Angularjs ng-model doesn"t work inside ng-if
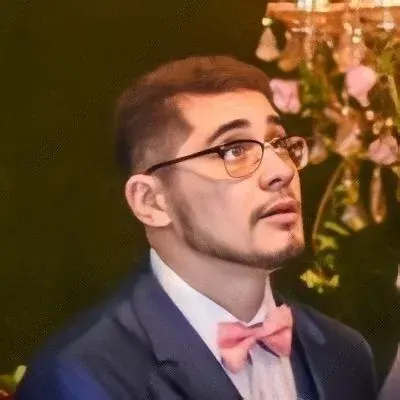
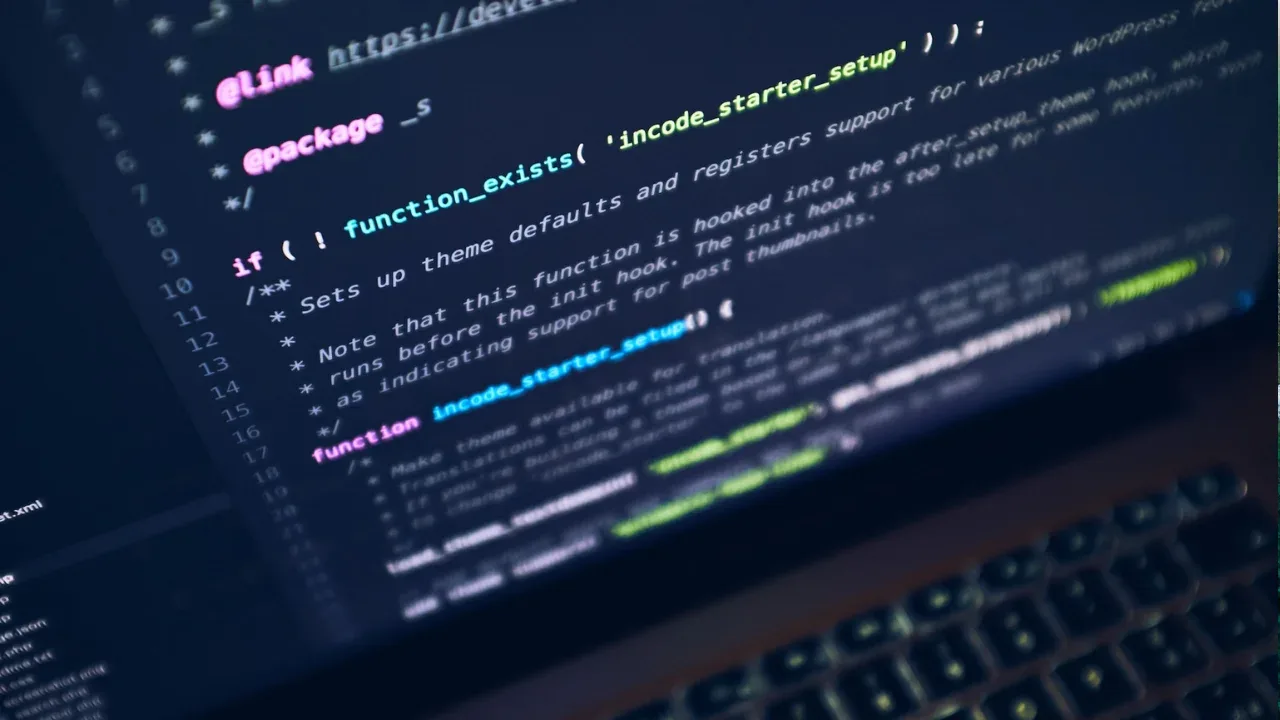
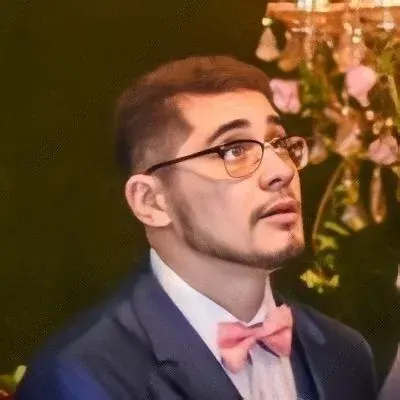
Solving the Mystery of ng-model
Inside ng-if
in AngularJS 🕵️♂️🔍🔧
So, you started coding in AngularJS and encountered a peculiar issue: ng-model
doesn't seem to work when used inside an ng-if
directive 🤔. Don't worry, you're not alone! Many developers have stumbled upon this problem and scratched their heads 🤯.
