What is the difference between Promises and Observables?
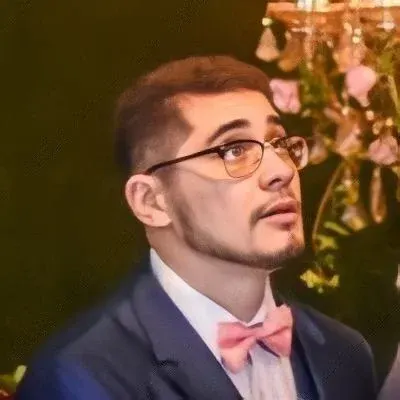
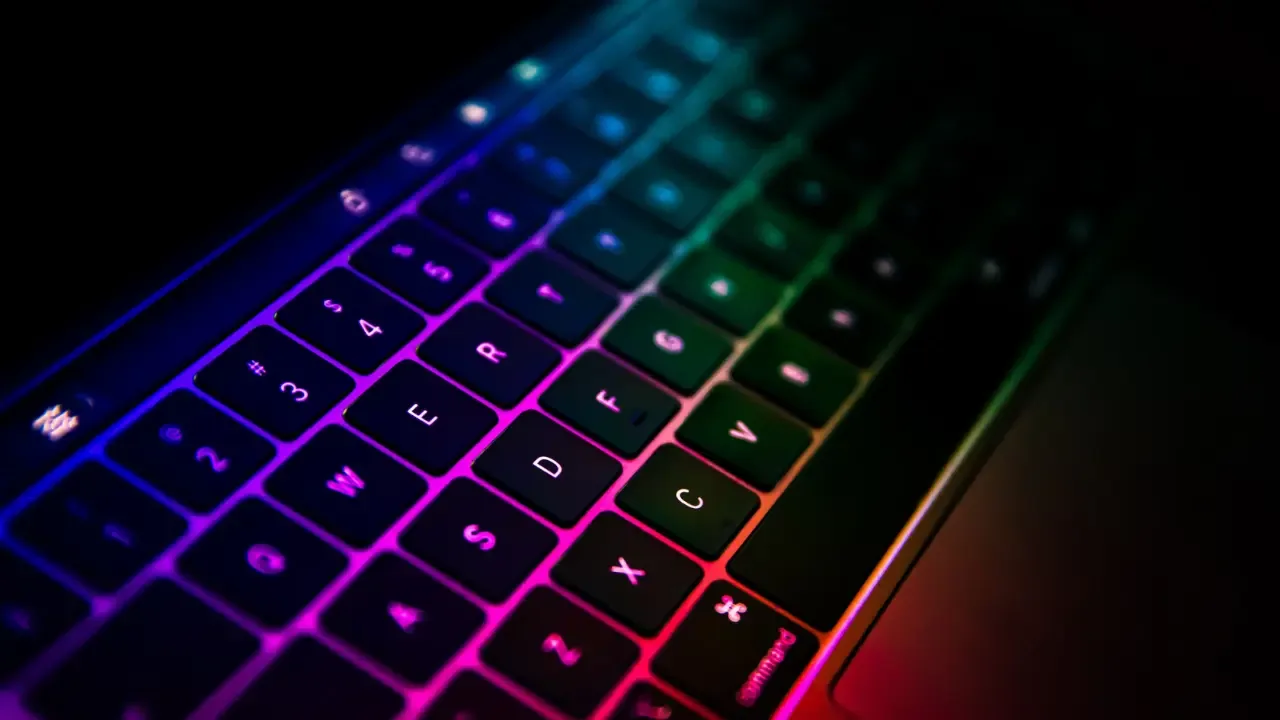
The Battle of Promises and Observables: Unraveling the Angular Mystery! 👊💥🔍🕵️♀️
So, you're knee-deep in Angular development, and you've come face to face with two powerful entities: Promises and Observables. You stare at them, and they stare back, mocking you with their similarities and differences. Fear not, for I am here to guide you through this epic battle and help you understand the differences between Promises and Observables. 🤝💡
What is a Promise? 🤝🔐
In the realm of JavaScript, a Promise is an object that represents an asynchronous operation. It has two possible outcomes - it can either be fulfilled with a value or rejected with a reason. Think of a Promise as a contract, an agreement that something will be done in the future. You can use Promises when you have an operation that will be completed once, and you're only interested in the final outcome. 🤞✨
Here's an example to solidify your understanding:
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
const data = "Hello, Promises!";
resolve(data);
}, 2000);
});
}
fetchData().then((data) => {
console.log(data);
});
In this example, we create a Promise that resolves with the string "Hello, Promises!"
after a 2-second delay. We then handle the resolved value using the .then()
method.
