AngularJS For Loop with Numbers & Ranges
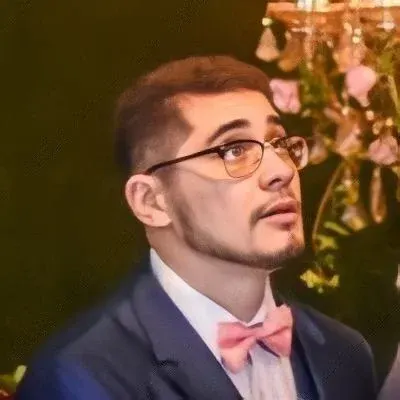
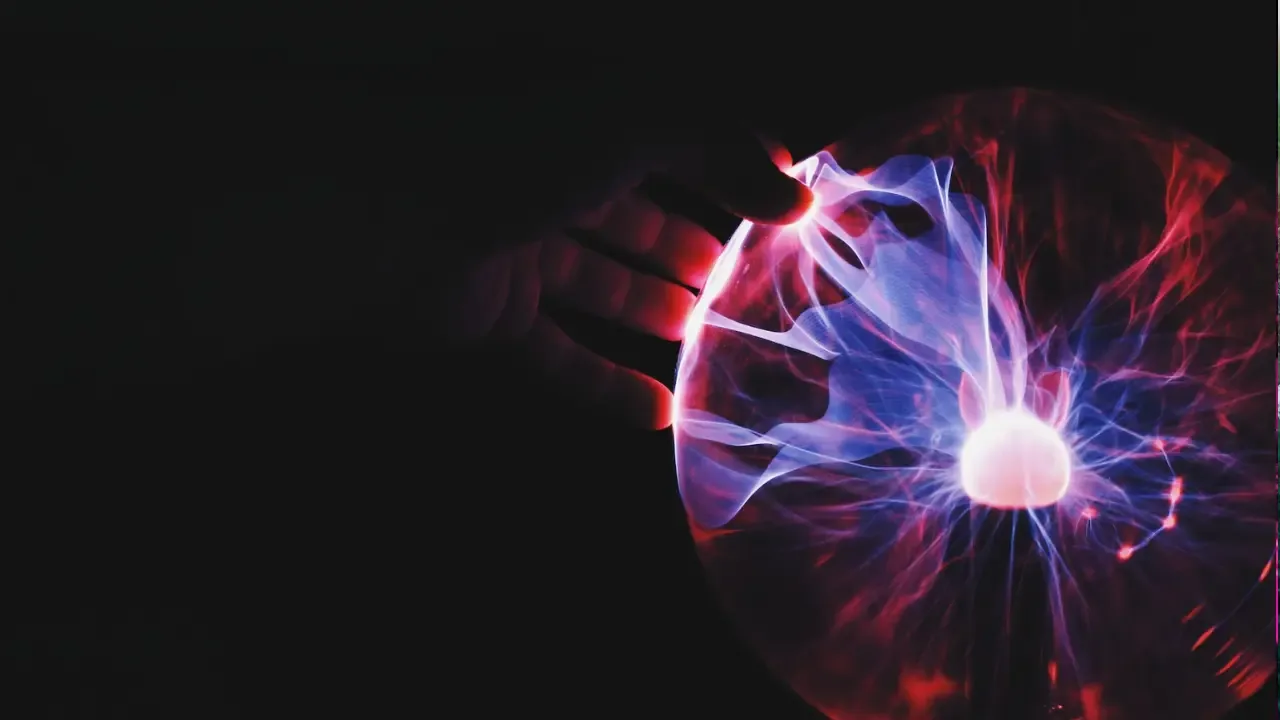
AngularJS For Loop with Numbers & Ranges
AngularJS provides a convenient way to iterate over arrays using the ng-repeat
directive. However, when it comes to using numbers or ranges in a loop, things can get a bit tricky. In this blog post, we'll address the common issues associated with for loops using numbers and ranges in AngularJS, provide easy solutions, and show you how to implement it in your code.
