AngularJS - Binding radio buttons to models with boolean values
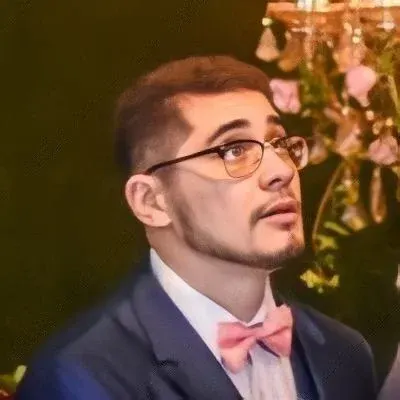
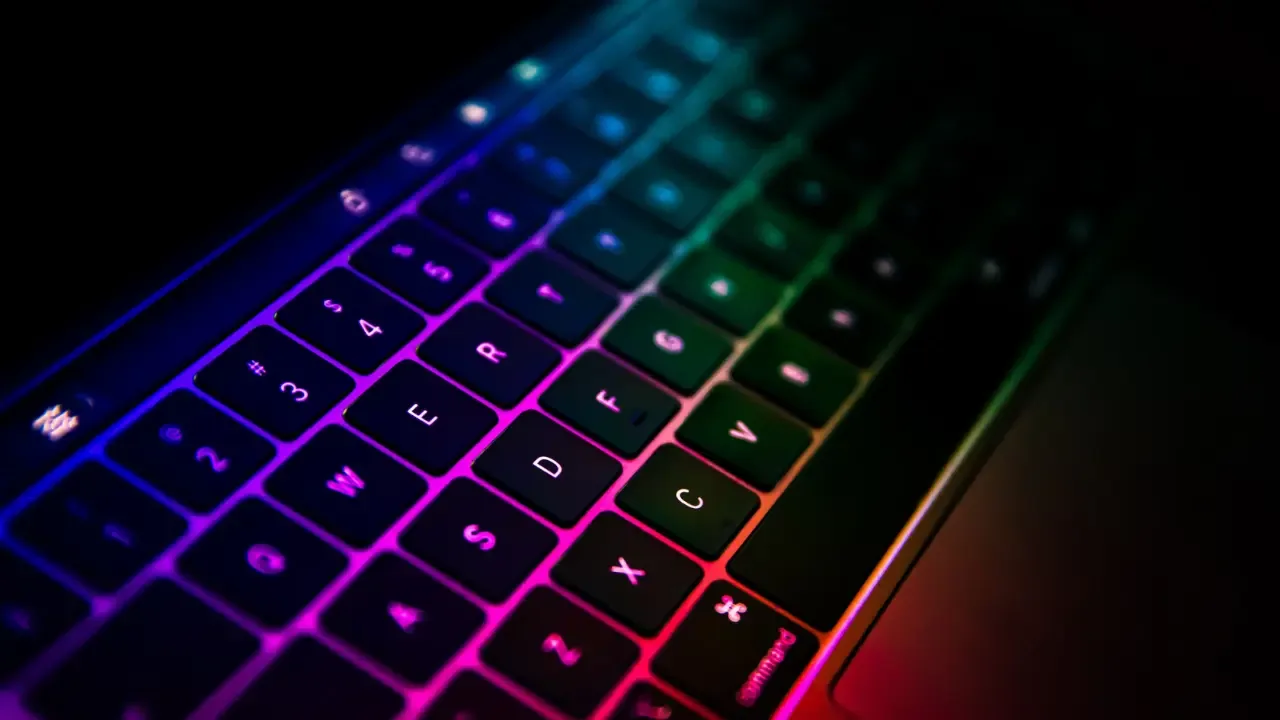
Binding Radio Buttons to Models with Boolean Values in AngularJS
If you're struggling with binding radio buttons to a model with boolean values in AngularJS, you're not alone. Many developers encounter this issue when trying to display exam questions or other forms that require selecting a single option from a set of choices.
The Problem
In the given example, the radio buttons are supposed to be bound to the isUserAnswer
property of each choice object. However, using true
or false
as the values for the isUserAnswer
property doesn't work as expected.
<label data-ng-repeat="choice in question.choices">
<input type="radio" name="response" data-ng-model="choice.isUserAnswer" value="true" />
{{choice.text}}
</label>
The issue is that AngularJS interprets the true
and false
values as strings when binding to the data-ng-model
directive. As a result, the radio buttons are not correctly selected based on the underlying boolean values.
