What is the correct way to share the result of an Angular Http network call in RxJs 5?
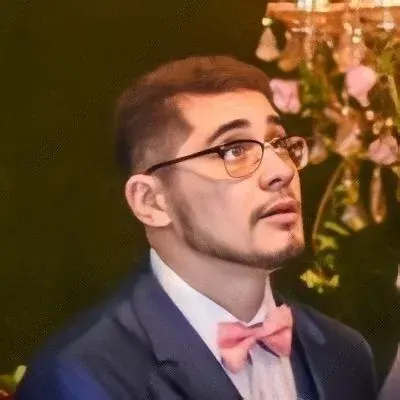
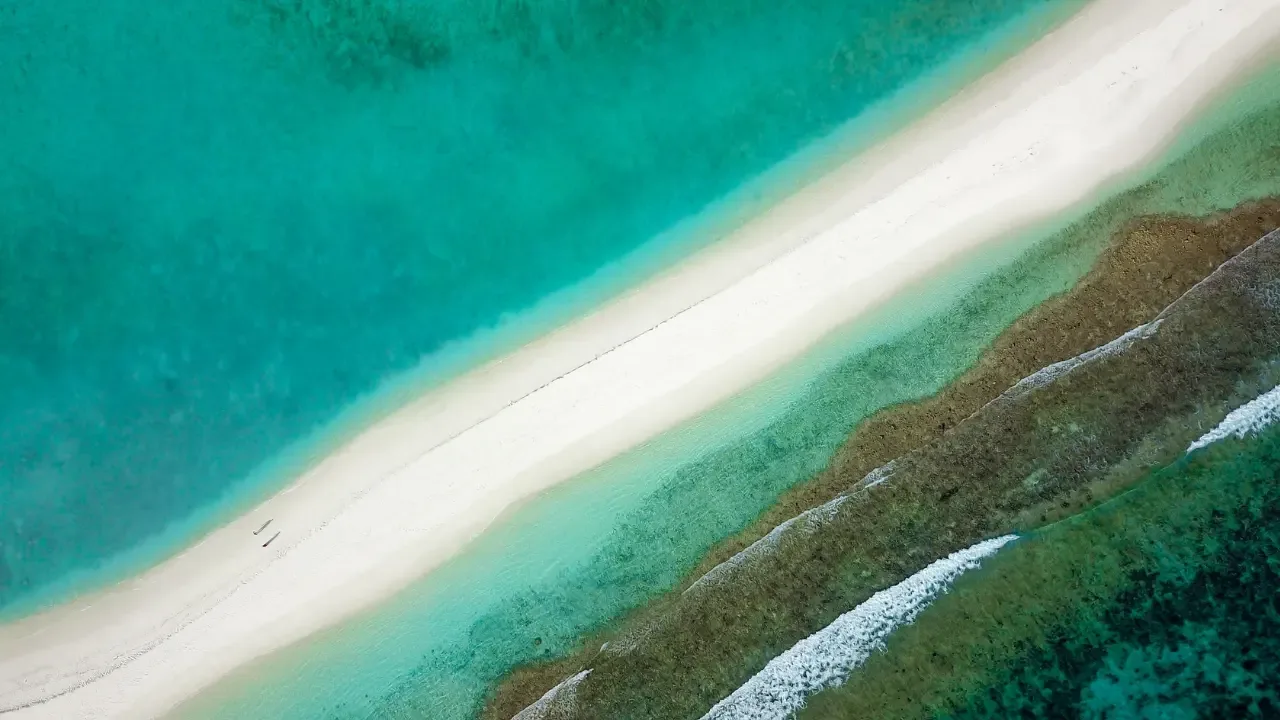
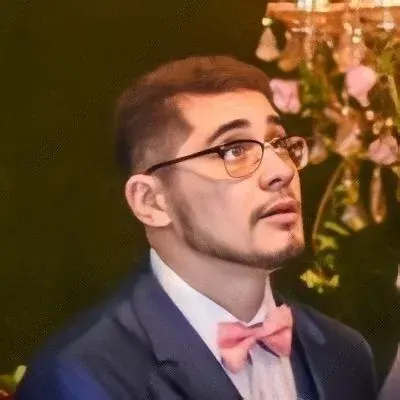
š Title: The Correct Way to Share the Result of an Angular Http Network Call in RxJs 5
š Hey there, fellow developers! Today, we're going to tackle a common challenge in Angular: sharing the result of an Http network call in RxJs 5. š It might seem confusing at first, but fear not! We've got your back.
š” The Challenge: Multiple Subscribers, Multiple Network Requests
So, imagine this situation: you have a method in Angular that performs an Http network call and returns an Http Observable. Simple, right? But what if you have multiple subscribers to this Observable? š¤
Let's say we have the following code:
getCustomer() {
return this.http.get('/someUrl').map(res => res.json());
}
let network$ = getCustomer();
let subscriber1 = network$.subscribe(...);
let subscriber2 = network$.subscribe(...);
Looks fine at a glance, but here's the catch: multiple subscribers might cause multiple network requests, which is not what we want. š āāļø
ā¹ļø The Common Scenario
This situation might sound uncommon, but in reality, it's quite common. For instance, if you subscribe to the Observable to display an error message and use the async pipe in the template, you already have two subscribers. š®
š The Solution: .share() to the Rescue!
Thankfully, RxJs 5 provides us with a solution - the .share()
operator. By simply modifying our code like this:
getCustomer() {
return this.http.get('/someUrl').map(res => res.json()).share();
}
We ensure that the network request is only made once, regardless of how many subscribers we have. Problem solved! š
š” The Idiomatic Way
Now, you might be wondering - is this the idiomatic way to handle this in RxJs 5? The answer is YES! Using .share()
is the recommended approach to share the result of an Http network call among multiple subscribers in RxJs 5. It's concise, efficient, and easy to implement. š
š Ready to Level Up Your Angular Game?
Now that you've mastered the art of sharing Http network call results in RxJs 5, take your Angular skills to the next level! š Dive deeper into the world of observables and operators, uncover new tricks and techniques, and become an Angular rockstar!
š¢ Your Call-to-Action
Have any thoughts or experiences to share about sharing Http network call results in Angular using RxJs 5? Leave us a comment below and let's start a conversation! š£ļø Don't forget to share this post with your fellow developers - together, we can conquer Angular challenges! š