Angular pass callback function to child component as @Input similar to AngularJS way
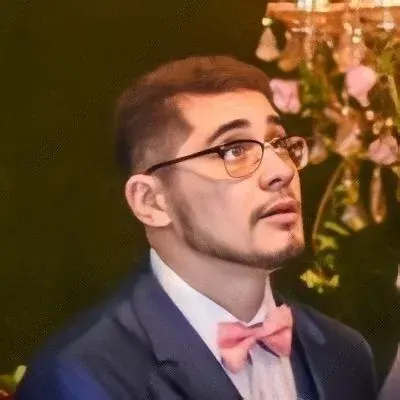
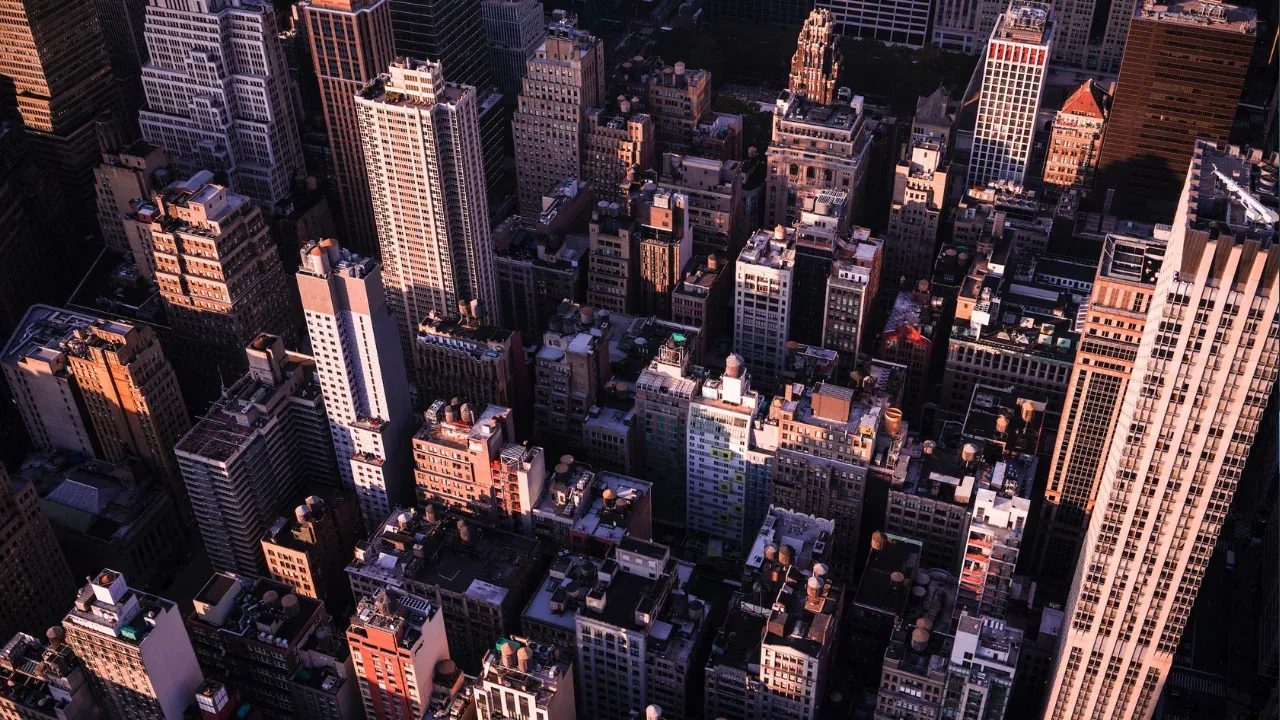
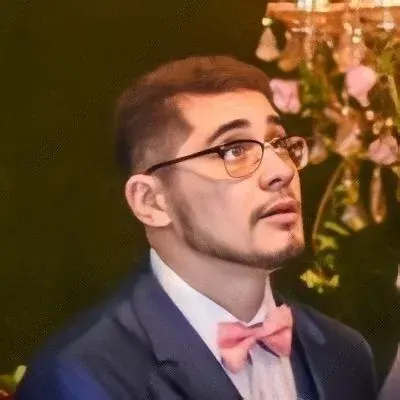
Angular: Passing a Callback Function to a Child Component as @Input
Are you an Angular developer trying to pass a callback function to a child component, similar to the AngularJS way? 🤔 In AngularJS, we had the &
parameter that allowed us to pass a callback to a directive. But what about Angular? Is it possible to pass a callback as an @Input
for an Angular component? And if not, what would be the closest alternative? Let's find out! 😎
The Challenge
Suppose we have a parent component that wants to pass a callback function to a child component. In AngularJS, we could easily achieve that with the &
parameter. But in Angular, things are a bit different. Let's consider the following code snippet as an example:
@Component({
selector: 'suggestion-menu',
template: `
<div (mousedown)="suggestionWasClicked(suggestion)">
</div>
`,
})
export class SuggestionMenuComponent {
@Input() callback: Function;
suggestionWasClicked(clickedEntry: SomeModel): void {
this.callback(clickedEntry);
}
}
And in the parent component, we want to pass the insertSuggestion
function as a callback to the child component:
<suggestion-menu [callback]="insertSuggestion"></suggestion-menu>
The Solution
Unfortunately, Angular does not provide a built-in way to pass a callback function as an @Input
to a child component. But don't worry, we have a workaround! 🚀
Instead of directly passing the callback function as an @Input
, we can use an intermediary property. Let's modify our code to implement this workaround:
@Component({
selector: 'parent-component',
template: `
<suggestion-menu [callback]="getCallback"></suggestion-menu>
`,
})
export class ParentComponent {
insertSuggestion(clickedEntry: SomeModel): void {
// Your logic here... 🧠
}
getCallback(): Function {
return this.insertSuggestion.bind(this);
}
}
In this example, we created a separate method getCallback
in the parent component, which returns the actual callback function insertSuggestion
bound to the parent component's context. By using the bind
method, we ensure that the callback function has the correct this
reference when executed.
Now, in the child component, we can receive the intermediary property as an @Input
and invoke it as a callback:
@Component({
selector: 'suggestion-menu',
template: `
<div (mousedown)="suggestionWasClicked(suggestion)">
</div>
`,
})
export class SuggestionMenuComponent {
@Input() callbackGetter: Function;
suggestionWasClicked(clickedEntry: SomeModel): void {
const callback = this.callbackGetter?.();
if (callback) {
callback(clickedEntry);
}
}
}
Notice that we're checking if the callbackGetter
property exists before invoking it as a function. This is important to avoid runtime errors if the parent component doesn't provide a callback getter.
The Next Steps
Congratulations! You can now pass a callback function to a child component in Angular using the workaround we described. But don't stop here! There's so much more you can do with Angular. Explore the official Angular documentation, experiment with different techniques, and level up your Angular skills! 💪
Did you find this article helpful? Do you have any other Angular-related questions or topics you'd like us to cover? Let us know in the comments below! We'd love to hear from you and keep providing valuable resources for your Angular journey. Happy coding! 😄👩💻👨💻