The Solution
One possible solution to this problem is to enclose the boolean values in quotes within the model object.
$scope.question = {
questionText: "This is a test question.",
choices: [{
id: 1,
text: "Choice 1",
isUserAnswer: "false"
}, {
id: 2,
text: "Choice 2",
isUserAnswer: "true"
}, {
id: 3,
text: "Choice 3",
isUserAnswer: "false"
}]
};
By using string representations of boolean values, AngularJS can correctly evaluate them and bind them to the radio buttons. However, this workaround may not be ideal if you're dealing with a REST service that expects the isUserAnswer
property to be a boolean.
Alternative Approach
If changing the structure of your model or modifying the REST service's JSON serialization is not feasible, there's another way to set up the model binding without altering the model itself.
Instead of directly binding the isUserAnswer
property to the radio buttons, you can introduce an auxiliary property within the controller to handle the boolean values.
<label data-ng-repeat="choice in question.choices">
<input type="radio" name="response" data-ng-model="isUserAnswer[choice.id]" />
{{choice.text}}
</label>
In the controller, initialize the isUserAnswer
object and map each choice's id
to its corresponding isUserAnswer
value.
$scope.isUserAnswer = {};
angular.forEach($scope.question.choices, function(choice) {
$scope.isUserAnswer[choice.id] = choice.isUserAnswer;
});
This approach allows you to preserve the original structure of the model while still achieving the desired binding behavior.
Conclusion
Binding radio buttons to models with boolean values in AngularJS can be tricky, but with the right approach, you can overcome this challenge. Whether you choose to modify the model, introduce auxiliary properties, or explore other alternatives, it's important to find a solution that fits your specific use case.
If you want to see these solutions in action, check out this jsFiddle. Feel free to experiment with different scenarios and share your thoughts in the comments below.
Remember, understanding and solving these kinds of problems is an essential part of mastering AngularJS. Happy coding! 💻🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
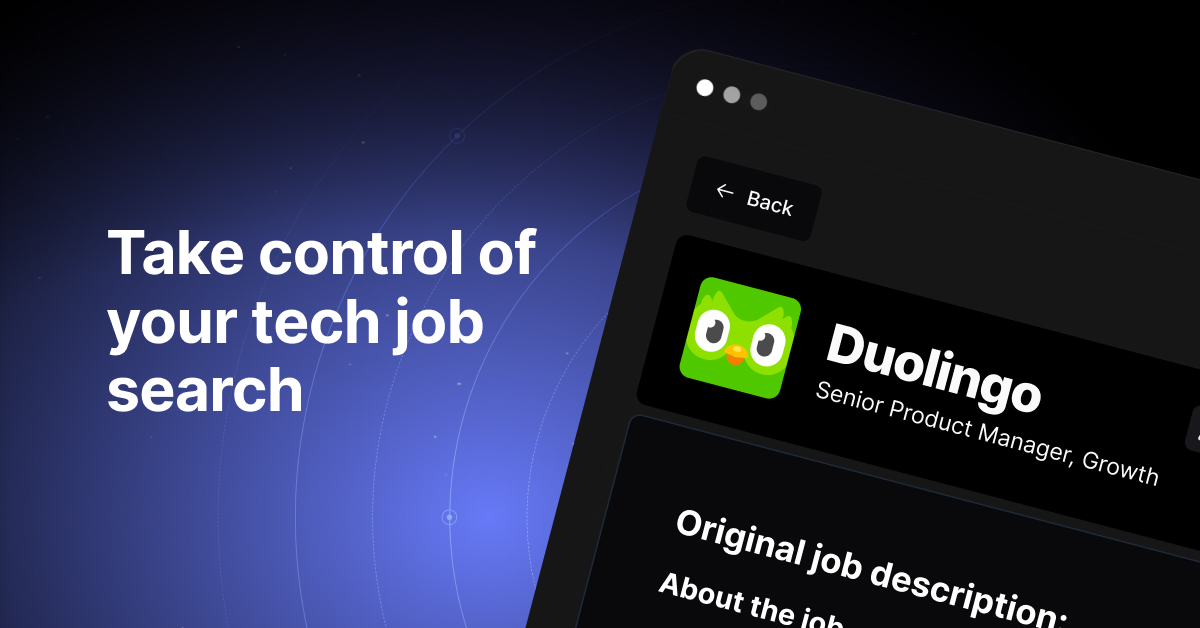