Property "value" does not exist on type "EventTarget"
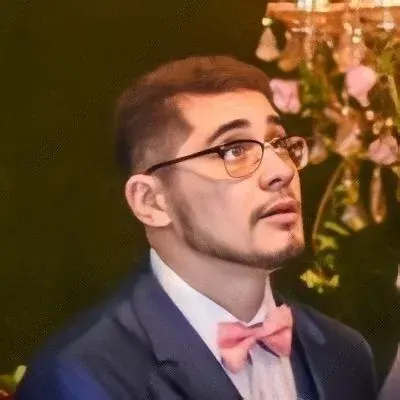
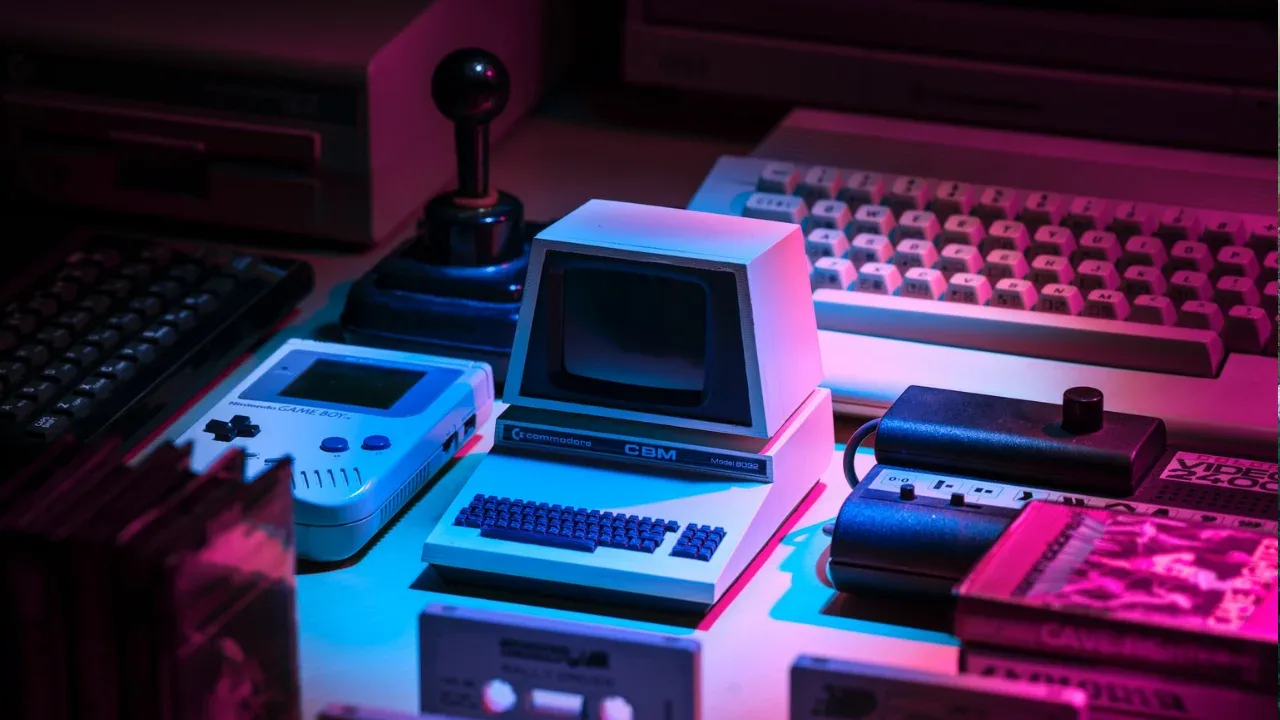
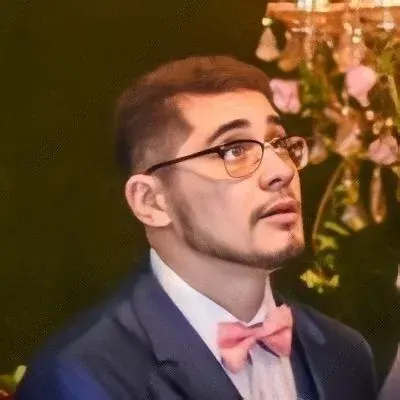
Property 'value' does not exist on type 'EventTarget' - Simple Solutions! 😎
Have you ever encountered the error message "Property 'value' does not exist on type 'EventTarget'" while working with TypeScript and Angular 2? Well, worry no more! In this article, we'll walk you through some common issues surrounding this error and provide easy solutions to help you resolve it quickly.
Understanding the Error
The error is caused by TypeScript's type checking mechanism, which is designed to ensure type safety in your code. In this case, TypeScript is complaining because it cannot find the 'value' property on the 'EventTarget' type. The 'EventTarget' type is a native DOM interface that doesn't have a predefined 'value' property.
The Code Snippet
Let's take a look at the code snippet that triggered the error:
import { Component, EventEmitter, Output } from '@angular/core';
@Component({
selector: 'text-editor',
template: `
<textarea (keyup)="emitWordCount($event)"></textarea>
`
})
export class TextEditorComponent {
@Output() countUpdate = new EventEmitter<number>();
emitWordCount(e: Event) {
this.countUpdate.emit(
(e.target.value.match(/\S+/g) || []).length);
}
}
As you can see, the error stems from the emitWordCount
method, where we are accessing the 'value' property of the e.target
. However, TypeScript doesn't recognize the 'value' property on the 'EventTarget' type, which leads to the error.
🛠️ Easy Solutions
Luckily, fixing this error is straightforward. We have a couple of options to consider:
Solution 1: Use Type Casting
One way to resolve this issue is by explicitly casting the event target to the appropriate type, in this case, HTMLInputElement. We can accomplish this by using the 'as' keyword like this:
emitWordCount(e: Event) {
const target = e.target as HTMLInputElement;
this.countUpdate.emit(
(target.value.match(/\S+/g) || []).length);
}
By casting e.target
to HTMLInputElement
, TypeScript now recognizes the 'value' property and the error will disappear.
Solution 2: Use Event Type
Another approach is to make use of the appropriate event type instead of the generic 'Event'. In this scenario, we can use the 'KeyboardEvent' type, which provides a more specific interface for keyboard events. Here's how to modify the code:
emitWordCount(e: KeyboardEvent) {
this.countUpdate.emit(
(e.target.value.match(/\S+/g) || []).length);
}
By using 'KeyboardEvent' as the event type, TypeScript understands that 'e.target
' is an input element and recognizes the 'value' property.
These solutions ensure that TypeScript recognizes the 'value' property and the error is resolved.
📣 Call to Action
Now that you've learned how to fix the "Property 'value' does not exist on type 'EventTarget'" error, it's time to put your newfound knowledge into action! Remember, practice makes perfect, so try implementing these solutions in your own code.
If you found this article helpful, be sure to share it with your friends and colleagues who might be facing the same issue. And don't hesitate to leave a comment if you have any questions or other TypeScript-related topics you'd like us to cover.
Happy coding! 💻✨