The Problem
By default, AngularJS supports for loops using numbers within its HTML directives like this:
<div data-ng-repeat="i in [1, 2, 3, 4, 5]">
do something
</div>
But what if you have a dynamic number as a range and want to loop over it? The approach mentioned above requires creating an empty array in the controller and pushing the desired range values into it. The code might look like this:
var range = [];
for (var i = 0; i < total; i++) {
range.push(i);
}
$scope.range = range;
And then in the HTML, you would use:
<div data-ng-repeat="i in range">
do something
</div>
While this solution works, it adds unnecessary complexity as we won't be using the range array within the loop.
The Solution
Fortunately, there is a simpler way to achieve the desired result without the need for an additional array. AngularJS allows you to set a range or define a regular for loop using min/max values. Although this feature doesn't exist by default, you can implement it with a custom filter or by extending AngularJS's built-in ng-repeat
directive.
Here's an example of what it might look like using a custom filter:
app.filter('range', function () {
return function (min, max, step) {
step = step || 1;
var input = [];
for (var i = min; i <= max; i += step) {
input.push(i);
}
return input;
};
});
In the HTML, you can now use the range
filter like this:
<div data-ng-repeat="i in 1 | range:100">
do something
</div>
This code snippet will iterate from 1 to 100, providing you with the desired for loop functionality without the need for unnecessary variables.
Conclusion
By leveraging custom filters or extending AngularJS's built-in directives, you can simplify for loops using numbers and ranges in AngularJS. This not only reduces code complexity but also improves readability and maintainability of your codebase.
Next time you encounter a scenario where you need to loop over a range of numbers in AngularJS, consider using the techniques mentioned in this blog post. Don't let unnecessary complexity bog down your code - find clever solutions that make your code more efficient and elegant.
Have you encountered any other interesting ways to implement for loops using numbers or ranges in AngularJS? Share your insights in the comments below and let's learn together!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
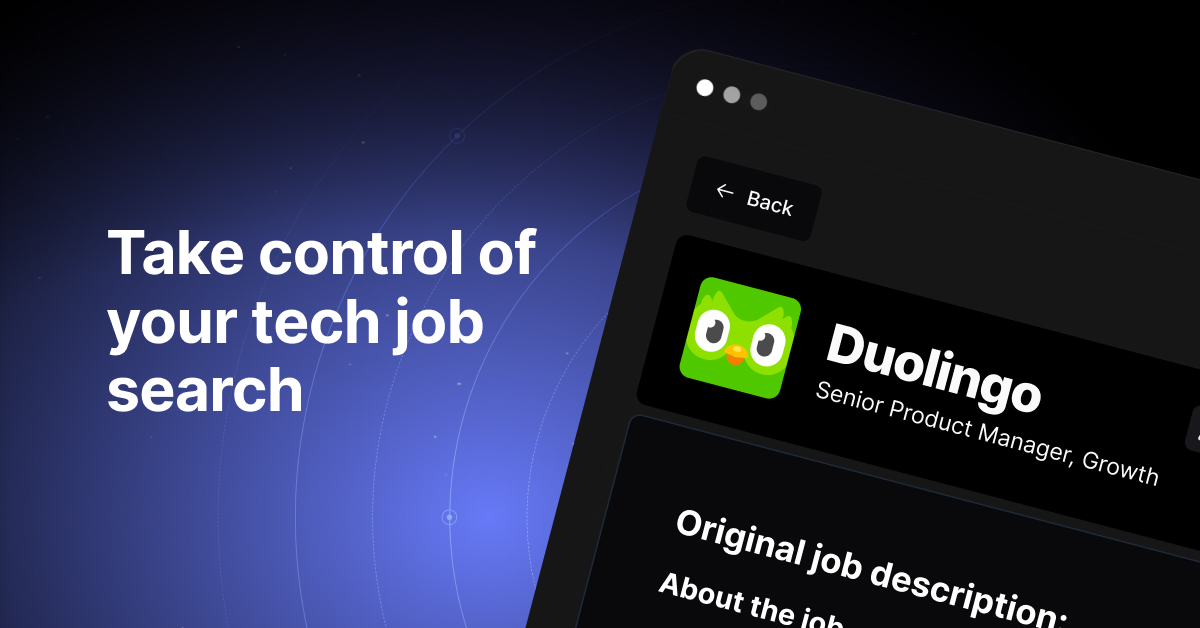