Angular JS break ForEach
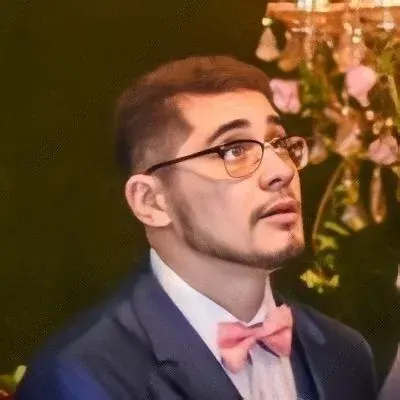
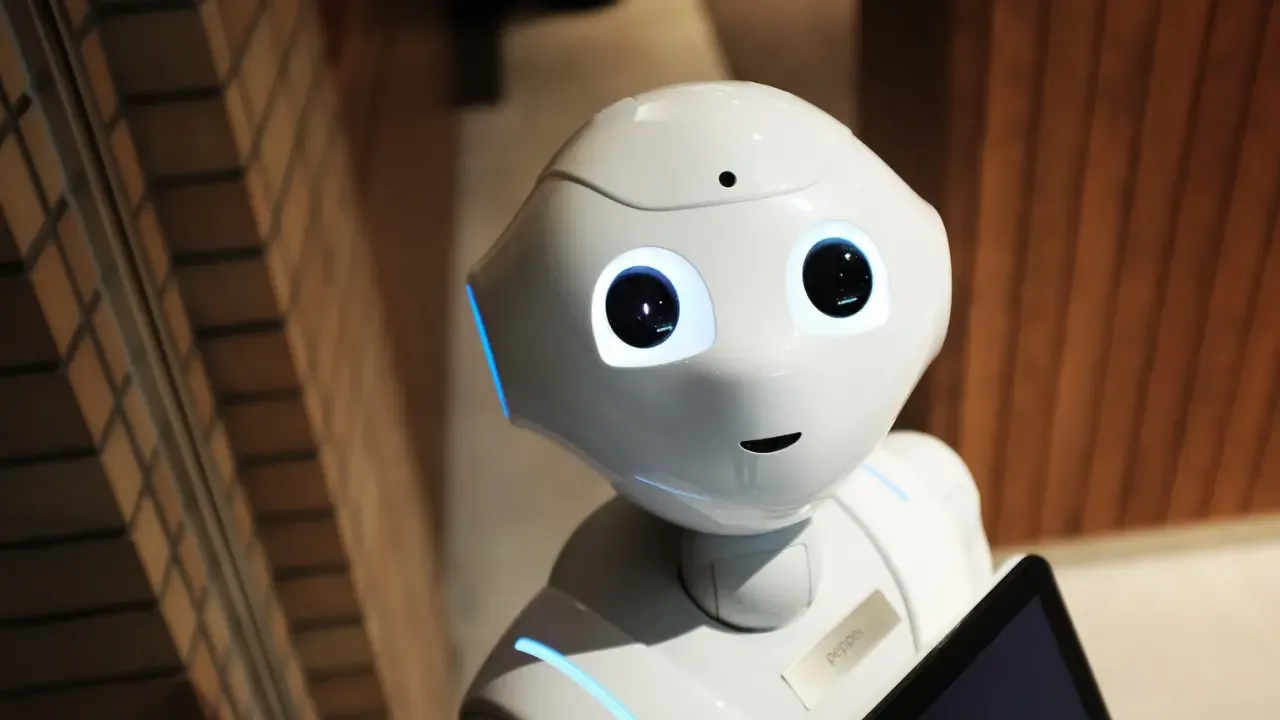
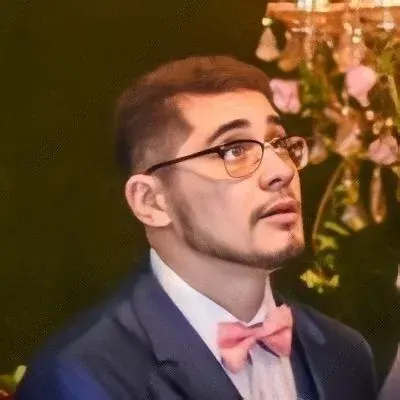
How to Break a ForEach Loop in AngularJS
So you're working with AngularJS and you have a forEach loop, but you want to break out of the loop if a certain condition is met. You've tried using the break
statement, but it doesn't seem to be working. Don't worry, we've got you covered! In this article, we'll show you how to break out of a forEach loop in AngularJS and provide you with easy solutions to achieve the desired result.
The Problem
Let's take a look at the code you provided:
angular.forEach([0, 1, 2], function (count) {
if (count == 1) {
break;
}
});
The above code snippet tries to break out of the forEach loop when the count
variable equals 1. However, the code throws an error because the break
statement is not valid inside a forEach loop. This is because forEach is a higher-order function that takes a callback function as an argument, and breaking out of the forEach loop from within the callback function is not supported.
The Solution
To achieve the desired result of breaking out of a forEach loop in AngularJS, you can use a boolean flag variable that is checked within the callback function. Here's an example:
var shouldBreak = false;
angular.forEach([0, 1, 2], function (count) {
if (count === 1 && !shouldBreak) {
shouldBreak = true;
return;
}
// Rest of your code here
});
In the above code, we introduce a boolean flag variable shouldBreak
that is initially set to false
. Inside the forEach loop, we check if shouldBreak
is still false
and if the count
variable equals 1. If both conditions are met, we set shouldBreak
to true
and use the return
statement to exit out of the current iteration of the loop.
By using this approach, you can effectively break out of the forEach loop when the desired condition is met.
A Better Alternative: Using a for Loop
Another alternative to breaking out of a forEach loop in AngularJS is to use a traditional for loop which allows the break statement. Here's an example:
var arr = [0, 1, 2];
for (var i = 0; i < arr.length; i++) {
var count = arr[i];
if (count === 1) {
break;
}
// Rest of your code here
}
In the code above, we use a for loop instead of a forEach loop. This allows us to use the break
statement to exit out of the loop when the desired condition is met.
Conclusion
Breaking out of a forEach loop in AngularJS can be a bit tricky since the break
statement is not supported directly within the callback function. However, by using a boolean flag variable or opting for a for loop instead, you can achieve the desired result.
Remember, when working with AngularJS or any other programming language, it's important to understand the specific behavior and limitations of the language constructs you are using. With this knowledge, you'll be better equipped to overcome any challenges that come your way.
We hope you found this guide helpful in solving your problem! If you have any further questions or suggestions, feel free to leave a comment below. Happy coding! 🚀