Have questions or thoughts? We'd love to hear from you in the comments section below! Let's make our AngularJS apps faster and better together. 😄🚀
Conclusion 🎉
Caching HTTP 'Get' service responses in AngularJS can greatly improve the performance of your application by reducing unnecessary server calls. By implementing this mechanism using $http
and $cacheFactory
, you can cache the response data and return it quickly for subsequent calls. 🔄✨
Remember, optimizing your application's performance is not just about writing efficient code; it's about making the best use of available resources. So, why not give caching a try in your AngularJS app today? Your users will thank you for the speedy experience! 🙌💨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
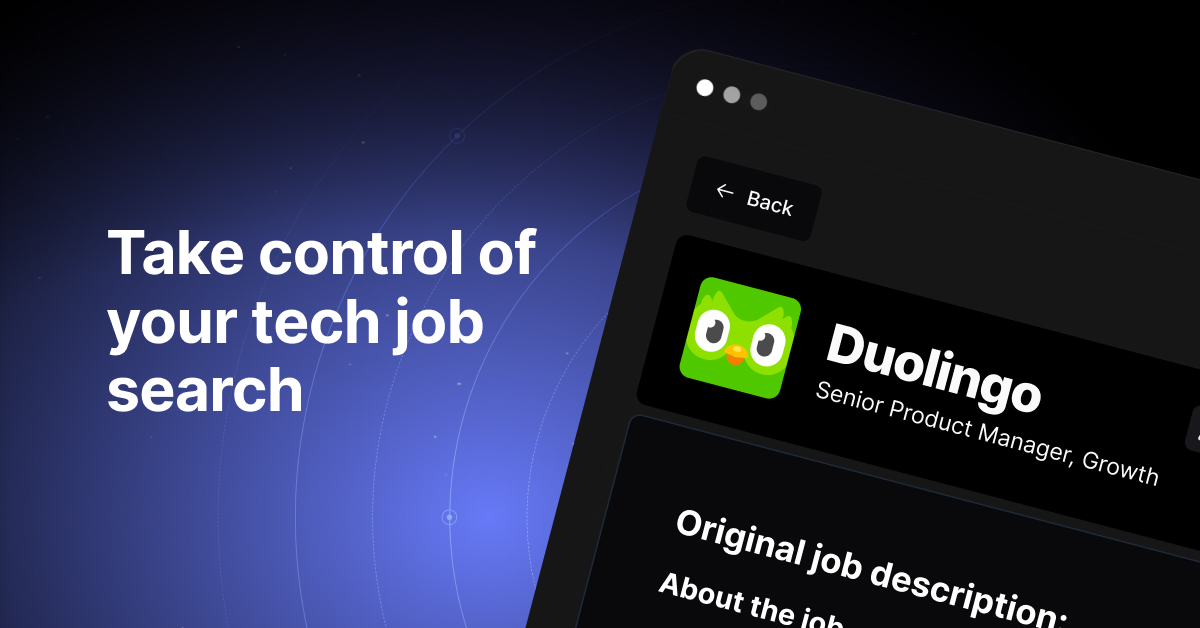