What is the difference between Subject and BehaviorSubject?
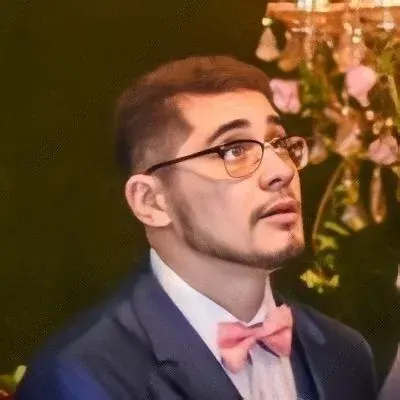
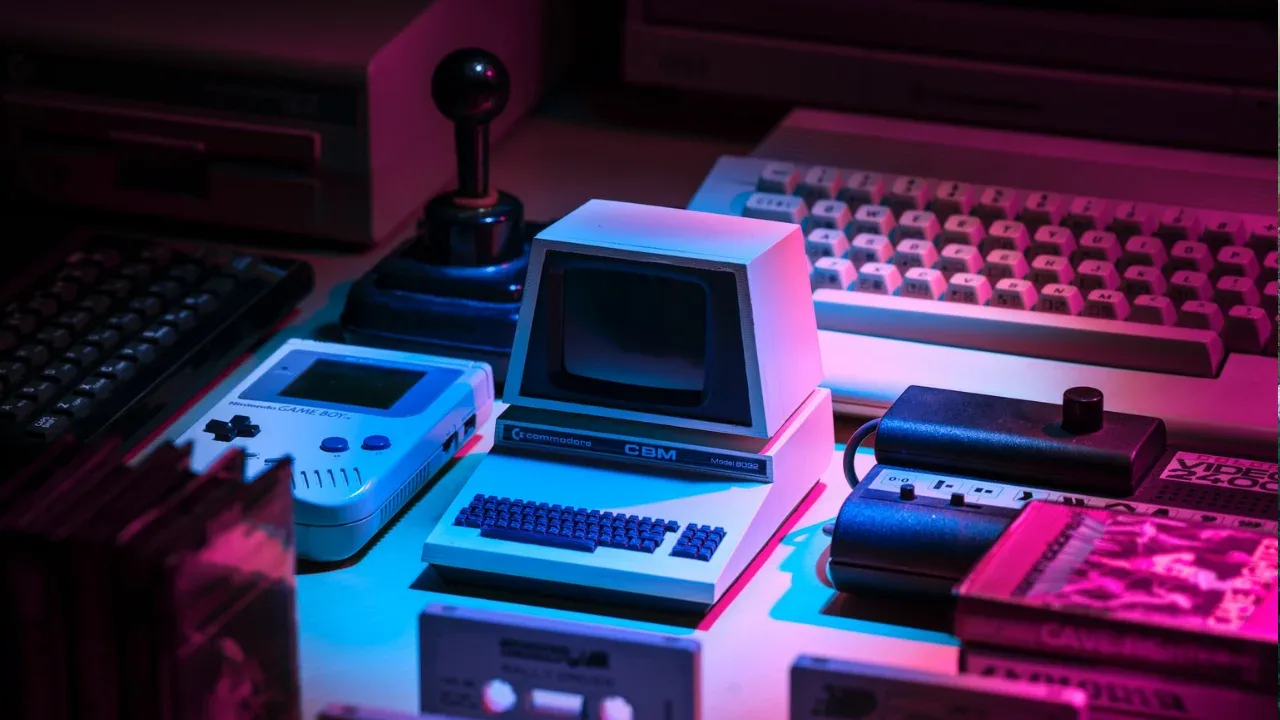
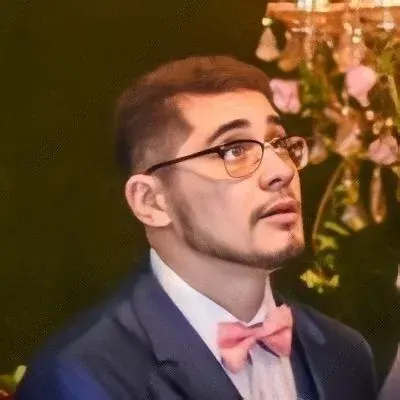
Subject vs BehaviorSubject: Explained in Plain English! ππ
Are you a JavaScript developer who often finds yourself confused between Subject
and BehaviorSubject
? π€ Don't worry, my friend! You're not alone in this quest for clarity. π
In this blog post, we'll explore the key differences between these two powerful RxJS subjects, and how they can be used to level up your reactive programming game. Buckle up and let's dive right in! πͺπ
Subject: The Mysterious Communicator π’π€«
A Subject
, my dear readers, is the core building block of RxJS. It acts as a multicast observable, meaning it can emit values to multiple subscribers. Think of it as a mysterious communicator that can shout out important news to anyone willing to listen. π£οΈπ°
Here's an example to bring this concept to life:
const mySubject = new Subject();
mySubject.subscribe((value) => {
console.log('Subscriber 1:', value);
});
mySubject.subscribe((value) => {
console.log('Subscriber 2:', value);
});
mySubject.next('Hello, world!');
π€―Mind-blowing, isn't it? By calling mySubject.next('Hello, world!')
, we trigger a notification that reaches both subscribers, resulting in the following output:
Subscriber 1: Hello, world!
Subscriber 2: Hello, world!
BehaviorSubject: The Thoughtful Storyteller ππ€
Now, let's meet our thoughtful storyteller, BehaviorSubject
. Just like Subject
, it can also multicast values to multiple subscribers. However, it has an essential superpower β the ability to emit a default value upon subscription. ππ‘
To better explain this, let's modify our previous example:
const myBehaviorSubject = new BehaviorSubject('Initial value');
myBehaviorSubject.subscribe((value) => {
console.log('Subscriber 1:', value);
});
myBehaviorSubject.subscribe((value) => {
console.log('Subscriber 2:', value);
});
myBehaviorSubject.next('Hello, world!');
