AngularJS - Does $destroy remove event listeners?
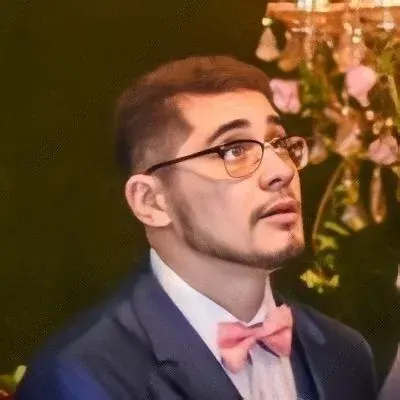
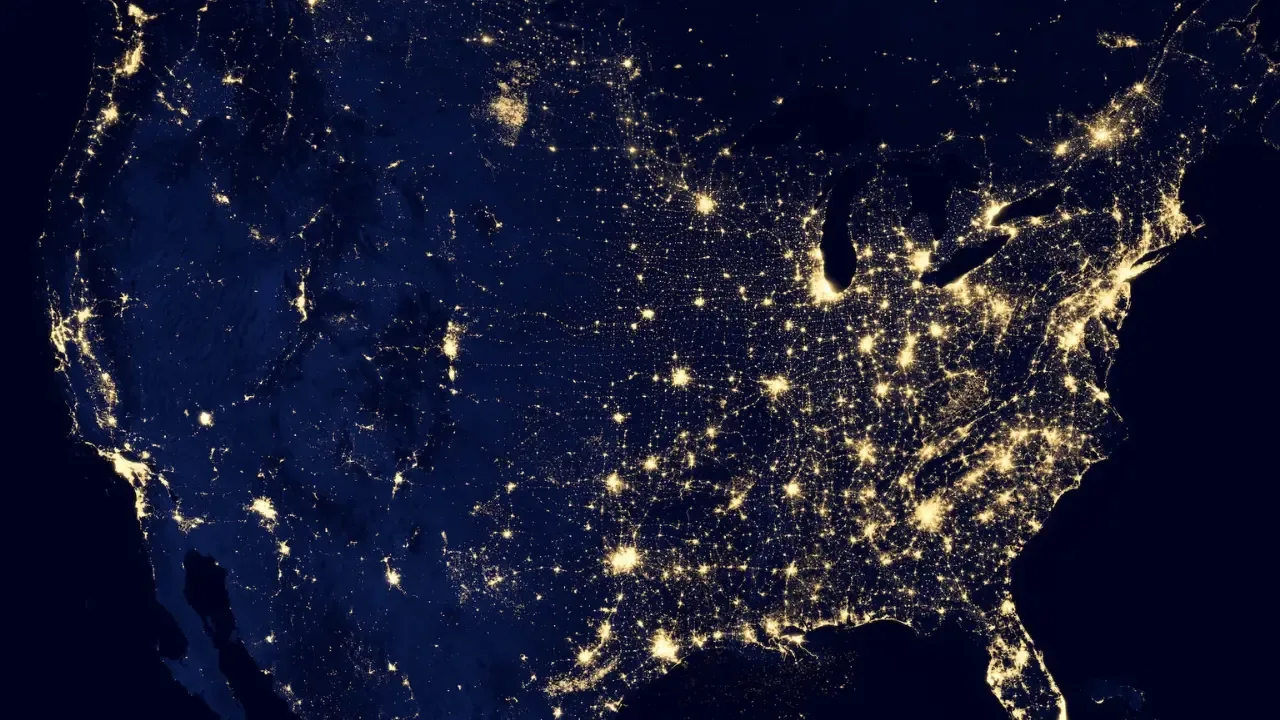
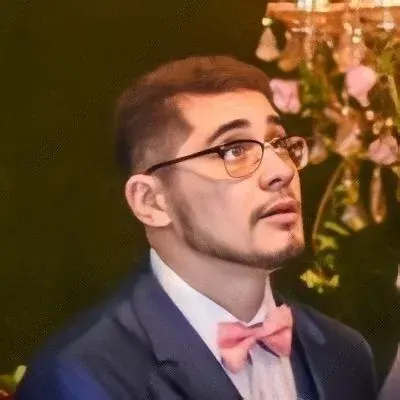
AngularJS - Does $destroy remove event listeners? ๐๏ธ๐ง
So you've built an awesome AngularJS app and you're worried about memory leaks caused by event listeners. Don't worry, we've got you covered! In this blog post, we'll answer the burning questions around AngularJS' $destroy event and whether it removes event listeners.
Understanding the problem ๐ค
According to the AngularJS documentation, event listeners registered to scopes and elements are automatically cleaned up when they are destroyed. However, if you registered a listener on a service or a DOM node that isn't being deleted, you'll have to clean it up yourself to avoid memory leaks.
Solution 1: Using element.on('$destroy', ...) ๐งน๐ซ
To ensure proper cleanup of event listeners in your AngularJS directive, you can use the $destroy
event. This event is emitted when the directive is removed from the DOM. By listening to this event, you can run a clean-up function to remove your event listener.
Here's an example of how you can use the $destroy
event:
element.on('$destroy', function() {
element.off('click', eventListener);
});
In this example, element
represents the DOM element to which the event listener is attached, and eventListener
is the function that handles the event. By calling element.off('click', eventListener)
inside the $destroy
event handler, you remove the event listener, ensuring it won't cause any memory leaks.
Solution 2: Using scope.$on('$destroy', ...) โ๏ธ๐ซ
Another way to clean up event listeners in AngularJS is by using the $destroy
event on the directive's scope. The process is similar to the previous solution, but the event is emitted on the scope instead of the element.
Here's an example of how you can use the $destroy
event on the scope:
scope.$on('$destroy', function() {
element.off('click', eventListener);
});
In this example, scope
represents the directive's scope, and element.off('click', eventListener)
inside the $destroy
event handler removes the event listener, ensuring proper cleanup.
Answering the questions โ
Now let's address the questions from the original context:
When the directive is destroyed, are there any memory references to the
element.on
to keep it from being garbage collected?No, there are no memory references that keep the
element.on
from being garbage collected. As long as you remove the event listener when the directive is destroyed using one of the solutions described above, theelement.on
function will be properly cleaned up.
Angular documentation states that I should use a handler to remove event listeners on the
$destroy
emitted event. I was under the impression thatdestroy()
removed event listeners, is this not the case?Yes, you were correct in assuming that the
destroy()
function removes event listeners. However, this function is used to destroy a scope, not to remove event listeners. To ensure proper cleanup of event listeners, you should use eitherelement.on('$destroy', ...)
orscope.$on('$destroy', ...)
, as explained in the solutions above.
Conclusion and call-to-action ๐ข๐ช
Event listeners can be a potential source of memory leaks in AngularJS apps, but with the power of the $destroy event, you can easily clean them up. By using either element.on('$destroy', ...)
or scope.$on('$destroy', ...)
, you can ensure that event listeners are removed when components are destroyed, preventing memory leaks and maintaining the performance of your app.
So go ahead, dive into your codebase, find those event listeners, and give them the clean-up they deserve! Your app and your users will thank you. And don't forget to share this blog post with your fellow AngularJS developers to help them prevent memory leaks too! ๐๐
If you have any questions or want to share your experiences with cleaning up event listeners, feel free to leave a comment below. Let's keep our AngularJS apps clean and efficient together! ๐๐ป