Stop mouse event propagation
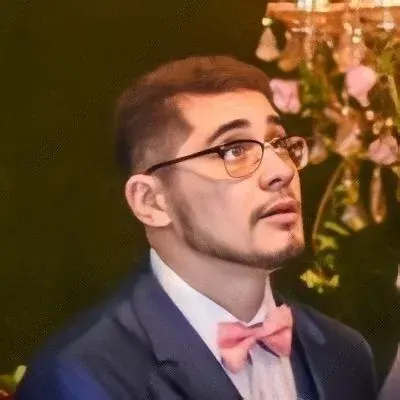
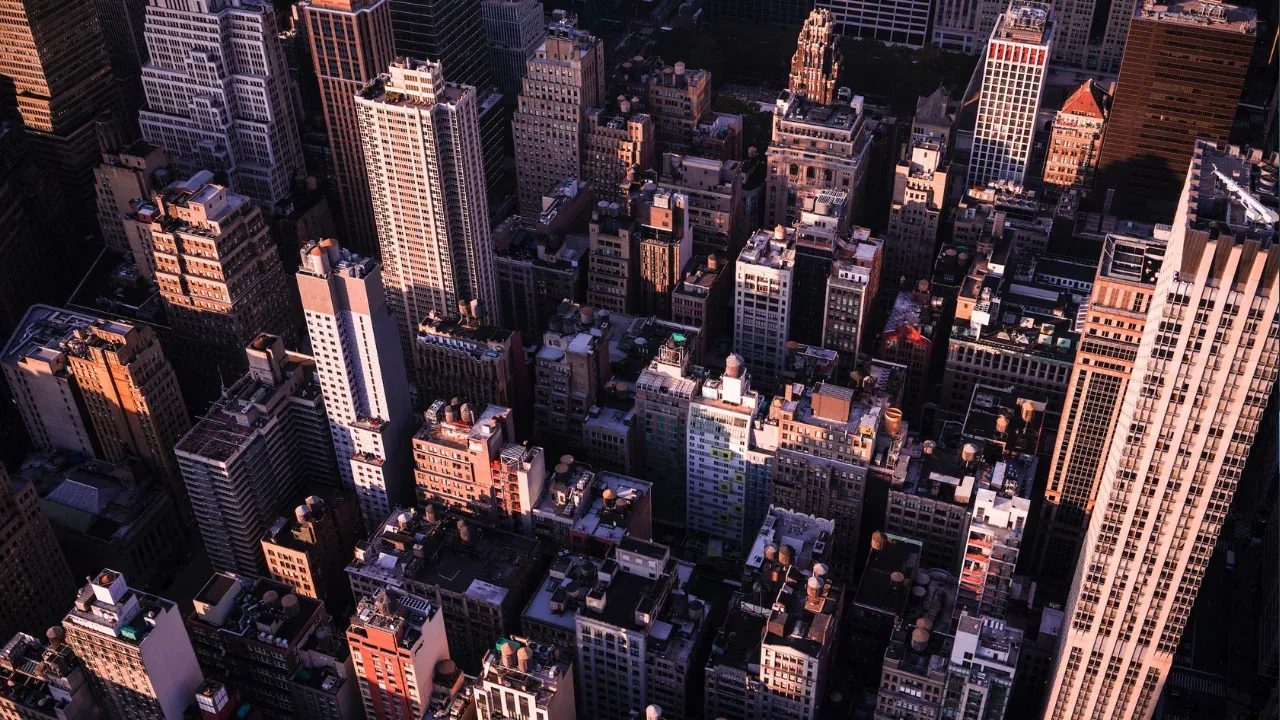
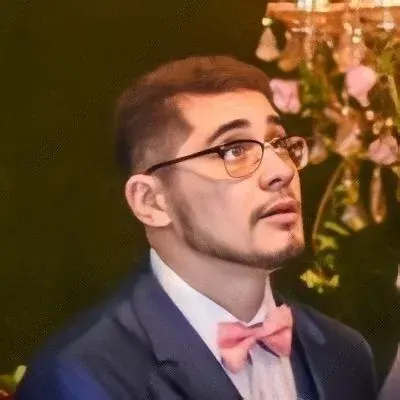
Stop Mouse Event Propagation in Angular: A Quick and Easy Guide
š« Welcome to our tech blog! Today, we are going to explore a common issue faced by Angular developers: stopping mouse event propagation. š±ļø This post aims to provide you with simple solutions and demystify any confusion you may have regarding this topic. So, let's dive right in and find out the easiest way to stop mouse event propagation in Angular! š
The Common Issue
Many Angular developers often wonder which approach they should take to stop mouse event propagation. š¤ Should they pass a special $event
object and manually call stopPropagation()
? Or is there a more straightforward alternative available?
The Solution
Fortunately, Angular has a built-in solution for stopping mouse event propagation. š Instead of manually calling stopPropagation()
, you can utilize a handy Angular directive called stopPropagation
. This directive allows you to stop event bubbling with just a few lines of code. Let's see how it's done! š
Steps to Stop Mouse Event Propagation:
Import the
stopPropagation
directive from@angular/platform-browser
.
import { Directive } from '@angular/core';
import { EventManager } from '@angular/platform-browser';
@Directive({
selector: '[stopPropagation]'
})
export class StopPropagationDirective {
constructor(private eventManager: EventManager) { }
ngOnInit() {
this.eventManager.addGlobalEventListener('document', 'click', this.stopPropagation);
}
stopPropagation(event: Event) {
event.stopPropagation();
}
}
Attach the
stopPropagation
directive to the HTML element for which you want to stop mouse event propagation.
<div (click)="myClickHandler()" stopPropagation>
<!-- Your content here -->
</div>
That's it! š By adding the stopPropagation
directive to the desired HTML element, you can prevent mouse event propagation from occurring beyond that particular element. You no longer need to manually pass the $event
object or worry about calling stopPropagation()
. Angular does the hard work for you! š
Illustrated Example
Let's take a look at a practical example to solidify our understanding. Consider the following scenario: you have a button inside a dropdown menu, and you want to stop the dropdown from closing when the button is clicked. In this case, we can utilize the stopPropagation
directive. šŖ
<div (click)="closeDropdown()">
<button (click)="buttonClickHandler($event)" stopPropagation>Do not close</button>
</div>
In the above code snippet, the stopPropagation
directive is applied to the <button>
element. Now, when the button is clicked, the click event will not bubble up to the parent element, thereby preventing the dropdown menu from closing.
Call-to-Action: Engage and Share!
From now on, you have a simple and elegant solution to stop mouse event propagation in Angular! ⨠Don't forget to share this helpful guide with your fellow Angular developers. Let's spread the knowledge and make coding easier for everyone! š
If you have any other questions or need further assistance, feel free to leave a comment below. Our tech community is always here to help! š