Guess what? This time, when we execute this code snippet, the output will surprise you! π
Subscriber 1: Hello, world!
Subscriber 2: Hello, world!
Did you notice that both subscribers received the 'Hello, world!'
message, just like with Subject
? But wait, there's more! π
If you add a new subscriber after the initial value is emitted, it will still receive the latest value from the BehaviorSubject
, along with any future updates:
myBehaviorSubject.subscribe((value) => {
console.log('Subscriber 3:', value);
});
π Breaking News:
Subscriber 3: Hello, world!
Our new subscriber receives the latest value, 'Hello, world!'
, upon subscription. Amazing, right?
Recap and Recommendations β¨π
To summarize, here are the key differences between Subject
and BehaviorSubject
:
Subject
doesn't emit a default value upon subscription, whileBehaviorSubject
does.BehaviorSubject
holds the latest value, and new subscribers receive the current value instantly.Both
Subject
andBehaviorSubject
can multicast values to multiple subscribers.
Now that we've cleared up the confusion, it's time for you to choose which subject best fits your use case. Take a moment to consider your requirements before diving into the world of reactive programming. π€π
Take the Next Step! πΆββοΈ
If you're still hungry for more knowledge, don't worry! We have tons of valuable resources on our website that will help you master reactive programming concepts using RxJS. ππͺ
Check out our in-depth tutorials, practical examples, and engaging community discussions. ππ©βπ» Don't forget to subscribe to our newsletter to stay up-to-date with the latest trends in the JavaScript world. ππ
Remember, becoming a reactive programming ninja takes time and practice. So, keep calm and keep coding! π»βοΈ
Let's hear from you! π£
Have you ever struggled with understanding Subject
and BehaviorSubject
? How did you overcome it? Share your thoughts, experiences, and any additional questions you have in the comments section below. Let's grow and learn together! πβ€οΈ