Property "of" does not exist on type "typeof Observable
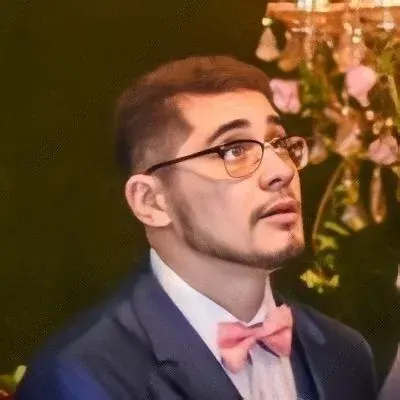
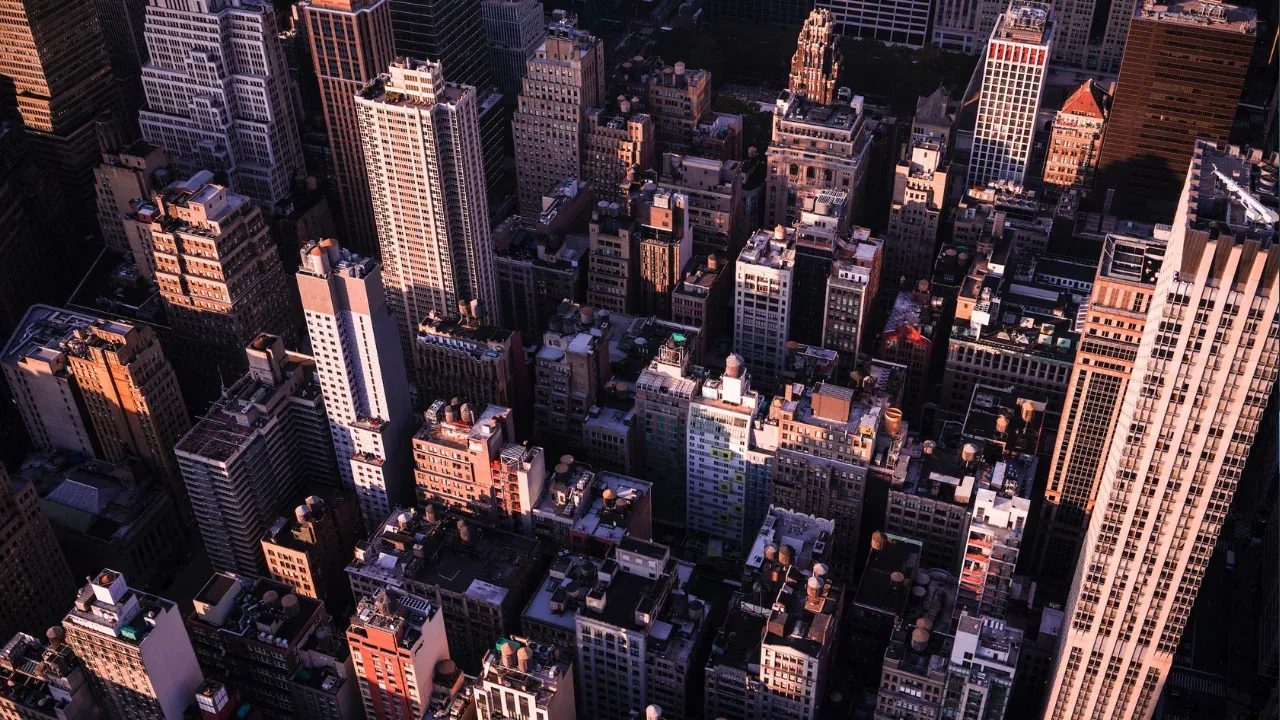
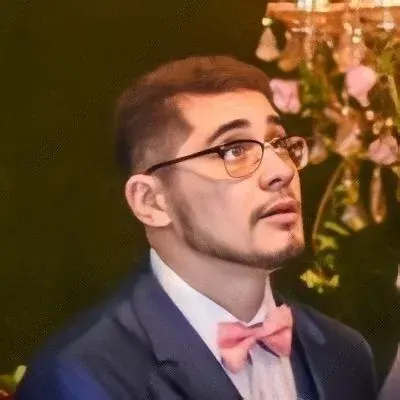
π What's the Problem?
So, you were going about your coding business, using Observable.of('token')
to work with your authentication token. But something went wrong. No matter how hard you've imported it, TypeScript keeps throwing an error in your face: "Property 'of' does not exist on type 'typeof Observable'." Argh, frustrating! π‘
But fear not! I'm here to help you unravel this conundrum and get you back on track with your token handling in no time. Let's dive in and find out what's causing this error and how to fix it gracefully. π
π Understanding the Error
The error message you're seeing indicates that TypeScript cannot find the of
property on the Observable
type. TypeScript is a statically typed superset of JavaScript, which means it needs to know what properties and methods are available on a certain type. In this case, it seems TypeScript is unaware of the of
method on the Observable
type.
π‘ The Solution
To fix this TypeScript error, there are a couple of approaches you can take. Let's explore them both.
1. Import the correct operator
If you're using a more recent version of RxJS, the of
operator has been moved to a separate module. So instead of importing it from 'rxjs/Observable'
, you should import it from 'rxjs'
directly. Here's how you can do it:
import { of } from 'rxjs';
of('token');
By importing directly from 'rxjs'
, you'll have access to the of
operator without any issues, and TypeScript will be a happy camper. ποΈ
2. Create a custom Observable using the create
method
If you prefer to stick with the Observable
type import from 'rxjs/Observable'
, you can create your own custom observable using the create
method. Here's how:
import { Observable } from 'rxjs/Observable';
const customObservable = Observable.create(observer => {
observer.next('token');
observer.complete();
});
customObservable.subscribe(token => {
// Do something with the token
});
By creating a custom observable, you have full control over its behavior and can emit any value or sequence you desire. This workaround bypasses the need for the of
operator altogether.
π£ Call-to-Action: Engage and Share!
Now that you've conquered this pesky TypeScript error, take a moment to celebrate your victory! π But don't stop thereβshare your newfound knowledge with your coding buddies. Together, we can make the coding world a better place, one error at a time.
If you found this blog post helpful, feel free to share it with others who are having the same issue. Let's spread the word and help more developers slay those TypeScript dragons! πͺ
And of course, if you have any questions or want to share your own experiences, drop a comment below. I'd love to hear from you and geek out on TypeScript together! π©βπ»π¨βπ»