Rendering Angular components in Handsontable Cells
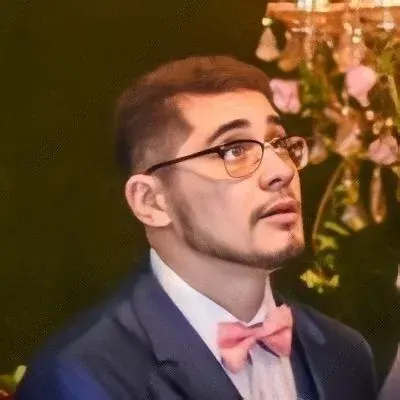
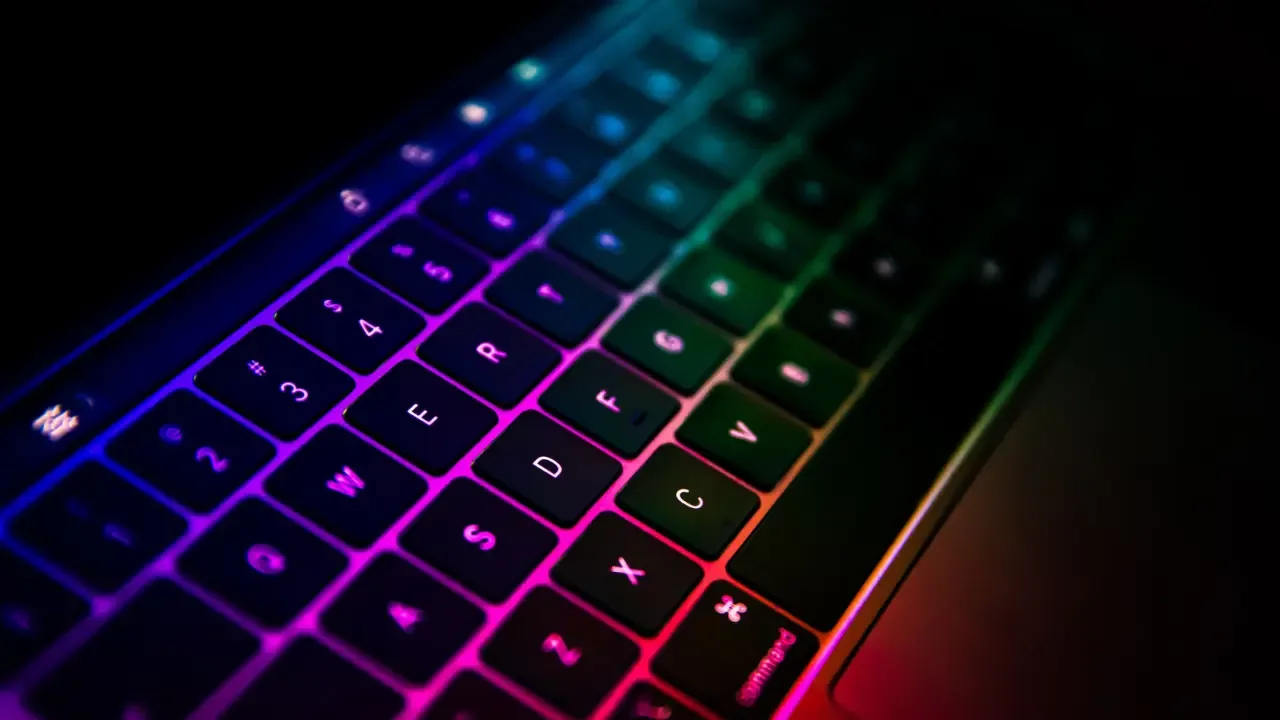
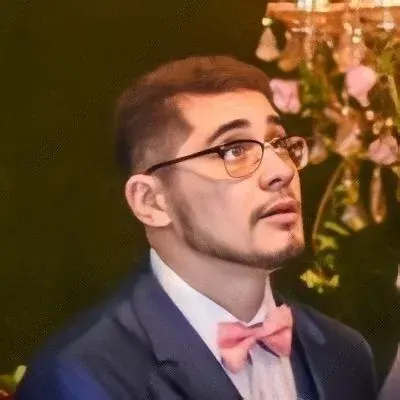
Rendering Angular Components in Handsontable Cells 🎯💻🔧
Are you struggling with displaying Angular Components in a table using Handsontable? You're not alone! Many developers face issues when it comes to rendering Angular Components like an Autocomplete Dropdown Search inside Handsontable cells. But fret not! In this blog post, I'll address common issues and provide easy solutions to help you overcome this challenge. So, let's dive in! 🏊♀️💡
The Problem 😫
In a recent project, I encountered a scenario where I needed to display Angular Components (like an Autocomplete Dropdown Search) in a table. I decided to use Handsontable due to its features, such as multi-selecting cells with Ctrl
+click
. However, I faced some difficulties when it came to rendering the Angular Components inside the cells.
