AngularJS ng-class if-else expression
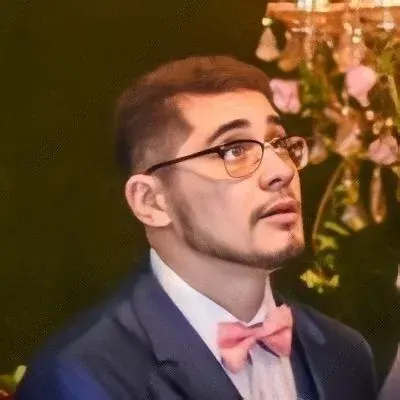
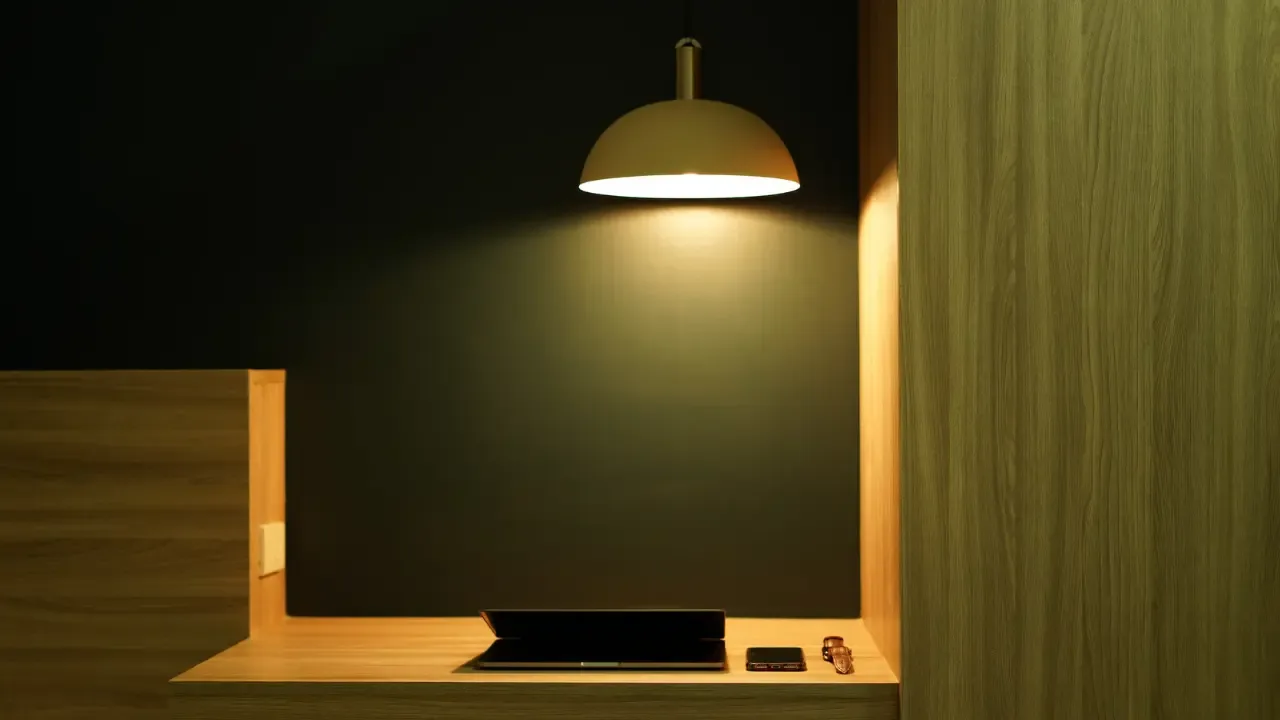
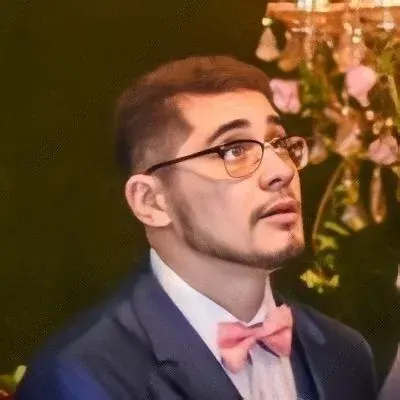
AngularJS ng-class if-else expression
Are you having trouble with the ng-class
directive in AngularJS? Specifically, are you wondering if you can use an if-else expression to simplify your code and avoid specifying each value? Well, you've come to the right place! In this blog post, we'll address this common issue and provide you with easy solutions.
The Problem
Let's take a look at a typical use case of ng-class
in AngularJS:
<div class="bigIcon" data-ng-click="PickUp()" ng-class="{first:'classA', second:'classB', third:'classC', fourth:'classC'}[call.State]"></div>
In the above example, the ng-class
directive is used to dynamically apply different CSS classes based on the value of call.State
. This is a powerful feature that allows you to easily switch between styles based on different states or conditions.
Now, let's say you want to apply classC
whenever call.State
differs from first
or second
. Can you achieve this using an if-else expression?
The Solution
Unfortunately, AngularJS does not support using an if-else expression directly in the ng-class
directive. However, there are a couple of simple workarounds you can use to achieve the desired result.
Solution 1: Using a Function
One way to solve this problem is by creating a function in your controller that returns the appropriate class based on the value of call.State
.
<div class="bigIcon" data-ng-click="PickUp()" ng-class="getClass(call.State)"></div>
Here's an example implementation of the getClass
function in your controller:
$scope.getClass = function(state) {
if (state === 'first' || state === 'second') {
return {
'classA': true,
'classB': true
};
} else {
return 'classC';
}
};
In the above code, we check if state
is equal to first
or second
. If it is, we return an object with classA
and classB
set to true
, which applies the corresponding CSS classes. Otherwise, we simply return 'classC'
, resulting in the classC
CSS class being applied.
Solution 2: Using a Ternary Operator
Another way to solve this problem is by using the ternary operator within the ng-class
directive.
<div class="bigIcon" data-ng-click="PickUp()" ng-class="call.State === 'first' || call.State === 'second' ? { 'classA': true, 'classB': true } : 'classC'"></div>
In this solution, we use the ternary operator to check if call.State
is equal to first
or second
. If it is, we return an object with classA
and classB
set to true
. Otherwise, we simply return 'classC'
.
Conclusion
While AngularJS does not directly support an if-else expression in the ng-class
directive, there are workarounds you can use to achieve the desired result. By creating a function or using the ternary operator, you can simplify your code and avoid specifying each value for different states.
Now that you have learned these easy solutions, go ahead and try them out in your AngularJS projects. Don't let ng-class
confusion hold you back from creating awesome web applications! 💪
If you have any questions or further insights on this topic, we would love to hear from you. Share your thoughts in the comments below and let's engage in a lively discussion! 👇