Use component from another module
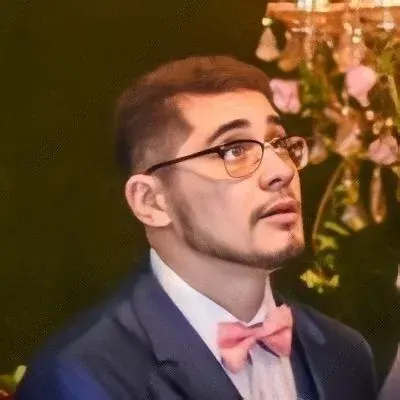
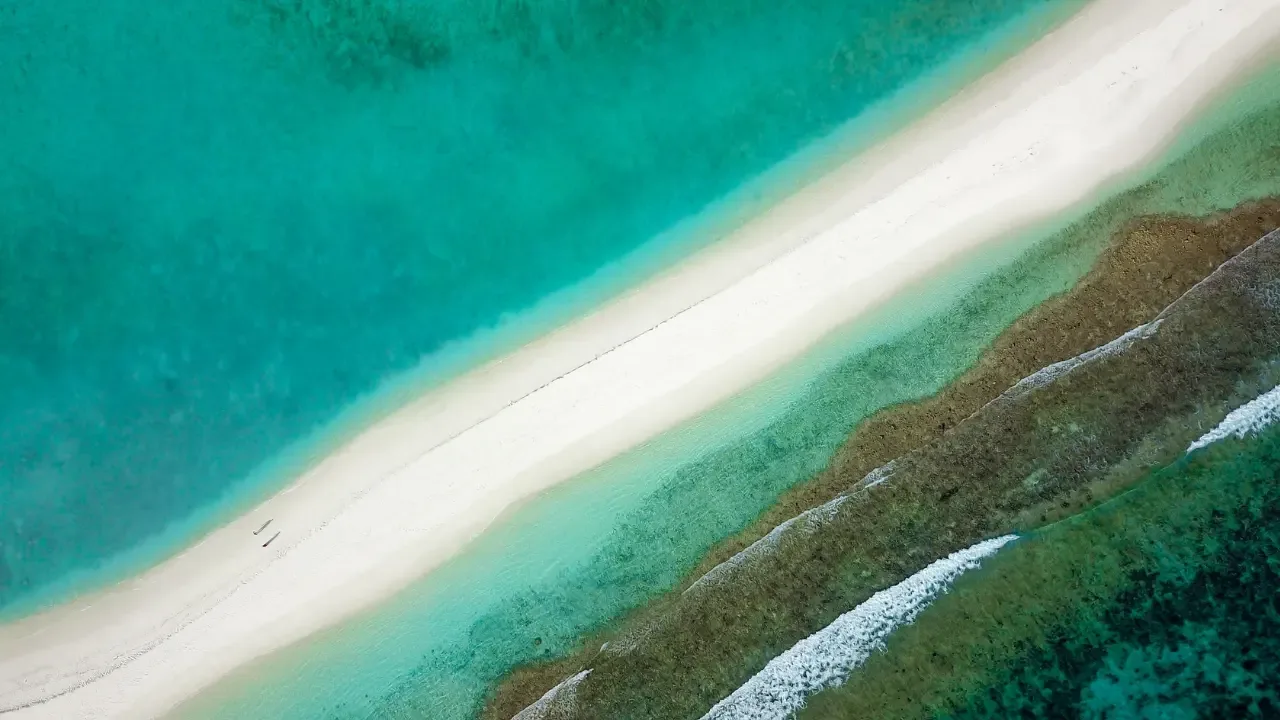
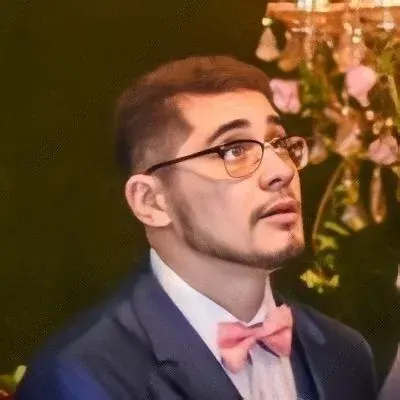
The Ultimate Guide to Using Components from Another Module in Angular 🚀💡
So you've created an Angular 2 app and decided to separate your components into modules. But now you've run into a little hiccup - you want to use a component from one module in another module. No worries, we've got you covered! In this guide, we'll walk you through the steps to successfully use a component from another module in your Angular app. Let's dive in! 💪
Understanding the Problem 🤔
In this scenario, we have an AppModule
and a TaskModule
, and we want to use the TaskCardComponent
from the TaskModule
in the BoardComponent
of the AppModule
.
Solution #1: Importing the Component 💻
The first solution involves importing the component directly into the module where you want to use it.
Open the
BoardComponent
file in yourAppModule
.Add an import statement for the
TaskCardComponent
from theTaskModule
.
import { TaskCardComponent } from '../task/task-card/task-card.component';
Make sure to use the correct file path in the import statement.
Now you can use the
TaskCardComponent
in theBoardComponent
as if it was declared in the same module.
@Component({
selector: 'app-board',
templateUrl: './board.component.html',
styleUrls: ['./board.component.css']
})
export class BoardComponent {
// You can now use the TaskCardComponent here
}
You're done! The
TaskCardComponent
is now available for use in theBoardComponent
of theAppModule
.
Solution #2: Exporting and Importing the Module 📦
If you find yourself using multiple components from the same module in different modules, it might be more efficient to export the entire module and import it wherever you need it. Let's explore this solution!
Open the
TaskModule
file.Add the
exports
property to theNgModule
decorator and include theTaskCardComponent
in the array.
@NgModule({
declarations: [TaskCardComponent],
imports: [MdCardModule],
exports: [TaskCardComponent], // Add this line
providers: []
})
export class TaskModule {}
Save the file and open the
AppModule
file.Remove the import statement for the
TaskCardComponent
if you added it in Solution #1.Import the entire
TaskModule
instead.
import { TaskModule } from './task/task.module'; // Import the module
@NgModule({
declarations: [
AppComponent,
BoardComponent,
LoginComponent,
PageNotFoundComponent
],
imports: [
BrowserModule,
FormsModule,
HttpModule,
MdButtonModule,
MdInputModule,
MdToolbarModule,
routing,
TaskModule // Import the module here
],
providers: [UserService],
bootstrap: [AppComponent]
})
export class AppModule {}
You're all set! The
TaskCardComponent
and any other components from theTaskModule
are now available for use in theAppModule
.
Conclusion 🎉
Using components from another module in Angular doesn't have to be complicated. By following our step-by-step solutions, you can easily incorporate components from different modules into your app.
Remember, Solution #1 is great for using a specific component from a module, while Solution #2 is perfect when you need to use multiple components from the same module in different modules.
Now it's your turn! Try implementing these solutions in your own Angular app and see the magic happen. If you have any questions or need further assistance, feel free to leave a comment below. Happy coding! 😄💻