NullInjectorError: No provider for AngularFirestore
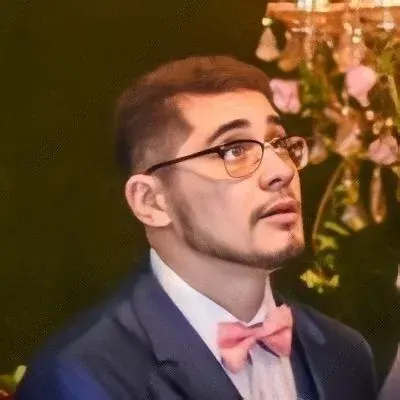
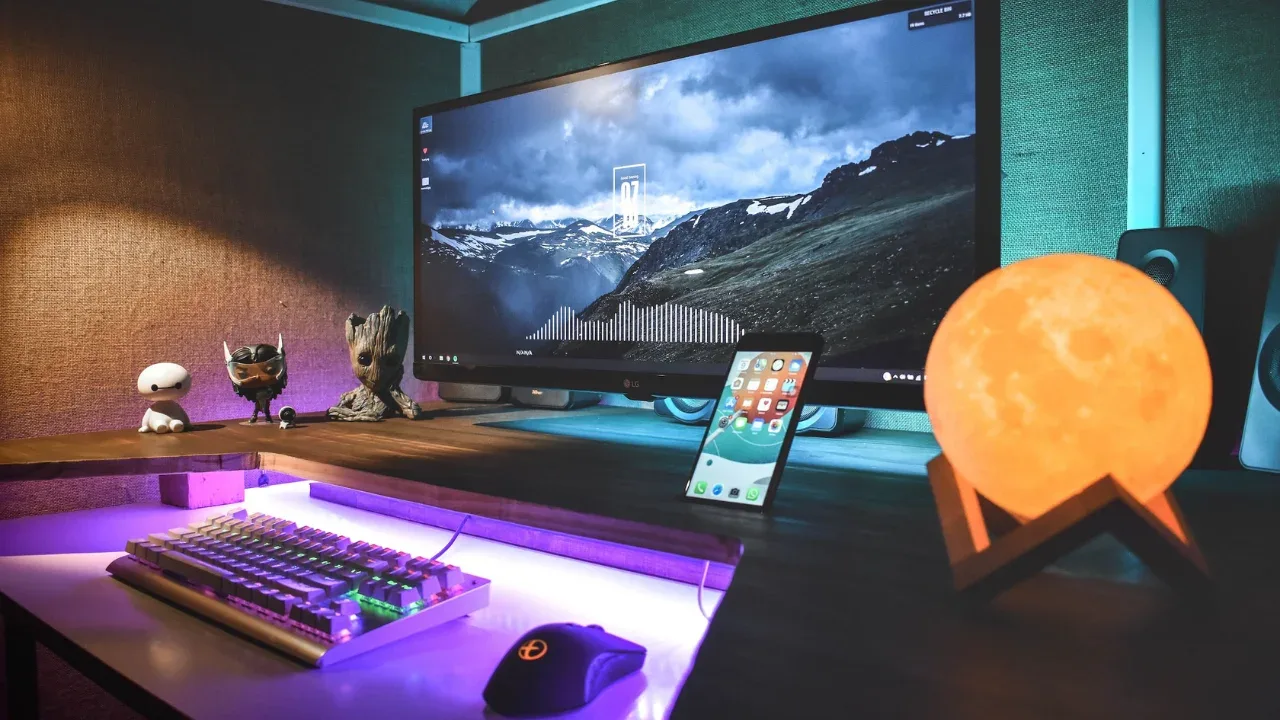
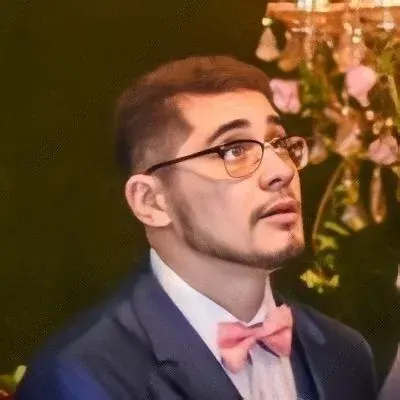
📝 Fixing the NullInjectorError: No provider for AngularFirestore
So you're learning Angular and came across this error: NullInjectorError: No provider for AngularFirestore. Don't worry, I've got you covered! I'll walk you through some common issues that might cause this error and provide an easy solution to fix it.
🚀 The problem
The NullInjectorError occurs when Angular's dependency injection system cannot find a provider for a requested dependency. In this case, it means that AngularFirestore is not being properly provided to your application.
✅ Possible solutions
Importing AngularFireModule.forRoot()
In your
app.module.ts
file, make sure you have importedAngularFireModule
and calledinitializeApp()
with your Firebase config object. However, it seems like you have already done this correctly. Double-check that your Firebase config variables inenvironment.ts
are correct.import { AngularFireModule } from 'angularfire2'; import { environment } from '../environments/environment'; //... @NgModule({ imports: [ BrowserModule, AngularFireModule.initializeApp(environment.firebase) ], //... }) export class AppModule {}
Providing AngularFirestore in your component
The next step is to provide AngularFirestore in your component that uses it. In your
app.component.ts
file, make sure you add AngularFirestore to the constructor.import { Component } from '@angular/core'; import { AngularFirestore } from 'angularfire2/firestore'; //... export class AppComponent { title = 'app'; constructor(db: AngularFirestore) {} }
By adding
db: AngularFirestore
to the constructor, you are telling Angular to inject an instance of AngularFirestore when creating an instance of AppComponent.Checking the version compatibility
Ensure that the version of
angularfire2
you are using is compatible with the version of Angular you are using. In some cases, a mismatch in versions can cause problems. Check the compatibility table in the AngularFirestore official documentation to ensure you have the correct version combination.Importing AngularFirestoreModule
Starting from AngularFirestore version 5, you need to import
AngularFirestoreModule
explicitly in yourapp.module.ts
file. Make sure to add it to theimports
array.import { AngularFirestoreModule } from 'angularfire2/firestore'; //... @NgModule({ imports: [ BrowserModule, AngularFireModule.initializeApp(environment.firebase), AngularFirestoreModule ], //... }) export class AppModule {}
Checking for typos or typos in imports
Lastly, make sure you don't have any typos or errors in your import statements. Check if the file paths are correct and if the module names are accurate.
🎉 That's it!
Now you should be able to successfully build and run your Angular application without the NullInjectorError: No provider for AngularFirestore error popping up. If you encounter any further issues, double-check the Documentation for AngularFire2 and make sure to update the package versions as required.
🏗️ Let's engage!
If you found this blog post helpful, please consider sharing it with your friends or on your social media accounts. Let me know in the comments if you have any other Angular-related questions or topics you'd like me to cover. Happy coding! 👩💻🔥