How to truncate text in Angular2?
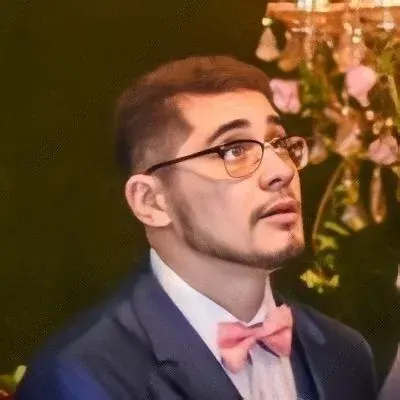
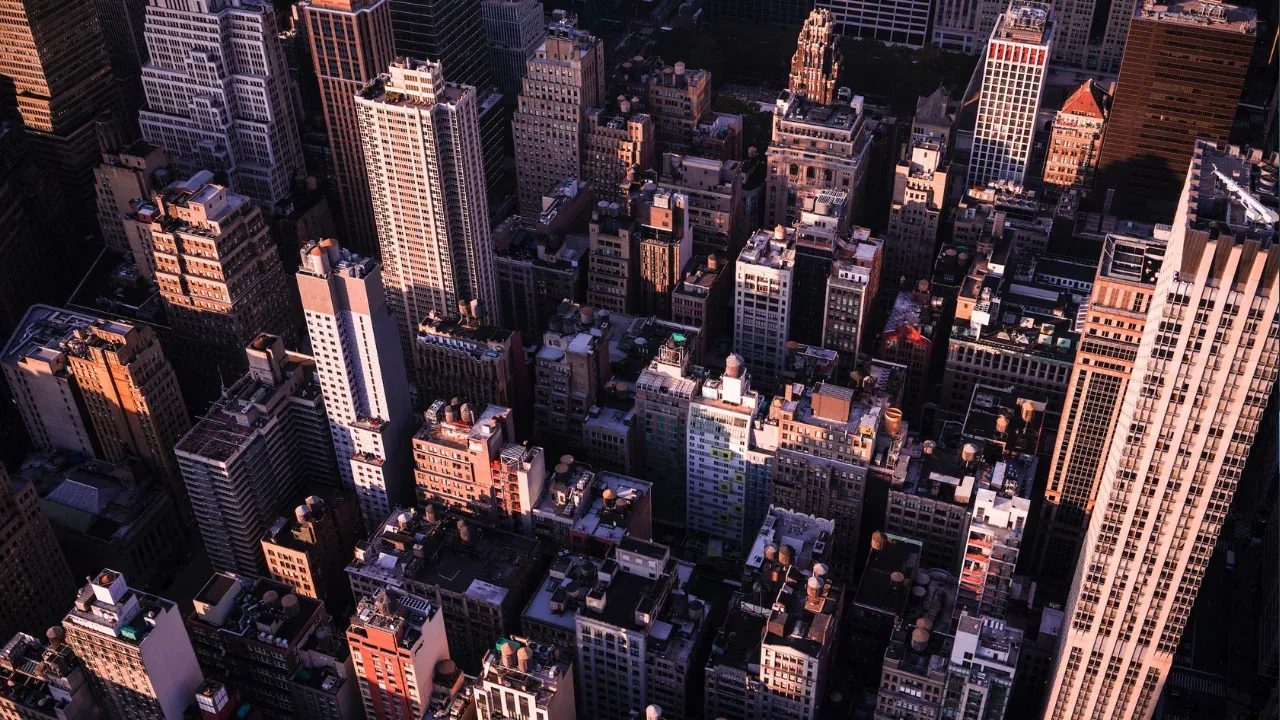
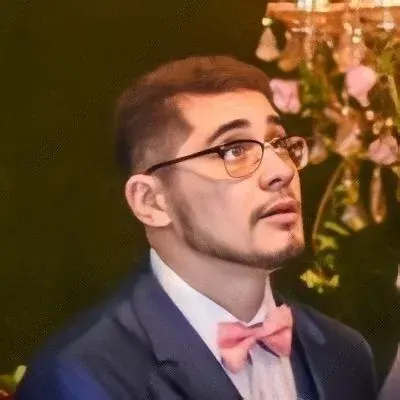
How to Truncate Text in Angular2? 💥
Introduction ✨
So, you're working with Angular2 and you need to truncate text strings? You're in luck! We have the perfect solution to help you limit the length of your text in just a few simple steps. Whether you need to truncate a title, a paragraph, or any other text element, we've got you covered. Let's dive right in!
The Problem 🤔
It seems that you want to limit the length of a string to a specific number of characters. For example, you want to show only the first 20 characters of a title using Angular2. This is a common requirement in web applications, and luckily, Angular2 provides a few solutions to achieve this.
The Solution 💡
Option 1: Using a Pipe
Angular2 offers a handy pipe called slice
that allows you to extract a subset of a string. Here's how you can use it to truncate your title:
{{ data.title | slice:0:20 }}
This code will display only the first 20 characters of the data.title
string. Easy as pie, right? 🍰
Option 2: Using a Custom Function
If you prefer a more programmatic approach, you can create a custom function in your component that truncates the text for you. Here's an example of how you can achieve this:
truncateText(text: string, length: number): string {
if (text.length <= length) {
return text;
} else {
return text.substr(0, length) + '...';
}
}
You can then call this function in your template:
{{ truncateText(data.title, 20) }}
This will give you the truncated version of the title, with an ellipsis at the end if it exceeds 20 characters.
Conclusion 🎉
Now that you know how to truncate text in Angular2, you can easily limit the length of your strings without breaking a sweat. Whether you choose to use the slice
pipe or create a custom function, the choice is yours!
Go ahead and give it a try in your Angular2 project. Happy coding! 😄
Have any issues or other cool tricks? Share with us! 📣
If you face any issues or have any other cool tricks related to truncating text in Angular2, don't hesitate to leave a comment below. We're all about sharing knowledge and helping each other out!
So, what are you waiting for? Let us know your thoughts, ask questions, or share your experiences. We're here to help you out! 💪
Stay tuned for more exciting Angular2 tips and tricks on our blog. Until then, happy coding! 👩💻👨💻