The Approach 🚀
The approach I took involved using the Handsontable renderer and dynamically adding the components to the DOM. Here's an example of the code structure:
// matrix.component.ts
this.hot = new Handsontable(this.handsontable.nativeElement, {
data: this.tableData,
colWidths: [80, 300],
colHeaders: ['Id', 'Custom Component'],
columns: [
{
data: 'id',
},
{
data: 'id',
renderer: (instance: any, td: any, row: any, col: any, prop: any, value: any, cellProperties: any) => {
if (cellProperties.hasOwnProperty('ref')) {
(cellProperties.ref as ComponentRef<CellContainerComponent>).instance.value = row;
} else {
cellProperties.ref = this.loadComponentAtDom(
CellContainerComponent,
td,
((component: any) => {
component.template = this.button4Matrix;
component.value = row;
})
);
}
return td;
},
readOnly: true,
},
],
});
private loadComponentAtDom<T>(component: Type<T>, dom: Element, onInit?: (component: T) => void): ComponentRef<T> {
let componentRef;
try {
const componentFactory = this.componentFactoryResolver.resolveComponentFactory(component);
componentRef = componentFactory.create(this.injector, [], dom);
onInit ? onInit(componentRef.instance) : console.log('no init');
this.appRef.attachView(componentRef.hostView);
} catch (e) {
console.error('Unable to load component', component, 'at', dom);
throw e;
}
return componentRef;
}
Common Issues 😩
Throughout my experimentations, I encountered some common issues that you might also come across:
Angular ngOnDestroy not being called: Angular wasn't triggering the ngOnDestroy method of the
CellContainerComponent
.Error during component destruction: When attempting to destroy the components rendered earlier, either an error ('cannot read property 'nativeNode' of null') occurred or the components were displayed incorrectly.
Mixed up values during scrolling: While recycling already-rendered components, the values got mixed up during scrolling.
Easy Solutions ✅
Let's explore the solutions I tried for each of the above issues:
Do nothing: I initially tried leaving everything to Angular without any additional handling.
Problem: Angular never called the ngOnDestroy of the
CellContainer
.
Saving componentRefs: I attempted to save the
ComponentRef
instances in an array and destroy the components after a certain rendering threshold.Problem: Component destruction resulted in either errors or incorrect display of components.
Checking element presence during rendering: I checked if an element was already present during rendering, and if so, recycled the existing component by assigning a new value.
Problem: The values got completely mixed up during scrolling.
The Solution ⚒💡
If you're eager to see my solution to these issues and an implemented solution for #3, you can find it on GitHub.
Let's Connect! 🤝🌐
Do you have any other ideas or suggestions on how to handle this problem in a cleaner way? I'd love to hear from you! Share your thoughts and experiences in the comments below. Together, we can make the application faster, smoother, and more user-friendly. 😊💬
And if you want to explore more about Handsontable and its functionality, check out their tutorial on Cell Function.
Happy coding! ✨👩💻🚀