What is an Observable? 🌈🕒
Unlike Promises, Observables are powerful entities from the ReactiveX library that represent a stream of values over time. They can emit multiple values asynchronously, allowing you to react to changes over time. Observables provide a way to handle data streams and perform various operations on them using operators. They give you superpowers to handle events, HTTP requests, and even user input! 🦸♀️📺
Let's dive into an example to see Observables in action:
import { Observable, interval } from 'rxjs';
const observable = new Observable((observer) => {
const timer = interval(1000);
timer.subscribe((value) => {
observer.next(`Hello, Observables! ${value}`);
});
});
observable.subscribe((data) => {
console.log(data);
});
In this code snippet, we create an Observable using the rxjs
library. It emits a value every second using the interval
operator. We then subscribe to the Observable and log the emitted values to the console.
When to use Promises and Observables? 🤔🌟
Promises are great when you have a single asynchronous operation with a one-time outcome. If you just need a value once, use a Promise. On the other hand, Observables are your go-to tool when you want to handle events over time, work with continuous data streams, perform HTTP requests, or deal with user interactions. Observables give you more flexibility and control for handling complex scenarios. 🔄🚀
Conclusion and Battle Verdict 🏁⚖️
In the battle between Promises and Observables, who emerges victorious? The answer lies in your specific use case. Promises are simpler and easier to grasp for one-time operations, while Observables shine when dealing with continuous streams and complex scenarios. Both have their unique strengths, and mastering both will level up your Angular game. 🎮🔥
Now that you understand the battle between Promises and Observables, it's time to wield your newfound knowledge and choose wisely when building your Angular applications. So, which one will you pick for your next masterpiece? Let us know in the comments below! 💬👇
Remember, stay curious and keep evolving in your coding journey. Angular awaits your greatness! 💪🌟
Until next time, happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
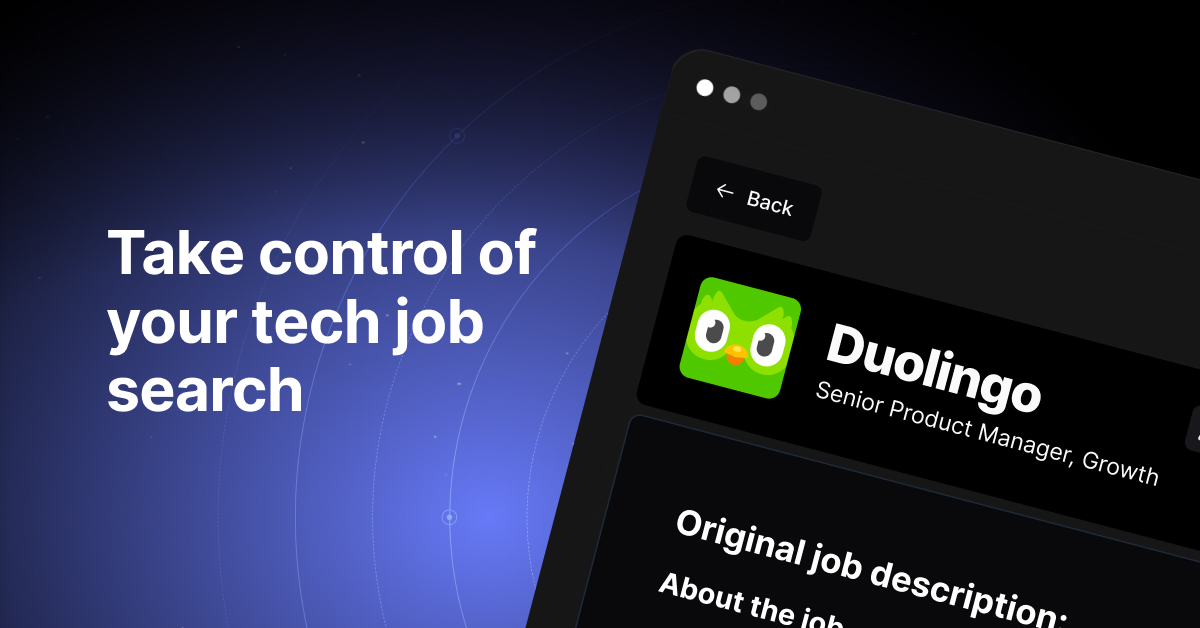