In this blog post, we'll dive into the depths of this issue, understand why it happens, and provide you with easy solutions to make ng-model
play nicely inside ng-if
. Let's get started! 💪
The Mystery of the Disappearing ng-model
👻
To understand the problem at hand, let's examine the code provided in the question:
<div ng-app>
<div ng-controller="main">
Test A: {{testa}}<br />
Test B: {{testb}}<br />
Test C: {{testc}}<br />
<div>
testa (without ng-if): <input type="checkbox" ng-model="testa" />
</div>
<div ng-if="!testa">
testb (with ng-if): <input type="checkbox" ng-model="testb" />
</div>
<div ng-if="!someothervar">
testc (with ng-if): <input type="checkbox" ng-model="testc" />
</div>
</div>
</div>
In this code snippet, we have three checkboxes (Test A
, Test B
, and Test C
) bound to their respective ng-model
variables (testa
, testb
, and testc
). The issue arises when the second checkbox (Test B
) is placed inside an ng-if
directive.
Unveiling the Root Cause 👩💻🔬
The problem lies in the way ng-if
creates a child scope. When an ng-if
evaluates to true
, it creates a new child scope, inheriting from the parent scope. However, when it evaluates to false
, the child scope is destroyed altogether.
Here's what happens in our code:
Initially,
testa
,testb
, andtestc
are undefined in the parent scope.The first checkbox (
Test A
) works perfectly fine because it doesn't rely onng-if
.When
testa
is checked, it becomestrue
in the parent scope.The second checkbox (
Test B
) is wrapped inside anng-if
. Whentesta
istrue
, theng-if
evaluates tofalse
, causing the child scope to be destroyed along withtestb
and its bindings.As a result, the second checkbox (
Test B
) no longer updates its correspondingng-model
variable (testb
).
Easy Solutions to the Rescue! 🚀✨
Fortunately, we have a few simple solutions to make ng-model
work smoothly within ng-if
. Let's explore them one by one:
Solution 1: Use ng-show
instead of ng-if
By replacing ng-if
with ng-show
, we can avoid destroying the child scope altogether. ng-show
simply toggles the display
CSS property, keeping the child scope intact.
<div ng-show="!testa">
testb (with ng-show): <input type="checkbox" ng-model="testb" />
</div>
Solution 2: Move ng-if
to a parent element
Another approach is to encapsulate the ng-if
condition at a higher level, so the child scope isn't destroyed. This way, the ng-model
will persist even when the condition evaluates to false
.
<div ng-controller="main">
Test A: {{testa}}<br />
<div ng-if="!testa">
Test B: {{testb}}<br />
<input type="checkbox" ng-model="testb" />
</div>
<div ng-if="!someothervar">
Test C: {{testc}}<br />
<input type="checkbox" ng-model="testc" />
</div>
</div>
Solution 3: Use an object to store your variables
Instead of binding checkboxes directly to primitive variables (testa
, testb
, etc.), create an object in the parent scope and bind the checkboxes to the properties of that object.
<div ng-controller="main">
Test A: {{data.testa}}<br />
<div ng-if="!data.testa">
Test B: {{data.testb}}<br />
<input type="checkbox" ng-model="data.testb" />
</div>
<div ng-if="!someothervar">
Test C: {{data.testc}}<br />
<input type="checkbox" ng-model="data.testc" />
</div>
</div>
app.controller('main', function($scope) {
$scope.data = {
testa: false,
testb: false,
testc: false
};
});
Using this approach, the object (data
) acts as a reference to the checkboxes' states, ensuring they persist within the ng-if
directive.
Engage with us! 🤝💬
We hope this guide helped unveil the mystery of ng-model
inside ng-if
for you. If you have any further questions or comments, we'd love to hear from you! Join the conversation by leaving a comment below. Let's solve AngularJS mysteries together! 😄💪
In the meantime, feel free to share this post with your fellow developers who might be facing the same issue. Sharing is caring! 😉🚀
Stay curious, keep coding, and embrace the magic of AngularJS! ✨🔥