Best way to represent a Grid or Table in AngularJS with Bootstrap 3?
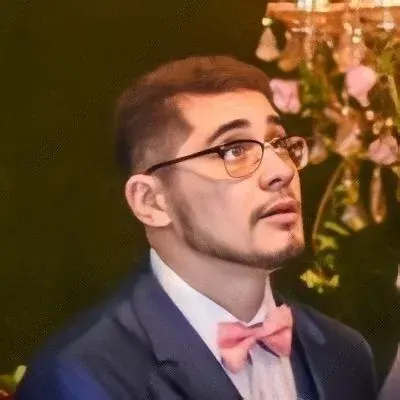
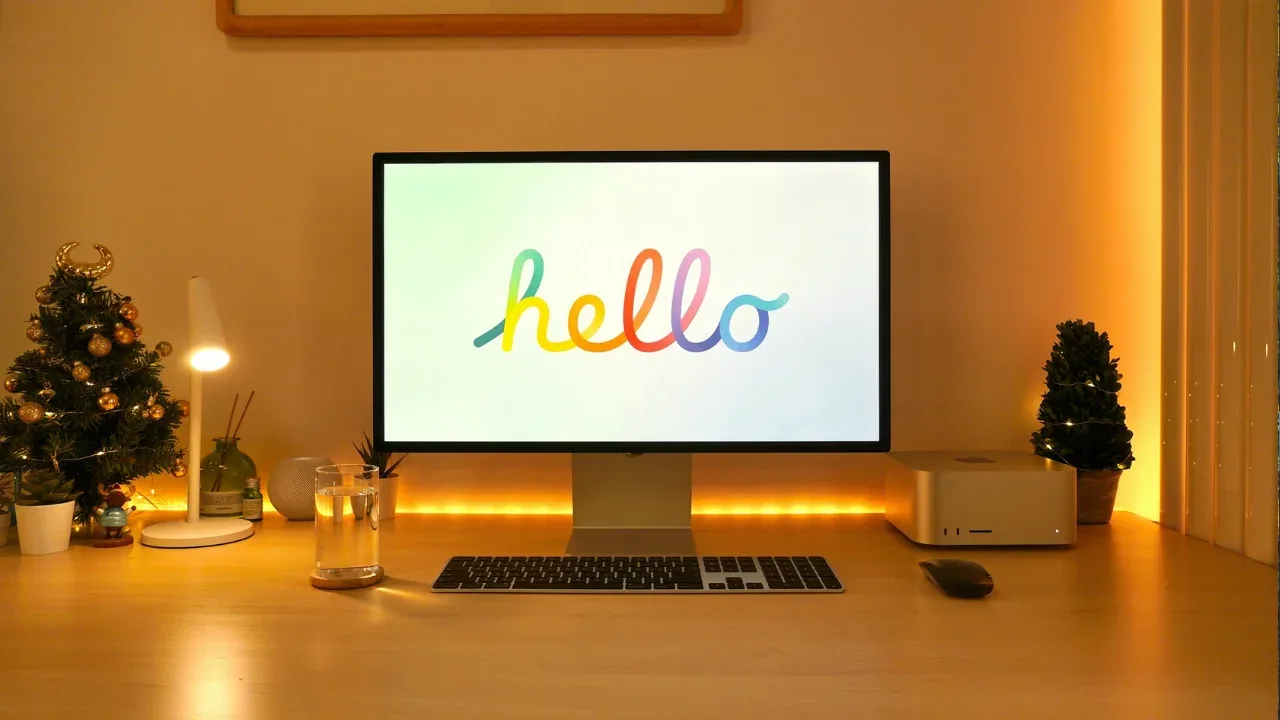
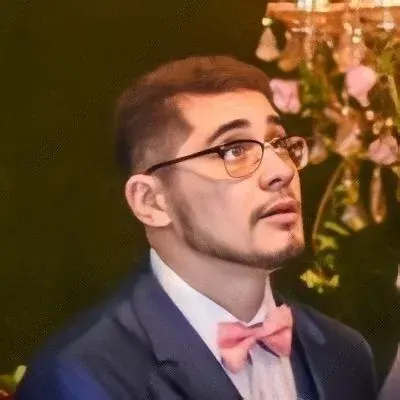
Best way to represent a Grid or Table in AngularJS with Bootstrap 3?
Are you building an AngularJS app with Bootstrap 3 and need to display a large grid or table? 📊👨💻 Look no further! In this blog post, we'll explore the best available control for AngularJS and Bootstrap that offers features like Sorting, Searching, Pagination, and more. 💪
The Problem 🤔
The questioner wants to display a table/grid with thousands of rows. While AngularJS and Bootstrap are great tools for building web applications, finding the right control to handle large amounts of data can be challenging. 👀
The Solution 🎉
Fortunately, there's a fantastic library that combines the power of AngularJS and Bootstrap: ngTable.⚡️
Step 1: Setup the Environment 🛠️
Before we dive into using ngTable, make sure you have AngularJS and Bootstrap 3 included in your project. Install ngTable using npm with the following command:
npm install ng-table
Step 2: Implement ngTable in Your App 📝
Now that we have ngTable, let's see how we can integrate it into our AngularJS app. First, include the required scripts in your HTML file:
<link rel="stylesheet" href="path/to/bootstrap.css">
<script src="path/to/angular.js"></script>
<script src="path/to/ng-table.js"></script>
Next, inject ngTable
into your app's module:
angular.module('myApp', ['ngTable']);
Step 3: Create a Table Using ngTable 🚀
Now that the setup is complete, it's time to create your table using ngTable directives. In your HTML file, use the following code:
<div ng-app="myApp" ng-controller="MyController">
<table ng-table="tableParams" class="table">
<tr ng-repeat="user in users">
<td data-title="'Name'">{{user.name}}</td>
<td data-title="'Email'">{{user.email}}</td>
</tr>
</table>
</div>
In your app's JavaScript file, define the MyController
controller and set up the $scope.tableParams
object:
angular.module('myApp').controller('MyController', function($scope, ngTableParams) {
$scope.tableParams = new ngTableParams({}, {
dataset: $scope.users
});
});
Step 4: Customize with Features 🌟
Now that you have a basic table, you can easily add features like Sorting, Searching, Pagination, and more.
For example, to enable Sorting, modify your MyController
controller as follows:
angular.module('myApp').controller('MyController', function($scope, ngTableParams) {
$scope.tableParams = new ngTableParams({
sorting: { name: 'asc' }
}, {
dataset: $scope.users
});
});
You can explore the ngTable documentation to learn more about available features and how to customize your table.
Conclusion 🎯
With ngTable, building a high-performance, feature-rich table or grid in AngularJS and Bootstrap 3 becomes a breeze. It provides easy solutions for displaying large amounts of data while offering essential features like Sorting, Searching, Pagination, and more.
So go ahead, give ngTable a try in your next AngularJS project, and unlock the power of data representation! 💪🔥
Have you tried ngTable in your AngularJS app? Share your experience and any additional tips in the comments below. Let's create amazing tables together! 😄🚀
Share this post with your friends and fellow developers who might be struggling with table/grid representation in AngularJS and Bootstrap!
References: