AngularJS app.run() documentation?
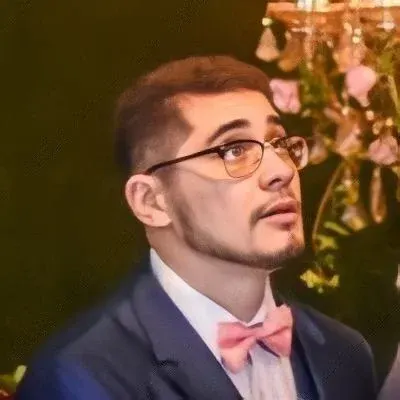
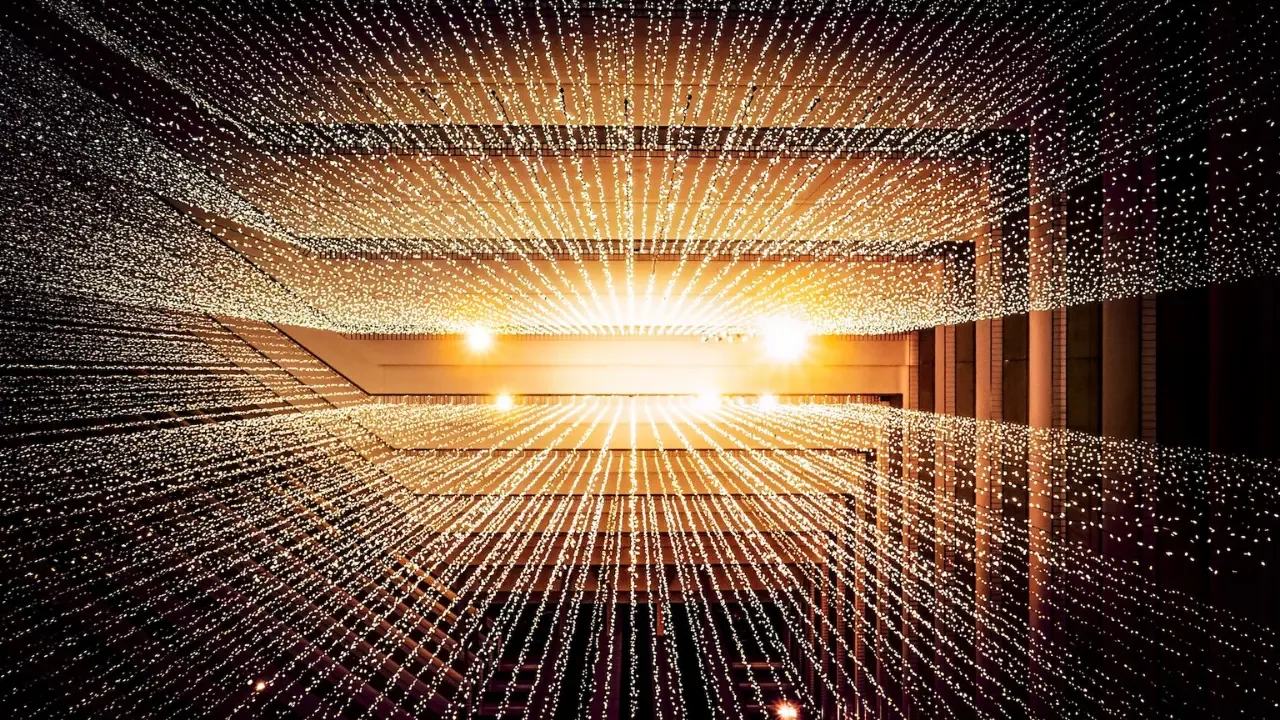
AngularJS app.run() Documentation: Understanding and Optimal Usage
š¤·āāļø Are you confused about when and how to use the app.run()
function in AngularJS? Don't worry, you're not alone! Many developers struggle to understand the best practices and optimal usage of this important function. In this guide, we will dive into the documentation around app.run()
, discuss common issues, provide easy solutions, and empower you to become an AngularJS pro! š
What is app.run()?
The app.run()
function in AngularJS is a powerful feature that allows you to perform initialization or setup tasks when your application starts. It runs after all the modules have been configured and the injector has been created.
To use app.run()
, you simply define a callback function that will be executed when your application starts up. This makes it an ideal place to perform tasks like setting up global event listeners, initializing third-party libraries, or even pre-fetching some data. šÆ
Where to use app.run()?
Now comes the question, "When should I use app.run()
in my application?" The answer is straightforward: use it after module definition, just like any other config function.
The correct order should be: app.module() -> app.config() -> app.run() -> app.controller()
.
