Is it necessary to unsubscribe from observables created by Http methods?
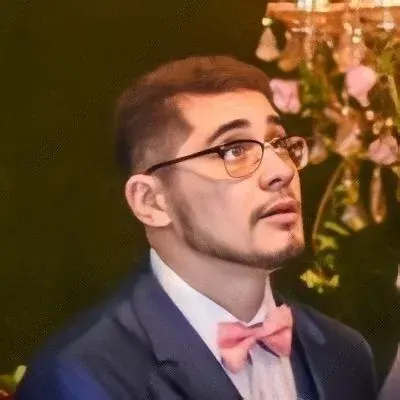
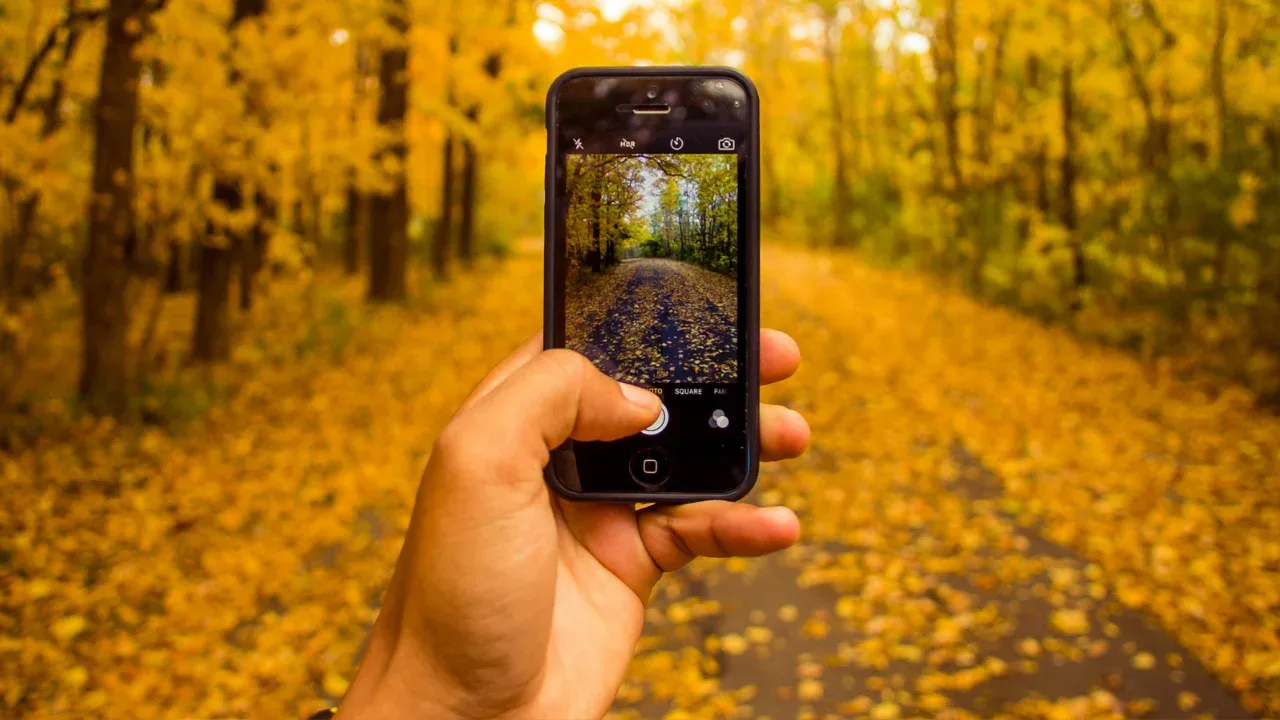
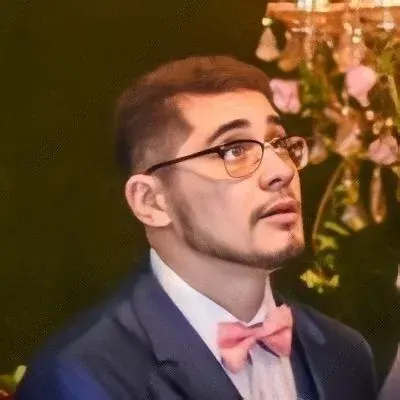
The Importance of Unsubscribing from Http Observables in Angular 2 📵
Have you ever encountered memory leaks in your Angular 2 application? 😰 It's a common problem that many developers face when they forget to unsubscribe from observables created by Http methods. But don't you worry, because in this blog post, we'll discuss why it's necessary to unsubscribe from these observables and provide you with easy solutions to prevent memory leaks in your Angular 2 app. Let's dive right in! 💦
Understanding the Problem 🧐
When you make Http calls in Angular 2 using the Http
module, an observable is returned. With observables, you can subscribe to receive the response data asynchronously. However, if you forget to unsubscribe from these observables when they're no longer needed, they will continue to listen for changes, which can lead to memory leaks and decreased performance. 😨
In the code snippet provided, you can see that the .subscribe
method is being used, but it's important to note that it's also unsubscribing immediately after dispatching the received film. This is a good practice, as it ensures that the observable is unsubscribed and any resources associated with it are released.
Easy Solutions to Prevent Memory Leaks 👌
Unsubscribe using take(1): One simple solution is to use the
take(1)
operator from the RxJS library. This operator subscribes to the observable and automatically unsubscribes after receiving the first emitted value. This ensures that you're only listening for the response data once, preventing any potential memory leaks. 🔄
Here's an example of how you can modify your code snippet to incorporate the take(1)
operator:
fetchFilm(index) {
this._http.get(`http://example.com`)
.map(result => result.json())
.map(json => {
this.dispatch(this.receiveFilm(json));
})
.take(1)
.subscribe();
}
Unsubscribe using ngOnDestroy hook: Another solution is to implement the
OnDestroy
lifecycle hook in your component and manually unsubscribe from the observable when the component is destroyed. This ensures that the observable is always unsubscribed, even if the first emitted value has not been received. 💥
Here's an example of how you can implement the OnDestroy
hook and unsubscribe from the observable:
import { OnDestroy } from '@angular/core';
import { Subscription } from 'rxjs';
export class YourComponent implements OnDestroy {
private subscription: Subscription;
constructor(private _http: Http) {}
fetchFilm(index) {
this.subscription = this._http.get(`http://example.com`)
.map(result => result.json())
.map(json => {
this.dispatch(this.receiveFilm(json));
})
.subscribe();
}
ngOnDestroy() {
this.subscription.unsubscribe();
}
}
By implementing the OnDestroy
hook and unsubscribing from the observable in the ngOnDestroy
method, you can ensure that the observable is always properly unsubscribed, preventing any memory leaks.
Take Action and Prevent Memory Leaks Today! 🚀
Now that you understand the importance of unsubscribing from observables created by Http methods in Angular 2, it's time to take action and prevent memory leaks in your application. Whether you choose to use the take(1)
operator or implement the OnDestroy
hook, make sure to apply these solutions consistently throughout your codebase.
Remember, preventing memory leaks not only improves the performance of your application but also contributes to better resource management. So, don't delay! Start implementing these solutions today and say goodbye to those pesky memory leaks! 💪
If you found this blog post helpful, don't forget to share it with your fellow Angular 2 developers and let them know about the importance of unsubscribing from Http observables. And if you have any questions or additional tips, feel free to leave a comment below. Happy coding! 💻🌟