It's important to note that app.run()
should be called only once in your application. Calling it multiple times will override the previous callback function. So, ensure that you have only one app.run()
function in your codebase. š«
Common Issues and Solutions
Issue: I am encountering errors when using app.run() with third-party libraries.
This problem often occurs when you try to access a library that has not been fully loaded or initialized yet. Remember that app.run()
runs immediately after the injector has been created, but the library might not be ready at that moment.
Solution: To solve this issue, make sure you properly inject and initialize third-party libraries in the app.run()
callback function. You can use AngularJS's dependency injection to ensure that the library is fully loaded before you try to use it. š¦
Here's an example:
app.run(['$timeout', function($timeout) {
$timeout(function() {
// Third-party library is fully loaded and ready to use
}, 1000);
}]);
In this example, we have used the $timeout
service to delay the execution of our callback function by one second, giving the third-party library enough time to load.
Issue: I need to retrieve some data before my application starts.
In certain scenarios, you might need to fetch some data from an API or server before your application can fully run. For example, you might want to load some user-specific configuration or authentication details. app.run()
can help you accomplish this task efficiently.
Solution: In your app.run()
callback function, you can make use of AngularJS's $http
service to fetch the required data asynchronously. Once the data is retrieved, you can store it in a service or factory that can be accessed by your controllers.
app.run(['$http', 'UserDataService', function($http, UserDataService) {
$http.get('/api/user-data')
.then(function(response) {
UserDataService.setData(response.data);
})
.catch(function(error) {
console.error('Error fetching user data:', error);
});
}]);
In this example, we make an API call to fetch user data and store it in the UserDataService
. This data can now be used by any controller or service within your application.
Empower Yourself with app.run()!
Now that you have a better understanding of app.run()
, it's time to level up your AngularJS development skills! Start incorporating app.run()
into your projects to perform initialization tasks and streamline your application's setup process.
Remember, the key is to call app.run()
after your module definition and place any necessary third-party library initialization or data fetching code inside the callback function.
If you come across any challenges or have questions while using app.run()
, feel free to leave a comment below. Let's grow and learn together! š
P.S. Don't forget to share this guide with your fellow AngularJS developers who might also benefit from understanding app.run()
better. Sharing is caring! š
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
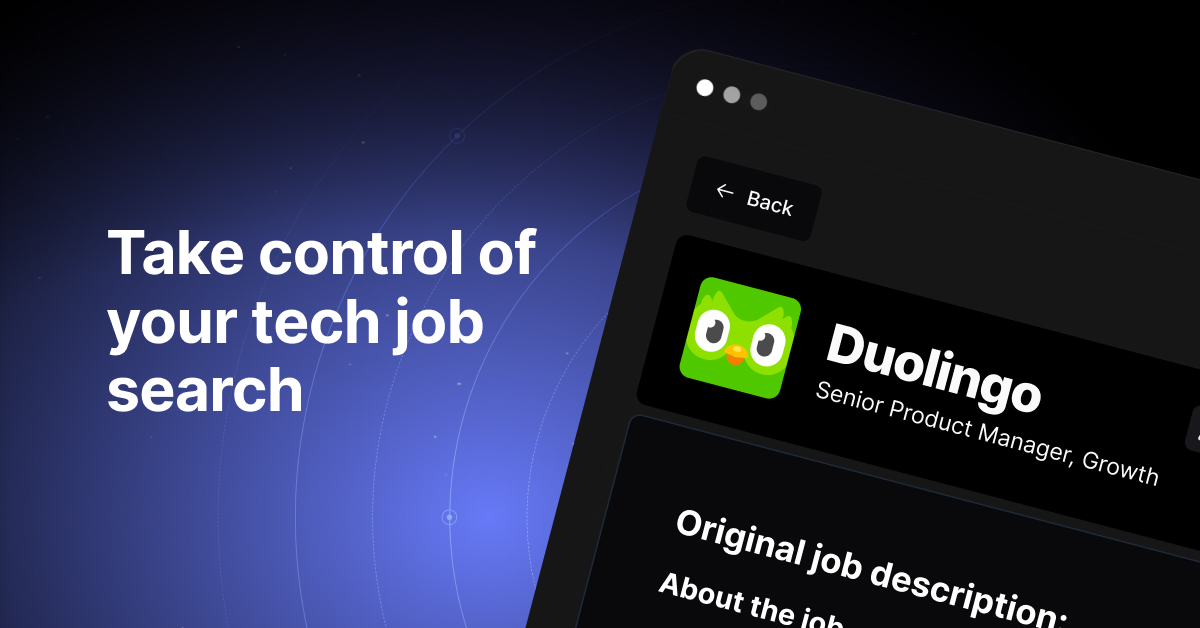